MATLAB Examples for Beginners
This blog will give you all basic and advance MATLAB examples to learn MATLAB. This post covers MATLAB examples such as basic data types, mathematical operations, data visualization techniques, and real-world applications in engineering, mathematics, and scientific research.
What Is MATLAB?
MATLAB, short for Matrix Laboratory, is a high-level programming language and interactive environment primarily used for numerical computation, data analysis, algorithm development, and visualization. It was developed by MathWorks and has since become a fundamental tool in various fields including engineering, mathematics, and scientific research. The primary strength of MATLAB lies in its ability to manipulate matrices, making it an invaluable resource for solving complex mathematical problems and performing statistical analyses.
One of the key applications of MATLAB is in the field of engineering, where it is utilized for simulating systems, modeling real-time data, and executing tasks related to control systems and signal processing. For instance, engineers often employ MATLAB examples to design and simulate electrical circuits, analyze fluid dynamics, and optimize engineering solutions. Furthermore, MATLAB’s built-in functions and toolboxes facilitate the exploration of various technical problems, reducing development time while increasing accuracy and efficiency.
In addition to engineering, MATLAB finds applications in the realm of mathematics. It provides a comprehensive platform for performing linear algebra tasks, calculus computations, and statistical analyses. Researchers and students alike can leverage MATLAB examples to better understand mathematical concepts through visual representations of data, enhancing their learning experience. In scientific research, MATLAB is widely used for data visualization, modeling biological systems, and running simulations which contribute to advancing knowledge across numerous disciplines.
Learning MATLAB is essential for both beginners and professionals aiming to enhance their programming skills and apply their knowledge effectively in technical fields. The user-friendly interface and vast community support make it easier for newcomers to grasp its functionalities, while experienced programmers benefit from its extensive libraries. This blog post will delve into practical MATLAB examples that demonstrate its versatility and power, providing insights that can aid users in mastering this essential programming tool.
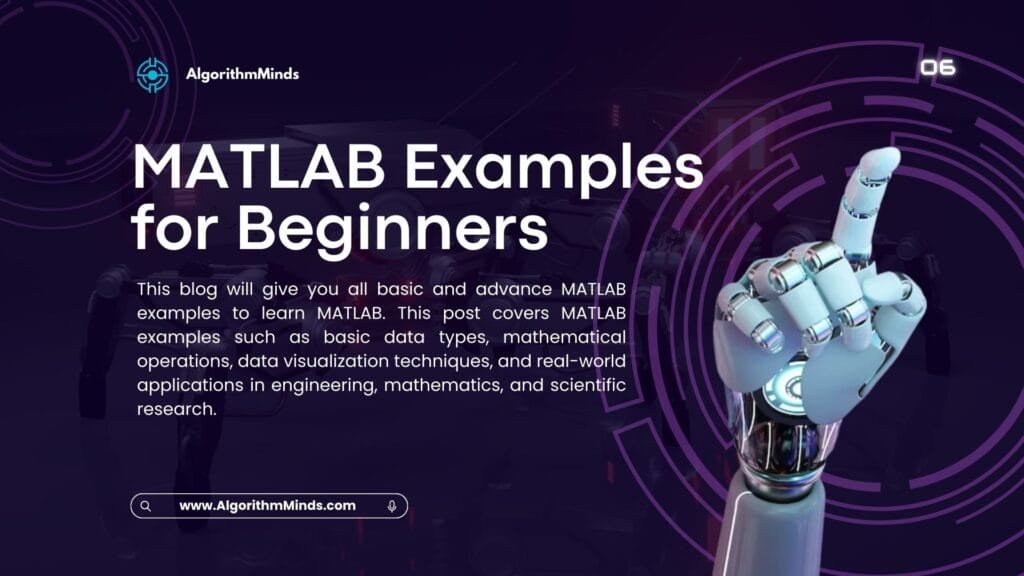
The Main Function of MATLAB
So, what is the main function of MATLAB? The primary function is to provide an interactive environment to perform numerical analysis and computational mathematics. It excels in handling matrix operations, making it an excellent tool for technical computing. Through its versatile toolbox, users can automate tasks, carry out complex calculations, and visualize the results effectively.
Exploring Five Key Uses of MATLAB
MATLAB versatility makes it a valuable tool for engineers, scientists, and mathematicians. Lets explore five key uses of MATLAB.
- Data Analysis and Visualization
One of the primary uses of MATLAB is its ability to analyze and visualize data. With built-in functions for data manipulation and graphical representation, users can create complex plots, graphs, and dashboards that reveal trends and patterns in data. This capability is essential in fields like finance, healthcare, and research.
- Algorithm Development
MATLAB provides an excellent platform for developing algorithms. Its syntax is intuitive, allowing users to prototype and test algorithms efficiently. Once the algorithms are refined, users can implement them in more extensive systems, contributing to advancements in technology.
- Engineering and Scientific Applications
Engineering disciplines heavily rely on MATLAB for tasks ranging from control system design to signal processing. Likewise, researchers in disciplines such as physics and biology find MATLAB useful for modeling complex systems and performing simulations, enhancing their understanding of various phenomena.
- Machine Learning and AI
Another significant use of MATLAB is in the realm of machine learning and artificial intelligence. Users have access to tools and functions that simplify tasks like training models, validating results, and optimizing parameters. These features are incredibly beneficial for data scientists aiming to bring AI solutions to life.
- Education and Learning
Lastly, MATLAB is widely used as a teaching tool in academic institutions. Its interactive environment helps learners grasp complex mathematical concepts and apply them practically, making it an invaluable resource for students in STEM fields.
Meet Ahsan – Certified Data Scientist with 5+ Years of Programming Expertise
👨💻 I’m Ahsan — a programmer, data scientist, and educator with over 5 years of hands-on experience in solving real-world problems using: MATLAB, Python, R, SQL, and Tableau
📚 With a Master’s in Engineering and a passion for teaching, I’ve helped countless students and researchers: Complete assignments and thesis coding parts, Understand complex programming concepts, Visualize and present data effectively
📺 I also run a growing YouTube channel — Algorithm Minds, where I share tutorials and walkthroughs to make programming easier and more accessible. I also offer freelance services on Fiverr and Upwork, helping clients with data analysis, coding tasks, and research projects.
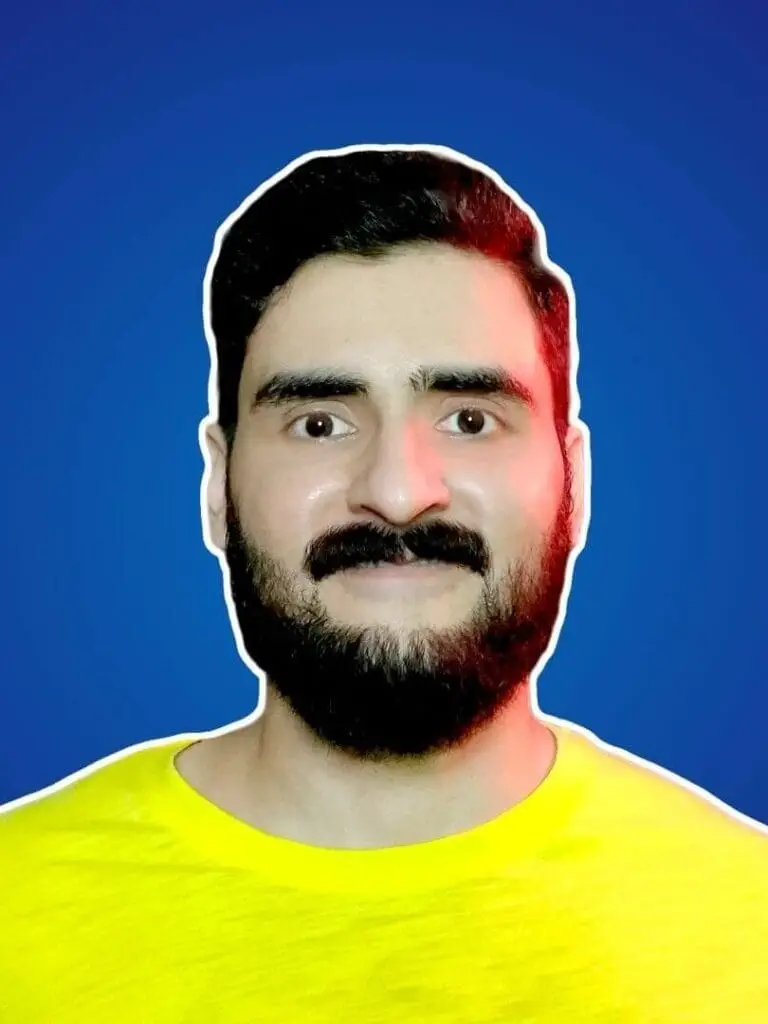
Why Clients Trust Me – Real Stories, Real Results

“Ahsan completed the project sooner than expected and was even able to offer suggestions as to how to make the code that I asked for better, in order to more easily achieve my goals. He also offered me a complementary tutorial to walk me through what was done. He is knowledgeable about a range of languages, which I feel allowed him to translate what I needed well. The product I received was exactly what I wanted and more.” 🔗 Read this review on Fiverr
Katelynn B.
Basic Data Types and Variables in MATLAB
MATLAB is a powerful programming environment designed primarily for numerical computing. Understanding the basic data types and variables is essential for effective programming within this framework. The fundamental data types in MATLAB include arrays, matrices, and cell arrays, each serving unique purposes tailored to various computational needs.
Arrays are the simplest form of data representation in MATLAB. They can be one-dimensional, such as vectors, or two-dimensional as in matrices. To create a vector, you can utilize the syntax v = [1, 2, 3, 4];
. For matrices, use semicolons to separate the rows, such as A = [1, 2; 3, 4];
. This straightforward manipulation of arrays allows for efficient data handling and mathematical operations, which are pivotal in MATLAB programming.
Cell arrays provide a more versatile data structure, allowing storage of different types of data in an array format. This is particularly beneficial when dealing with heterogeneous data. For example, you can create a cell array using C = {1, 'text', [1, 2, 3]};
, allowing you to store integers, strings, and arrays together. It is crucial to manage these variables effectively, particularly regarding memory usage. Using pre-allocation techniques, such as A = zeros(1,10);
, can improve performance by avoiding the overhead of dynamically resizing arrays.
When naming variables, adhere to best practices such as using descriptive names and avoiding spaces or special characters. Consistent naming conventions enhance readability and maintainability of the code. Overall, developing a strong understanding of the basic data types and effective variable management lays the groundwork for advancing in MATLAB programming, promoting the use of practical MATLAB examples in real-world scenarios.
Learn MATLAB with Online Tutorials
Explore our MATLAB Online Tutorial, your ultimate guide to mastering MATLAB! Whether you’re a beginner or an advanced user, this guide covers everything from basic MATLAB concepts to advanced topics
Mathematical Operations and Functions
MATLAB, a high-level programming environment, provides a powerful platform for performing a wide range of mathematical operations. The simplicity and versatility of MATLAB enable users to handle basic arithmetic with ease. Fundamental operations such as addition, subtraction, multiplication, and division can be executed using straightforward syntax. For instance, if we want to add two numbers, we can write:
A = 5 B = 3 C = A + B;
This snippet assigns the result of the addition to variable C. Likewise, you can easily perform subtraction with the minus sign, multiplication with the asterisk symbol (*), and division using the forward slash (/).
To delve deeper into more complex mathematical operations, MATLAB includes several built-in functions like interp1 that cater to an array of computations. For example, the sine, cosine, and tangent functions are integral for trigonometric calculations. The syntax used for these functions is as follows:
theta = pi/4 sin_val = sin(theta)
This example computes the sine of 45 degrees (pi/4 radians) and stores it in the variable sin_val. Moreover, the logarithmic functions, such as log and log10, allow for base e and base 10 logarithm calculations, respectively. For instance:
log_value = log(10) % Natural logarithm of 10
Furthermore, working with matrices is one of MATLAB’s strong suits. You can easily perform operations like matrix multiplication using the dot operator (.*) for element-wise multiplication or the regular operator (*) for matrix multiplication. For example:
A = [1, 2; 3, 4] B = [5, 6; 7, 8] C = A * B;
This shows how to multiply two matrices. The versatility of MATLAB not only simplifies these mathematical operations but also supports a vast range of examples that can enhance your programming skills and understanding of mathematical concepts.
Data Visualization Techniques with MATLAB Examples
Data visualization is a core aspect of data analysis that significantly enhances understanding through visual representation. MATLAB offers an array of functions designed to facilitate this process, making it easier for users to create compelling graphics that illustrate their data effectively. This section will explore various data visualization techniques using MATLAB examples that demonstrate the capabilities of its powerful plotting functions.
One of the fundamental plotting techniques in MATLAB includes 2D plotting. Using the plot
function, you can create line graphs that represent data relationships. For instance, if you have a series of data points indicating a trend over time, you can easily visualize this with a customizable plot, adding titles, axis labels, and legends for clarity. Furthermore, MATLAB supports the creation of scatter plots, which are beneficial for illustrating correlations between two variables visually. The scatter
function allows for additional features such as varying marker sizes based on a third variable, thus deepening insights drawn from the data.
Histograms are another essential technique for data visualization in MATLAB. They are particularly useful for displaying the distribution of a dataset. Utilizing the histogram
function, users can customize bin sizes and display their data in a way that highlights frequency distribution patterns. This visualization can be critical in analytical contexts where understanding data distribution is necessary for decision-making.
For three-dimensional data visualization, MATLAB provides options like surf
and mesh
to create surface and mesh plots. These techniques allow for visual exploration of complex datasets where relationships between three dimensions are essential. Through the manipulation of line styles and markers, users can enhance plot interpretations, making their findings both accessible and visually appealing.
In conclusion, mastering these data visualization techniques within MATLAB can significantly improve one’s programming and analytical skills. By implementing these approaches, one can ensure that the visual representation of data conveys a clear and comprehensive story, ultimately leading to more effective data-driven decisions.
Creating and Using Scripts and Functions using MATLAB Examples
In MATLAB, understanding the distinction between scripts and functions is crucial for efficient programming. A script is essentially a collection of MATLAB commands saved in a single file, which operates in the base workspace. This means that any variables defined within this script are accessible in the main workspace once the script is executed, allowing for straightforward data manipulation.
On the other hand, functions are more modular and are defined using the “function” keyword. They encapsulate a series of operations and can accept input arguments while returning output variables. Functions operate within their own workspace, meaning that they possess their own variables, which do not interfere with other functions or scripts. This scope management is essential to avoid potential conflicts and maintain the integrity of variables across different segments of a MATLAB program.
To create a script, start by opening a new editor window and typing your commands sequentially. For example:
x = 10 y = 20;sum = x + y disp(['The sum is: ', num2str(sum)]);
After saving this file with a .m extension (for instance, sum_script.m), you can run it by entering the file name in the command window. Conversely, to create a function, you would structure your code like this:
function result = add_numbers(a, b) result = a + b end
This function, save it as add_numbers.m, takes two inputs and returns their sum. You can call this function from the command window or another script by typing add_numbers(10, 20)
, demonstrating how functions can be reused in different contexts with varying inputs.
Variable passing is a key aspect of MATLAB functions. Arguments are passed by value, creating a copy of the data to be manipulated. However, when passing large data structures, consider passing a reference to improve efficiency. Using MATLAB examples effectively illustrates these concepts, ensuring that users can leverage scripts and functions for diverse practical applications in their programming efforts.
Basic MATLAB Functions
In MATLAB, mastering basic functions in MATLAB is essential for beginners as they form the foundation for more advanced computational tasks. Among the most commonly utilized MATLAB functions are sum
, mean
, max
, and min
. Each function serves a unique purpose and contributes to data manipulation and analysis.
The sum
function is straightforward, allowing users to compute the total of array elements. For instance, using sum(A)
where A
is an array, returns the sum of all elements in that array. It can also operate along specified dimensions, such as summing each column or row, by using syntax like sum(A, 1)
for rows and sum(A, 2)
for columns. This versatility makes it valuable for various mathematical computations.
Next, the mean
function calculates the average of array elements. The syntax mean(A)
provides the overall average, while mean(A, dim)
allows the user to specify the dimension. Understanding this function is critical for analyzing datasets, especially in determining trends and central tendencies in data.
Additionally, finding the maximum and minimum values within a dataset is crucial, and this is where the max
and min
functions come into play. Using max(A)
returns the largest element, whereas min(A)
finds the smallest. Both functions can also provide indices of the maximum or minimum values if specified, enhancing their utility in data analysis.
These basic MATLAB functions not only simplify calculations but also empower users to analyze their data effectively. Examples of practical applications abound, from statistical analysis to engineering problems, highlighting the integral role these functions play in utilizing MATLAB efficiently.
Advanced MATLAB Features and Functions Examples
As users progress in their MATLAB journey, it is essential to explore advanced features and functions that can significantly enhance the efficiency and performance of their programming tasks. One of the most powerful techniques in MATLAB is vectorization, which involves expressing operations on arrays without using explicit loops. By leveraging MATLAB’s inherent ability to handle matrix and array operations, users can improve performance dramatically, particularly when dealing with large datasets. This optimization can lead to shorter, more readable code and faster execution times. MATLAB ode45 and MATLAB diff functions effectively solve differential equations
Object-oriented programming (OOP) is another advanced feature in MATLAB worth exploring. OOP allows for the creation of objects that encapsulate both data and functions, thus promoting code reusability and organization. By defining classes and methods, programmers can structure their code more effectively, allowing for better collaboration and maintenance of complex projects. Understanding how to utilize OOP concepts in MATLAB can open up a new level of programming capability and flexibility for developers.
Moreover, MATLAB provides a variety of advanced plotting functions that can help visualize data in more insightful ways. Functions such as subplot
, surf
, and scatter3
allow users to create multidimensional plots, enhancing data presentation beyond standard two-dimensional graphs. Mastering these plotting capabilities enables users to convey their findings more effectively and engage audiences in their analysis.
For those looking to deepen their knowledge of these advanced MATLAB functions such as MATLAB interp1, numerous resources are available. Online courses, textbooks, and the official MATLAB documentation provide ample learning opportunities. Engaging with MATLAB’s community platforms for discussion and support can also facilitate continuous development. Ultimately, exploring these advanced features will not only optimize performance but will also enhance users’ overall programming capabilities in MATLAB.
Control Flow: Loops and Conditional Statements in MATLAB with Examples
Control flow constructs are essential in programming as they enable automation and decision-making within code. Loops such as MATLAB for, and MATLAB while, allow developers to execute blocks of code repeatedly based on specified conditions. Coupled with conditional statements like ‘if’, ‘else’, and ‘switch’, these control flow structures provide the building blocks for creating more complex algorithms and improving programming logic.
For instance, a ‘for’ loop can be employed to iterate through arrays efficiently, thereby allowing the programmer to perform repetitive calculations without the need for redundant code. An example of a ‘for’ loop in MATLAB might involve calculating the squares of the first ten natural numbers:
for i = 1:10square(i) = i^2;end
This snippet showcases how the loop systematically computes each square, storing the results in an array named ‘square’. In contrast, ‘while’ loops are typically used when the number of iterations is not known beforehand. A practical application could include monitoring a condition until it becomes false; for example, continuing to process data until a certain threshold is reached.
Conditional statements, on the other hand, facilitate decision-making in the code. The if statement in MATLAB checks a condition, executing a corresponding block of code only if the condition is true. For instance:
if x > 10disp('x is greater than 10');elsedisp('x is 10 or less');end
Additionally, the ‘switch’ statement is useful for selecting among multiple potential cases, enhancing code readability and organization. Utilizing these MATLAB examples not only improves the programmer’s ability to manage various conditions but also elevates the efficiency of the code. By mastering control flow, one can effectively automate repetitive tasks and implement complex algorithms, thus advancing their MATLAB programming skills significantly.
File Input and Output Operations
Effective data management is a critical aspect of programming, and MATLAB provides versatile options for file input and output operations. Understanding how to read from and write to different file formats is essential for handling data efficiently. This section will explore the various methods available in MATLAB through practical examples.
To start with text files, the ‘fopen’, ‘fgets’, and ‘fclose’ functions are fundamental. For instance, if you have a text file containing numerical data, you can read it using the following example:
fid = fopen('data.txt', 'r');data = fscanf(fid, '%f');fclose(fid);
This example opens a text file named ‘data.txt’, reads its contents into a variable, and then closes the file. Understanding such operations allows programmers to manage data flow seamlessly.
Moving on to spreadsheets, MATLAB offers a user-friendly function called ‘readtable’. This function simplifies the process of importing data directly from Excel files. For example:
T = readtable('data.xlsx');
This command efficiently loads data from ‘data.xlsx’ into a table format, making it easy to manipulate and analyze. The ability to work with spreadsheets is particularly advantageous when dealing with structured data.
Binary files offer an alternative approach for data storage and retrieval in MATLAB. The ‘fwrite’ and ‘fread’ functions can handle these operations. For example, to write an array to a binary file:
fid = fopen('data.bin', 'w');fwrite(fid, array, 'double');fclose(fid);
This command writes the variable ‘array’ to ‘data.bin’ as binary data. Reading back from a binary file is similarly straightforward and provides efficiency, especially for large datasets.
In summary, file input and output operations in MATLAB are indispensable for practical programming. By mastering these techniques and applying relevant MATLAB examples, programmers can enhance their data management skills, leading to more effective coding practices.
MATLAB Assignment Help
Expert Assistance for Homework & Projects
If you’re looking for MATLAB assignment help, you’re in the right place! I offer expert assistance with MATLAB homework help, covering everything from basic coding to complex simulations. Whether you need help with a MATLAB assignment, debugging code, or tackling advanced MATLAB assignments, I’ve got you covered.
Working with Toolboxes and User-defined Functions
MATLAB offers a range of specialized toolboxes that cater to various domains, enhancing its capabilities for specialized tasks such as image processing, signal processing, and optimization. These toolboxes provide a rich set of functions and algorithms designed to streamline complex computation and analysis tasks. Utilizing these toolboxes not only saves time but also allows programmers to leverage high-performance pre-built functions tailored for specific applications.
To access the available toolboxes, users can navigate to the “Add-Ons” menu in the MATLAB interface, which lists the toolboxes installed and those available for purchase. For instance, the Image Processing Toolbox includes functions for image enhancement, filtering, and segmentation. By loading the toolbox using the command vision.ImageFileReader
, users can begin working on image processing projects effortlessly. Similarly, the Signal Processing Toolbox offers functions for analyzing, filtering, and modeling signals.
Creating user-defined functions in MATLAB is another essential aspect of programming with this environment. These custom functions allow users to encapsulate code blocks for repetitive tasks, promoting efficient programming practices. For example, a function can be created to calculate the mean and standard deviation of a data set. The syntax to define a function is straightforward, such as using function [output]
to start the function and specifying the input parameters. This modular approach not only enhances code organization but also facilitates debugging and testing.
Moreover, MATLAB’s extensive documentation and active user community provide ample resources and examples for users to explore. By employing these toolboxes and user-defined functions, programmers can greatly improve their MATLAB programming abilities, making their work more effective and productive. Engaging with practical MATLAB examples further aids in cementing these concepts and skills.
Real-World Applications of MATLAB
MATLAB is a versatile tool often employed in various industries to address complex challenges through its robust computational capabilities. One prominent application is in the field of simulations. MATLAB allows engineers and scientists to create detailed simulations of physical systems, enabling them to study behavior over time under different conditions. This is particularly valuable in fields such as aerospace, automotive, and biomedical engineering, where predicting system performance before actual implementation is crucial.
Control systems represent another significant application of MATLAB. It provides users with sophisticated tools for designing and analyzing dynamic systems. Control engineers utilize MATLAB to model system dynamics, design control strategies, and simulate system response. This functionality is crucial in industries where stability and performance are critical, such as robotics and manufacturing processes. The ability to visualize system behavior through MATLAB’s graphing capabilities aids in fine-tuning system parameters for optimal performance.
In recent years, machine learning applications have gained momentum, with MATLAB emerging as a valuable resource. It offers a rich set of functions and toolboxes dedicated to developing, training, and testing machine learning algorithms. Researchers and data scientists leverage MATLAB for predictive analytics, classification tasks, and data clustering, making it easier to derive insights from large datasets. This capability is increasingly important in sectors such as finance, healthcare, and technology, where data-driven decision-making is paramount.
Data analysis is yet another domain where MATLAB shines. Its powerful data manipulation and visualization tools enable users to conduct in-depth analyses and gain meaningful insights from their data. The platform supports various statistical methods and can handle large datasets efficiently, allowing users to extract relevant information quickly. This aspect of MATLAB is particularly beneficial for professionals in marketing research, environmental science, and social sciences, where data interpretation plays a critical role in shaping strategies and policy decisions.
In conclusion, the diverse applications of MATLAB in simulations, control systems, machine learning, and data analysis showcase its relevance across multiple fields, making it an indispensable tool for professionals seeking to enhance their programming skills.
Tips for Maximizing Learning from MATLAB Examples
To effectively maximize your learning from MATLAB code examples PDF, it is essential to engage actively with the material rather than passively consuming it. One of the most effective strategies is to practice coding regularly. By downloading MATLAB code PDF files and running the examples on your own, you can solidify your understanding. It is recommended to replicate the examples initially, ensuring that you understand each line of code. Afterward, you may try modifying certain parameters to observe how these changes affect the output, which can provide insights into the functionalities of MATLAB.
Another valuable tip is to embrace a hands-on approach to utilize MATLAB’s built-in functions and commands. Instead of merely relying on predefined examples, experiment by creating your own scripts from scratch. This encourages problem-solving and nurtures creativity. Look for specific areas within the provided MATLAB code examples PDF that intrigue you and challenge yourself to apply those concepts to different projects.
Additionally, consider integrating online resources into your learning journey. Websites, forums, and communities focused on MATLAB can offer further insights and support. Platforms like MATLAB Central or Stack Overflow can be invaluable for seeking help when encountering obstacles. Engage with these communities by asking questions or exploring existing discussions related to your interests. This interaction not only provides additional knowledge but also exposes you to diverse problem-solving strategies.
Finally, set realistic learning goals and periodically assess your progress. Establish milestones for what you wish to achieve, whether it is mastering a specific set of functions or completing a particular project using the MATLAB examples. Keeping your learning targeted will enable you to gain confidence in your skills and knowledge.
Getting Started with MATLAB Examples: Writing Your First Program
Writing Your First MATLAB Program
x = 4;
y = 7;
z = x + y
Output:
11
Explanation:
- A semicolon (
;
) at the end of a line prevents MATLAB from displaying output. - Since
z = x + y
is not followed by a semicolon, MATLAB displays its value.
Understanding Variable Assignment
p = 10;
q = p;
q
Output:
10
Explanation:
- The variable
p
stores the value10
. - Assigning
p
toq
meansq
also holds10
, butp
remains unchanged.
Performing Basic Mathematical Operations
m = 5;
n = 8;
result = 3*m + n^2 - m*n + n/m - 7
Output:
52.6
Explanation:
- The expression includes addition (
+
), subtraction (-
), multiplication (*
), division (/
), and exponentiation (^
). - The right-hand side is evaluated first, and the result is assigned to
result
.
Updating a Variable
x = 6;
x = x + 2;
x
Output:
8
Explanation:
x is initially 6. The second statement adds 2 to the existing value of x, updating it to 8.
Printing Text Output
fprintf('Welcome to MATLAB!\n')
Output:
Welcome to MATLAB!
Explanation:
The fprintf function prints formatted text.
The \n at the end adds a new line after printing.
Using Formatted Output for Numbers
a = 5;
b = a^2;
c = a^3;
d = sqrt(a);
fprintf('%4d squared is %4d \n', a, b)
fprintf('%4d cubed is %4d \n', a, c)
fprintf('The square root of %d is %.3f \n', a, d)
5 squared is 25
5 cubed is 125
The square root of 5 is 2.236
Explanation:
%4d ensures proper alignment by reserving 4 spaces for integers.
%.3f formats a floating-point number to three decimal places.
Creating and Manipulating Arrays
x = [2 5 8];
y = [3 6 1];
z = x + y
Output:
5 11 9
Explanation:
Arrays are added element-wise: [2+3, 5+6, 8+1].
Extracting an Array Element
arr1 = [4 7 9];
arr2 = [2 5 6 8];
sum_value = arr1(2) + arr2(4)
Output:
sum_value = 15
Explanation:
arr1(2) is the second element of arr1, which is 7.
arr2(4) is the fourth element of arr2, which is 8.
Their sum (7 + 8) is 15.
Adding Comments in MATLAB
%
% This script demonstrates the use of comments
%
x = 5;
y = x^2;
%
% y = 2 * y; % This line is ignored by MATLAB
%
z = x + y
Output:
z = 30
Explanation:
Comments start with %, and MATLAB ignores these lines during execution
Splitting a Long Line
sum_numbers = 2 + 4 + 6 ...
+ 8 + 10
Output:
30
Explanation:
The three dots (...) allow a command to continue on the next line.
Removing a Variable Using clear
a = 10;
b = a * 2;
clear a
disp(a)
Output:
??? Undefined function or variable 'a'.
Explanation:
The clear command removes a from memory, making it inaccessible. However, b remains unaffected because it was already assigned a value before a was cleared. This command is useful when working with large arrays that are no longer needed, as it helps free up memory.
Using Built-in Mathematical Functions and Constants
theta = pi;
val1 = cos(pi/3);
val2 = log(exp(-cos(pi/3)));
Output:
val1 = 0.5000
val2 = -0.5000
Explanation:
MATLAB provides intrinsic constants like pi and various mathematical functions. Here, cos(pi/3) evaluates to 0.5, and the second expression simplifies to log(exp(-0.5)), which results in -0.5. The innermost function is evaluated first.
Understanding Variable Naming Rules
Variable1 = 25;
variable1 = 50;
data_set_2024 = Variable1 + variable1;
Output:
data_set_2024 = 75
Explanation:
MATLAB is case-sensitive, meaning Variable1 and variable1 are treated as separate variables. Also, underscores and numbers can be used in variable names, but reserved keywords (like pi) should not be reassigned.
Generating a Simple Plot
x_vals = 0:0.05:10;
y_vals = cos(x_vals);
plot(x_vals, y_vals, 'b-', 'LineWidth', 2)
xlabel('X Values')
ylabel('Cosine of X')
title('Cosine Function Plot')
grid on
Explanation:
x_vals
creates an array from0
to10
with steps of0.05
.y_vals
computes the cosine values ofx_vals
.- The
plot
function draws a blue line ('b-'
) with a thickness of2
. - Labels, a title, and a grid are added to enhance readability.
Basic for MATLAB Loop
x = 5;
for i = 1:4
disp(x);
end
Output:
5
5
5
5
Explanation: This is a basic for loop in MATLAB where the value of x is printed 4 times. The loop runs from i = 1 to i = 4 but the value of x doesn't change inside the loop. The disp() function is used to display the value of x on the command window.
Nested Loop (Double Loop)
total = 0;
for i = 1:3
for j = 1:2
total = total + i*j;
end
end
disp(total);
Output:
18
Explanation: In this example, we have two nested loops. The outer loop runs for i = 1 to i = 3 and the inner loop runs for j = 1 to j = 2. On each iteration, the product of i and j is added to the total. The final result is the sum of all the products.
Tips for Effective MATLAB Programming
Mastering MATLAB programming involves not only familiarity with its syntax but also an understanding of best practices that enhance code quality and efficiency. One key aspect of effective MATLAB programming is adopting a structured approach to code organization. By utilizing functions, scripts, and organized files, you can create readable and maintainable code. Utilize descriptive file names and keep related code within the same directory. This practice simplifies navigation, especially when you plan to download MATLAB examples for reference.
Debugging is another critical component of writing efficient MATLAB code. Employ the MATLAB built-in debugging tools, such as breakpoints, the Step In/Out features, and the Command Window, to isolate issues in your code. Carefully checking variable values and understanding error messages will greatly assist in troubleshooting. Furthermore, writing unit tests for your functions can catch errors early, helping you avoid problems when running more complex MATLAB examples with solutions.
Commenting your code is essential for readability and collaboration. Providing comments that explain the purpose and logic behind your code helps others (and yourself, in the future) to understand its functionality quickly. This is particularly useful when sharing simple MATLAB code examples within a team or classroom environment. In addition, it promotes good programming habits by encouraging you to clarify your thought process while coding.
Performance optimization is vital, particularly for large-scale computations. Ensure that you preallocate matrices instead of growing them dynamically during execution, as this can significantly reduce computation time. Utilize vectorization when possible, as MATLAB is optimized for handling arrays and matrices more efficiently than explicit loops. In this regard, seeking out MATLAB codes that implement efficient techniques can serve as valuable learning tools.
To enhance your MATLAB programming skills, consider exploring resources available online or through MATLAB’s own documentation. With practice and adherence to the tips outlined above, you can develop a solid foundation in MATLAB programming that will propel you toward more advanced projects and applications.
Utilizing the Downloadable PDF of MATLAB Examples
Downloading the MATLAB code PDF containing various examples is a straightforward process designed to enhance your learning experience. The first step involves locating the designated download link provided on the blog. Typically, this link is prominently displayed within the text or in the sidebar for easy access. Once you find the link, simply click on it, and your browser will initiate the download process.
Upon clicking the link, your browser will usually prompt you to confirm the download. If you have chosen to save the file, select the preferred location on your device where you would like the MATLAB code examples PDF to be stored. This functionality allows for quick access to the file whenever you need it, contributing to a seamless learning experience. For users who prefer to view the document as soon as it finishes downloading, you may also opt to open it directly from the browser window.
Having the MATLAB examples in PDF format offers numerous benefits. Firstly, it affords you the opportunity to access the material offline, allowing you to study and practice regardless of your internet connectivity. Secondly, the PDF format makes it easy to print out specific sections of the MATLAB code examples PDF, which can be particularly useful for hands-on practice or annotations. Furthermore, most PDF readers offer functionalities that enable users to highlight text, make notes, and even bookmark essential sections for future reference.
These features not only enhance the learning process but also ensure that the material is always available for quick review. Thus, downloading the MATLAB examples PDF is an invaluable step for anyone looking to deepen their understanding of MATLAB programming through practical examples.
Expert Programming Assignment Help
Struggling with coding concepts or looming deadlines? Our vetted programming specialists deliver precision guidance across core languages. We provide Python homework help for algorithms and debugging, C++ assignment support for memory management and OOP, and Java coursework solutions from syntax to advanced frameworks. Each solution includes clear explanations of underlying principles—not just answers—to strengthen your real-world coding skills.
Data Science & Visualization Assignment Experts
Tackle technical coursework with confidence. We offer specialized support for data-driven tools: Excel assignment help for complex formulas, macros, and data analysis, Tableau assignment help for dashboard design and data storytelling, MATLAB assignment help for simulations and matrix operations, and R programming help for statistical modeling and data analysis. Every project we assist with reflects academic precision and practical insight, helping your submissions stand out both in clarity and quality.
Ready to elevate your work? Let’s take your assignments to the next level.