Hands-On MATLAB Programming Examples
MATLAB Tutorials
To get started with MATLAB, you are encouraged to explore the extensive array of online resources, including official documentation, forums, and readily available online MATLAB tutorials on platforms like YouTube. These resources on our website can guide beginners through the learning curve, allowing them to unlock the full potential of MATLAB. As you progress, you will discover how this powerful tool can significantly enhance your analytical and problem-solving skills.
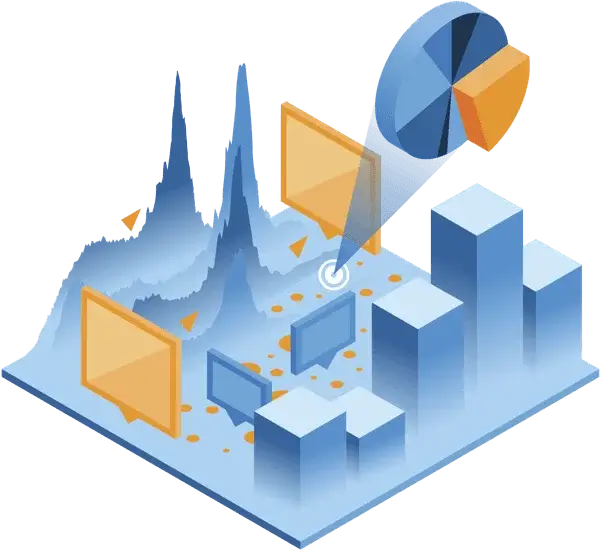
Introduction to MATLAB
MATLAB, short for Matrix Laboratory, is a high-level programming language and interactive environment developed by MathWorks. It is primarily used by engineers and scientists for various applications, including data analysis, visualization, and algorithm development. With its intuitive interface and ability to handle complex mathematical computations, MATLAB has become an essential tool in academia and industry alike.
The significance of MATLAB in engineering and science cannot be overstated. It enables users to perform complex calculations and visualize results seamlessly. The primary strength of MATLAB lies in its extensive library of built-in functions and toolboxes tailored for specific applications, such as signal processing, control systems, and machine learning. This versatility allows users to tackle a wide range of problems, transforming theoretical concepts into practical applications.
For beginners, understanding the basic features of MATLAB is crucial. The interface typically includes a command window, a workspace to manage variables, and a variety of tools for file management and code editing. Users can execute commands directly from the command window, making it an excellent starting point for hands-on learning. Familiarizing oneself with fundamental components, such as variables, matrices, and scripts, is essential for navigating MATLAB effectively.
Additionally, the rich visualization capabilities, including graphs and plots, enhance data interpretation and provide insights that are often vital in decision-making processes.
Meet Ahsan – Certified Data Scientist with 5+ Years of Programming Expertise
👨💻 I’m Ahsan — a programmer, data scientist, and educator with over 5 years of hands-on experience in solving real-world problems using: MATLAB, Python, R, SQL, and Tableau
📚 With a Master’s in Engineering and a passion for teaching, I’ve helped countless students and researchers: Complete assignments and thesis coding parts, Understand complex programming concepts, Visualize and present data effectively
📺 I also run a growing YouTube channel — Algorithm Minds, where I share tutorials and walkthroughs to make programming easier and more accessible. I also offer freelance services on Fiverr and Upwork, helping clients with data analysis, coding tasks, and research projects.
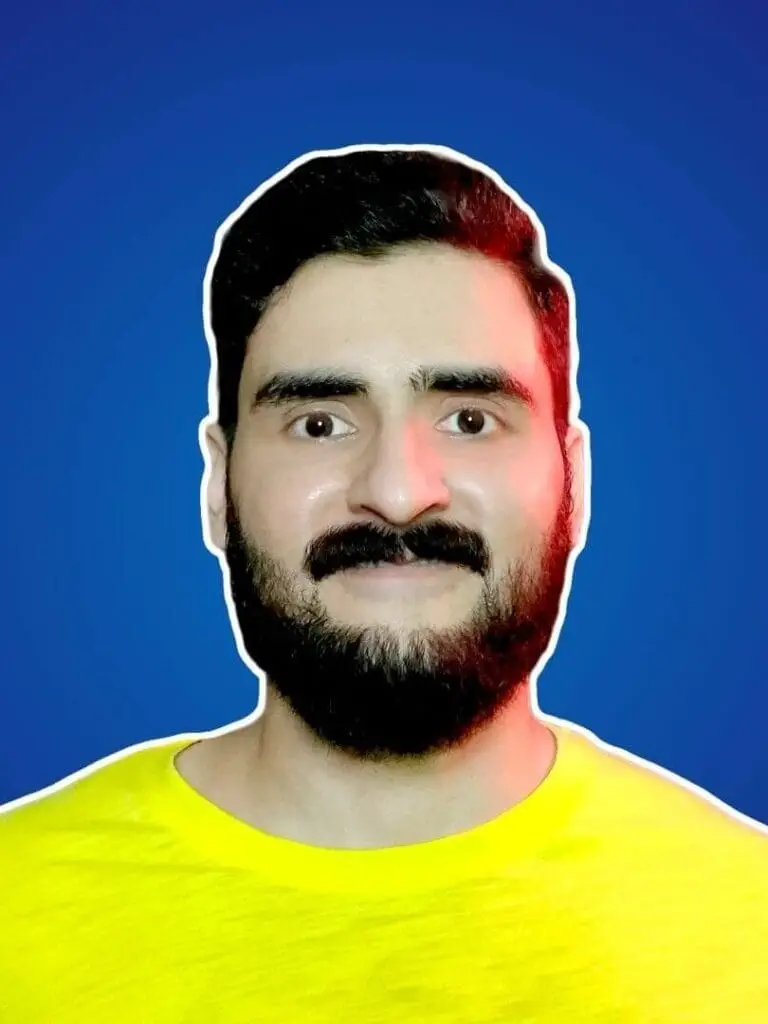
Why Clients Trust Me – Real Stories, Real Results

“Ahsan completed the project sooner than expected and was even able to offer suggestions as to how to make the code that I asked for better, in order to more easily achieve my goals. He also offered me a complementary tutorial to walk me through what was done. He is knowledgeable about a range of languages, which I feel allowed him to translate what I needed well. The product I received was exactly what I wanted and more.” 🔗 Read this review on Fiverr
Katelynn B.
The importance of MATLAB for students cannot be overstated, as it provides a robust environment for learning and applying complex mathematical concepts and computational techniques. The capabilities of MATLAB extend beyond mere computations. Engineers leverage MATLAB to create dynamic models that facilitate simulations and visualizations, allowing them to understand behaviors over time or under varying conditions.
The utilization of MATLAB for finance is increasingly prevalent as firms recognize the importance of adopting programming solutions to enhance their analytical capabilities. Financial analysts and quantitative developers harness MATLAB’s extensive toolbox to perform portfolio optimization, risk management assessments, and profitability analysis.
Choosing between MATLAB and Octave ultimately depends on the specific needs of individuals or organizations. For those involved in large-scale projects or in need of commercial support, MATLAB may be the better choice.
Basic MATLAB Commands and Syntax
MATLAB (Matrix Laboratory) is a powerful programming environment widely used for numerical computation, data analysis, and algorithm development. Understanding the basic commands and syntax is crucial for anyone looking to dive into the world of MATLAB tutorials. In this segment, we will explore essential commands, data types, and array manipulation techniques.
MATLAB commands are typically structured as single-line scripts or functions. A common first command is clc
, which clears the command window, making it easier to read new outputs. The clear
command removes variables from the workspace, while close all
closes any open figure windows. These introductory commands help maintain a clean working environment.
In terms of data types, MATLAB primarily deals with matrices and arrays. The basic data type is a numeric array, which can be created using the syntax A = [1, 2, 3; 4, 5, 6]
. This creates a 2×3 matrix. Other types include strings, cell arrays, and structures, each serving distinct purposes in different types of computations.
Array manipulation is fundamental in MATLAB programming. To access an element in the array, you can use the syntax A(1,2)
, which retrieves the element located in the first row and second column of matrix A
. To manipulate arrays, common operations include sum(A)
for summing elements and A'*A
for matrix multiplication. Mastering these commands is essential for executing more complex tasks in MATLAB.
As you become familiar with these foundational commands and syntax through various MATLAB tutorials, you will build a solid groundwork upon which more advanced programming skills can be developed.
Basic MATLAB Guide & 60 MATLAB Examples for Beginners
The relevance of basic MATLAB in the fields of data science and programming cannot be understated. Data scientists leverage MATLAB for its robust computational capabilities and its extensive toolboxes, which can handle a variety of tasks, from data analysis and image processing to machine learning and simulation.
This Guide will give you all basic and advance MATLAB examples to learn MATLAB. This post covers MATLAB examples such as basic data types, mathematical operations, data visualization techniques, and real-world applications in engineering, mathematics, and scientific research.
for Loop in MATLAB – Practical Tutorials
Understanding control flow and structures is crucial for programming efficiently in MATLAB. Control flow dictates the order in which statements are executed, which is essential for writing effective algorithms and functions. The primary control structures in MATLAB include loops and conditional statements, which allow for the implementation of complex logic in code.
One of the foundational constructs for iterative operations is the for loop. This loop allows a specific section of code to execute multiple times, making it useful for tasks that require repetition. For instance, consider a simple example where we want to calculate the sum of the first ten natural numbers. A for loop can efficiently handle this task:
sum = 0 for i = 1:10 sum = sum + i end disp(sum)
In this example, the loop iterates over the numbers from 1 to 10, cumulatively adding each number to the variable sum. Using MATLAB tutorials, learners can dive deeper into such iterations, refining their skills in developing algorithms that rely on repetitive tasks.
For loops stand as one of the foundational constructs of programming within MATLAB, playing a crucial role in automating repetitive tasks. Whether one is a data scientist analyzing complex datasets, an engineer developing simulations, or a student honing their programming skills, understanding for loops is vital.
This iterative control structure allows users to execute a predefined set of statements a specific number of times, thus enhancing efficiency and reducing the likelihood of errors commonly associated with manual data manipulation.
Tutorials for While Loop in MATLAB
On the other hand, the while loop is designed to execute statements as long as a specified condition remains true. This makes it effective for scenarios where the number of iterations is unknown beforehand. Here is a basic example demonstrating a while loop to find the first ten even numbers:
count = 0 num = 1 while count <10 num = num + 1 if mod(num, 2) == 0 disp(num) count = count + 1 end end
By reading of this guide, readers can expect to develop a comprehensive understanding of while loops in MATLAB. They will learn when to use while loops, how to structure them effectively, and practical tips for debugging and optimization, enabling them to apply these concepts in their respective fields of work or study.
If Else Statement in MATLAB
Conditional statements, such as if and switch, are equally important in guiding the flow of execution based on specific conditions. An if statement allows execution of a block of code only if a condition evaluates to true. For instance, a simple piece of code to evaluate whether a number is positive or negative can be written as:
number = -3 if number >0 disp('Positive') else disp('Negative'); end
Most programming languages, including MATLAB, offer various types of conditional statements—among the most common being the If-Else statement. This specific construct not only enables the execution of alternate code paths but also improves code readability, making it easier to understand how decisions are being made within a program. Learn all about If Else Statement in MATLAB in the following blog
MATLAB Assignment Help
If you’re looking for MATLAB assignment help, you’re in the right place! I offer expert assistance with MATLAB homework help, covering everything from basic coding to complex simulations. Whether you need help with a MATLAB assignment, debugging code, or tackling advanced MATLAB assignments, I’ve got you covered.
Why Trust Me?
- Proven Track Record: Thousands of satisfied students have trusted me with their MATLAB projects.
- Experienced Tutor: Taught MATLAB programming to over 1,000 university students worldwide, spanning bachelor’s, master’s, and PhD levels through personalized sessions and academic support
- 100% Confidentiality: Your information is safe with us.
- Money-Back Guarantee: If you’re not satisfied, we offer a full refund.
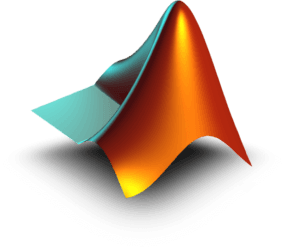
MATLAB Matrix and Array Operations – Tutorial for Beginners
In this guide, we dive deep into MATLAB matrix and array operations from mathworks company, a fundamental skill for anyone working with data or performing numerical analysis. Whether you’re just getting started or looking to strengthen your MATLAB skills, this video covers everything you need to know about constructing and manipulating matrices and arrays. You’ll learn:
- Constructing matrices with various data types and structures.
- How to use specialized matrix functions like zeros, ones, and diag to create custom matrices.
- Techniques for concatenating matrices to build larger data sets.
- Generating numeric sequences efficiently with the colon operator.
- How to expand matrices by adding rows or columns.
- Removing rows or columns from matrices for data manipulation.
- Reshaping and rearranging arrays to fit your analysis needs.
- Mastering multidimensional arrays and understanding their use in MATLAB.
- Array indexing, including both linear and logical indexing, for precise data access and manipulation.
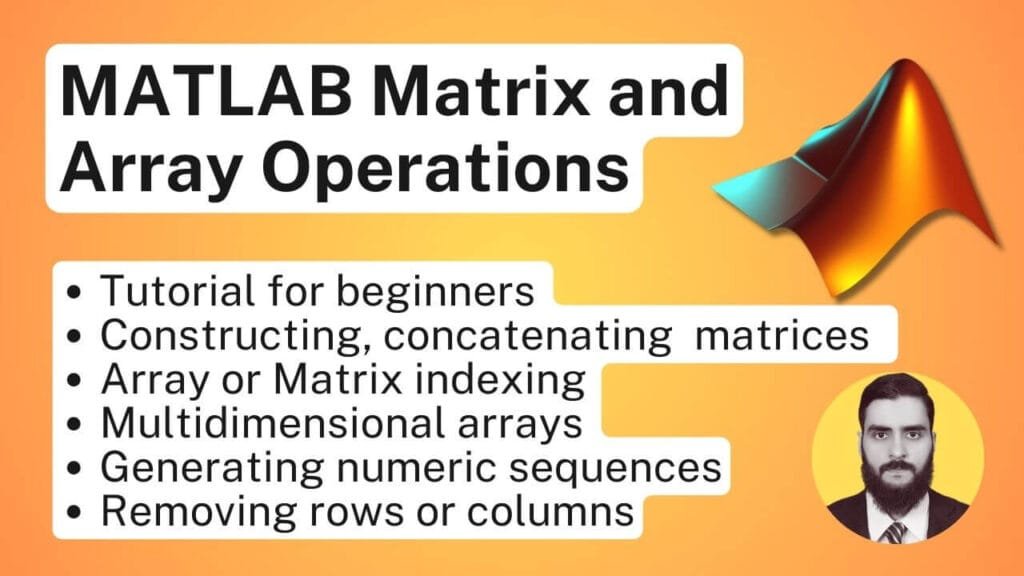
MATLAB Matrix and Arithmetic Operations
In this guide, we dive deep into essential mathematical concepts and how to implement them using MATLAB, covering everything from basic matrix addition and subtraction to more advanced topics like matrix inverse, determinant, and eigenvalues. Whether you’re new to MATLAB or looking to strengthen your understanding of linear algebra in computational settings, this video has you covered. We’ll walk through:
- Adding and Subtracting Matrices: Learn how to perform element-wise matrix operations.
- Vector Products & Transpose: Understand the connection between vectors and how to manipulate them.
- Multiplying Matrices: Master the process of matrix multiplication and its importance in mathematical computations.
- Matrix Inverse & Determinant: Discover how to compute matrix inverses and determinants, crucial for solving systems of equations.
- Solving Systems of Linear Equations: See how MATLAB simplifies solving linear systems using matrix operations.
- Eigenvalues: Explore the significance of eigenvalues in matrix theory and their role in various applications.
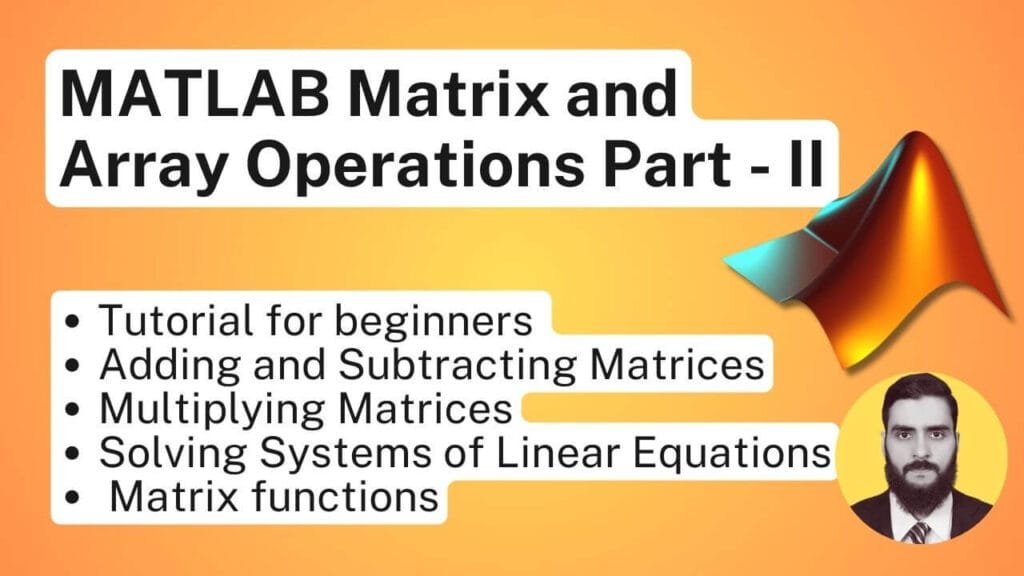
Functions in MATLAB – Online MATLAB Tutorials
In this guide, we’ll explore the core concepts of function basics in MATLAB from mathworks company, including the syntax for defining functions, how to create function handles, and how to pass functions to other functions. This video will help you gain a solid foundation in using functions effectively in MATLAB, setting you up for success in your projects.
If you’re working on MATLAB programming or need to optimize your code with function handles, this tutorial is packed with practical tips and examples you can apply immediately. You’ll learn how to:
- Understand fundamental MATLAB classes and their role in function creation.
- Master the syntax for defining MATLAB functions to streamline your coding process.
- Learn how to create function handles and pass functions as arguments to other functions.
- Discover how to call local functions using a function handle for better code organization and efficiency.
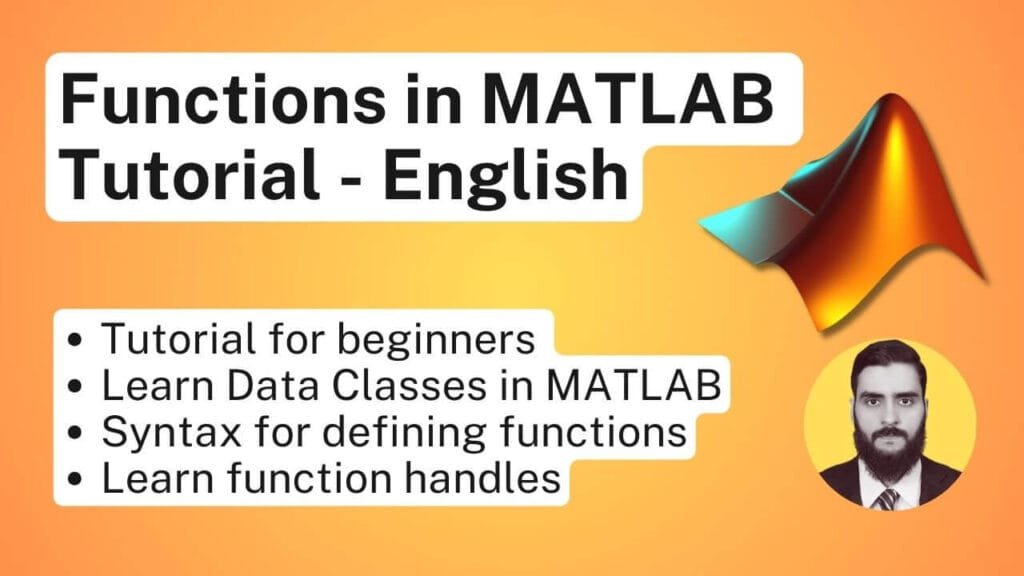
LOOPs in MATLAB – While and For Loop Programming
In this in-depth tutorial, we explore the core concepts of loops like while and for in MATLAB from the mathworks, with practical examples for every beginner to expert level. Whether you’re looking to understand the structure of for loops, while loops, or how to leverage the break and continue statements to control flow, this guide covers it all! What you’ll learn:
- For Loop Example: Learn how to use the for loop to repeat a block of code a specific number of times.
- While Loop Example: Master the while loop for executing code as long as a condition is true.
- Break Statement: See how to exit loops early with the break statement when certain conditions are met.
- Continue Statement: Learn how to skip over parts of your loop with the continue statement.
- Nested Loops: Understand how to work with nested loops for more complex operations and data processing.
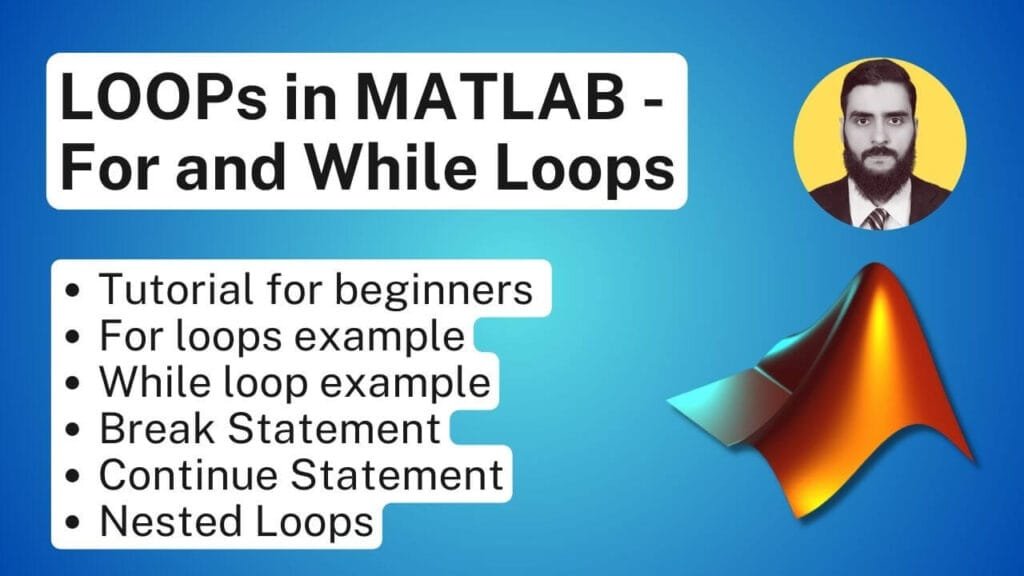
if, else, elseif in MATLAB – Conditional Statements Tutorials
In this guide, we break down the core conditional structures in MATLAB from mathworks inc, which are essential for controlling the flow of your code. If you’re new to MATLAB or programming in general, this is the perfect place to start. You’ll understand how conditional logic powers decisions and drives functionality in your MATLAB projects. You’ll learn through detailed examples how to use:
- if…end structure: Master the basics of conditional statements and make decisions in your code.
- if…else structure: Explore how to introduce alternative actions when a condition isn’t met.
- if…elseif…elseif…else…end structure: Dive deeper into multiple condition checks and refine your logic.
- Nested if structures: Discover how to build more complex decision-making processes within other if statements.
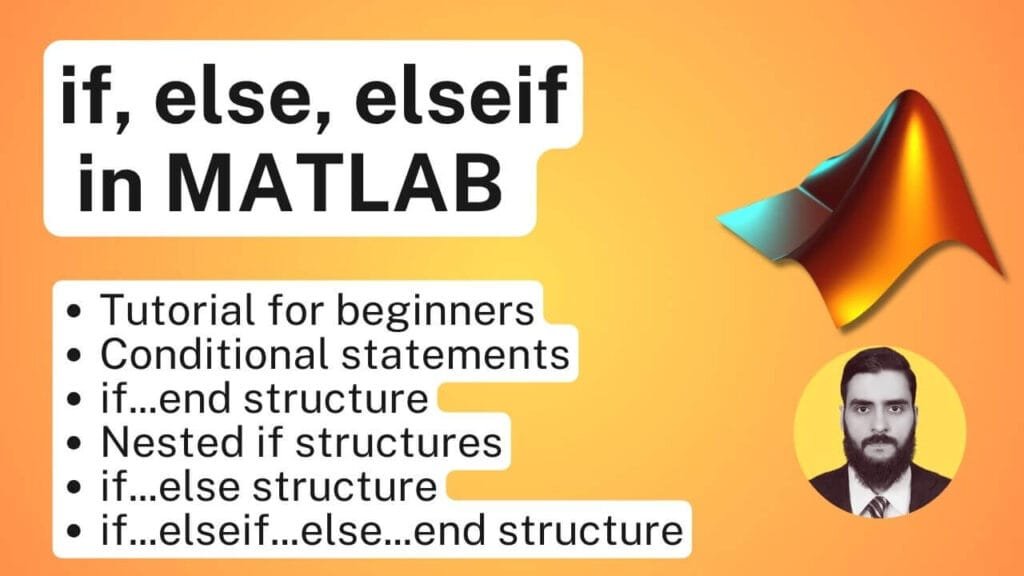
How to Plot Graph in MATLAB | Plotting Function Tutorials in MATLAB
Want to master plotting in MATLAB? In this video, we dive into the different types of MATLAB plots and how to create them, covering everything from basic line plots to more advanced visualizations. Whether you’re new to MATLAB or looking to sharpen your plotting skills, this tutorial will guide you through the essential techniques for data visualization.
This step-by-step tutorial not only teaches you the basics of MATLAB plotting, but also introduces advanced techniques to help you create professional-looking graphs that enhance your data analysis projects. Perfect for students, data scientists, and engineers alike! What you’ll learn:
- How to create 2-D line plots for simple data visualization
- How to use bar plots to visualize categorical data
- Explore polar plots for displaying data in a circular format
- Learn about stem plots to highlight data points in a visually appealing way
- Create scatter plots for representing relationships between variables
- Plot multiple lines on the same graph for comparison
- Customize line plots with markers for more informative visuals
- Combine two plots to create complex visualizations
- How to effectively plot histograms to analyze data distributions
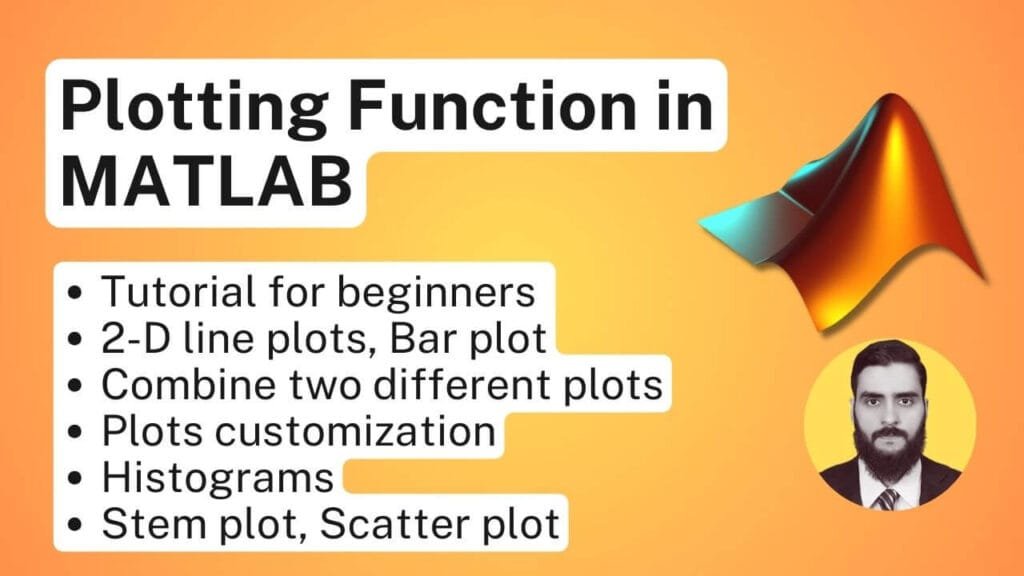
Solve Differential Equations in MATLAB using ode45, diff, and dsolve
In this guide, we dive deep into solving ordinary differential equations (ODEs) in MATLAB online, focusing on three powerful tools: diff dsolve and ode45. Whether you’re new to MATLAB or looking to enhance your skills, this tutorial covers the essential theory behind ODEs, as well as practical demonstrations on how to apply these functions to real-world problems. This tutorial is perfect for students, engineers, and anyone looking to get a deeper understanding of solving ODEs in MATLAB.
MATLAB ode45 function Tutorial
The ease of use and integration of Ode45 into MATLAB environments further cements its status as a preferred choice among practitioners. With intuitive syntax and built-in features, users can quickly model and solve ODEs, leading to more efficient workflows in their data science tasks. Understanding the functionalities offered by Ode45 is a crucial step for anyone looking to leverage numerical solutions for ordinary differential equations in their programming and research endeavors.
MATLAB diff function Tutorial
Moreover, MATLAB offers powerful tools for differentiation through MATLAB diff function, enabling users to compute derivatives analytically and numerically. This allows professionals and students alike to practically apply the concepts of differentiation in their projects, thereby bridging the gap between theory and real-world application.
In this guide, we will cover:
- A detailed introduction to ordinary differential equations (ODEs) theory and their importance in mathematics and engineering.
- How to solve ODEs symbolically using MATLAB’s dsolve function, and understand the solutions it provides.
- The basics of the ode45 solver in MATLAB, a versatile function for solving complex numerical differential equations and initial value problems (IVPs).
- Step-by-step demonstrations of applying ode45 for solving first-order and second-order differential equations, including systems of ODEs.
- Tips for choosing the best MATLAB ODE solver based on your problem, including using ode45 for accuracy and speed.
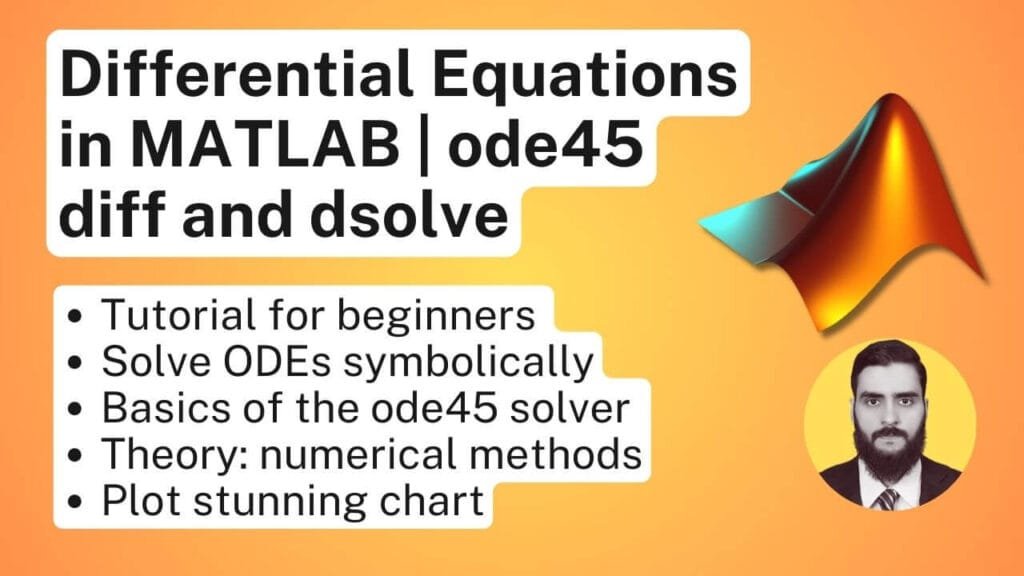
MATLAB Tutorials for Integration – Finding Definite and Indefinite Integrals
In this tutorial, we dive deep into the process of finding both indefinite and definite integrals using MATLAB. This guide provides a hands-on approach, walking you through MATLAB commands and techniques that will help you solve complex integration problems and plot the equations effortlessly. You’ll learn how to:
- Find indefinite integrals using MATLAB’s symbolic toolbox.
- Compute definite integrals and evaluate them over a specified range.
- Plot mathematical equations to visualize the integral results clearly.
- Understand how to calculate the integral of polynomials and how MATLAB handles these functions.
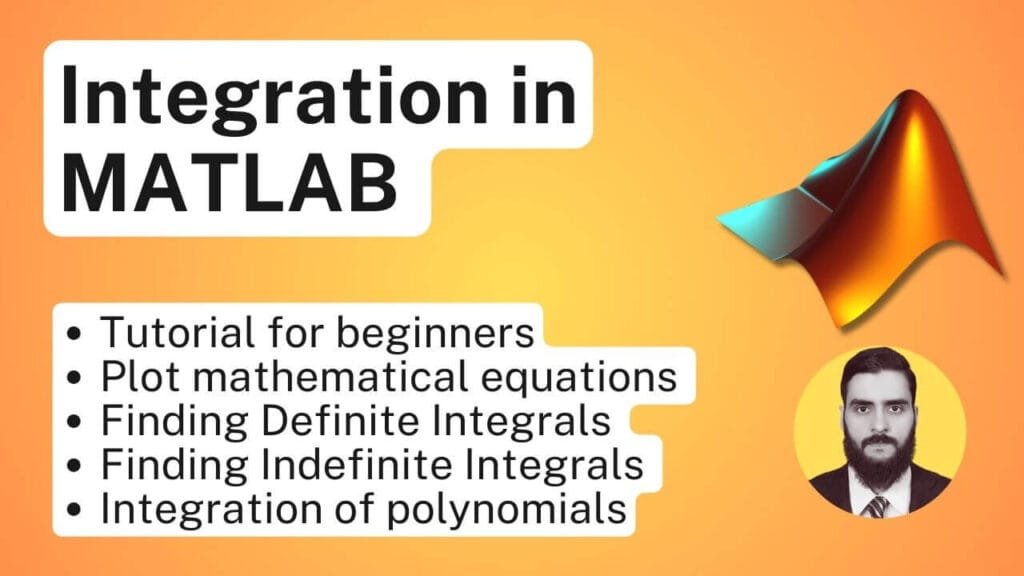
Solving System of Equations in MATLAB
In this tutorial, we’ll dive deep into solving systems of equations in MATLAB, focusing on powerful techniques like symbolic calculation, expanding and collecting equations, and factorization and simplification of algebraic expressions. You’ll have the knowledge and tools to solve systems of equations, simplify complex algebraic expressions, and use MATLAB to handle advanced symbolic math tasks with ease. We’ll cover:
- Symbolic Math Toolbox in MATLAB: Learn how to perform symbolic calculations for solving equations.
- Expanding and Collecting Equations: Understand how to expand and collect terms in an equation for easier manipulation.
- Factorization and Simplification: Discover how to factor and simplify algebraic expressions to find solutions more efficiently.
- Practical Applications: Get tips and tricks for real-world problem solving with MATLAB’s symbolic capabilities.
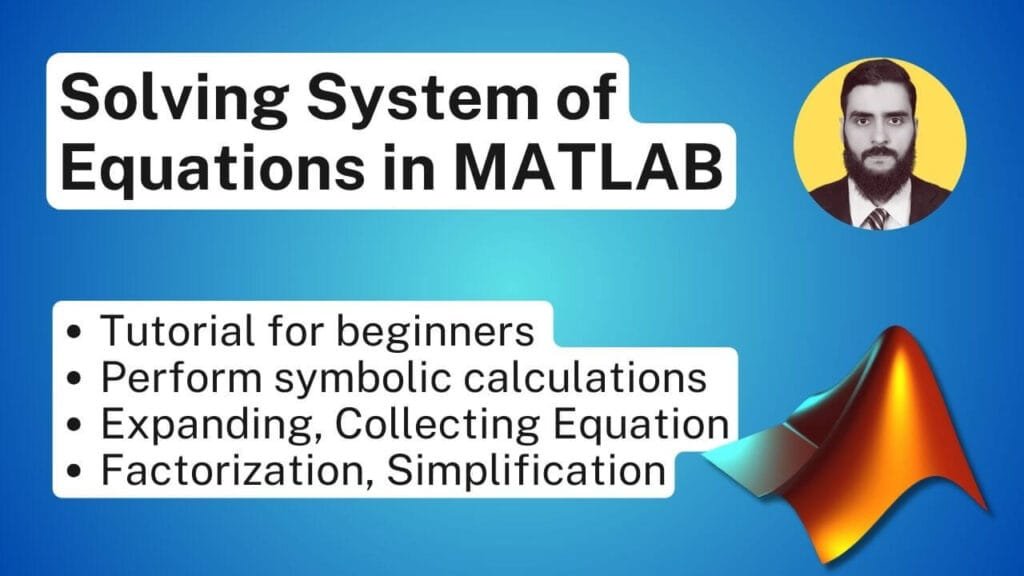
Python in MATLAB Tutorial
The integration of Python in MATLAB represents a significant advancement for data scientists and programmers seeking to expand their capabilities. By utilizing Python within the MATLAB environment, users can leverage the strength of both languages, creating a symbiotic relationship that enhances overall productivity. By integrating Python in MATLAB, users can seamlessly access these libraries, thereby enriching their analytical toolkit. Learn how to integrate Python in MATLAB
Plotting Tutorials in MATLAB
Data visualization is a critical component in interpreting results and communicating information effectively, and MATLAB offers a robust set of tools and functionalities for this purpose. The software’s capabilities allow users to create a variety of graphical representations ranging from simple line plots to complex 3D surfaces. At the foundation of MATLAB tutorials on data visualization are the basic plotting functions, which serve as the primary means to display data in an intuitive manner.
For instance, the plot
function generates 2D line graphs, which are fundamental for showing trends in data over time. Users can customize their plots easily using various parameters to adjust properties such as line styles, colors, markers, and axis limits. This not only enhances visual appeal but also improves clarity, making it easier for the audience to derive insights from the data being presented.
Additionally, specialized functions such as bar
, scatter
, and histogram
allow for the representation of categorical data and distributions, further expanding the visualization capabilities available to users.
Moreover, MATLAB provides advanced visualization features that enable the creation of more sophisticated graphics. Functions such as surf
and mesh
are used for 3D surface plots, offering insight into data sets with multiple variables. As users progress through MATLAB tutorials, they will discover how to employ customization options such as titles, labels, and legends, which play an essential role in making plots self-explanatory and informative.
The plot3 function in MATLAB simplifies the graphical representation of three-dimensional data by allowing users to plot points, lines, and surfaces in a Cartesian coordinate system. This functionality caters to a diverse audience, ranging from beginners who are just starting to explore data visualization techniques to experienced programmers looking to enhance their graphical presentations. By enabling a spatial understanding of data, 3D plotting becomes essential in various scenarios such as engineering simulations, scientific research, and statistical analysis.
Ultimately, mastering data visualization in MATLAB not only enhances the presentation of data but also equips users with the tools to make data-driven decisions based on insightful interpretations. With practice and exploration of these functionalities, users can greatly improve their ability to convey data effectively, reinforcing the importance of visualization skills in scientific, engineering, and analytical disciplines.
3D data visualization is an essential technique for analyzing and interpreting complex datasets across various domains. Unlike traditional 2D representations, integrating a third dimension allows for a more comprehensive understanding of data interactions and relationships. By effectively visualizing data in three dimensions using MATLAB Scatter3, researchers and professionals can uncover patterns and insights that may remain hidden in conventional graphs.
Learn Working with Functions in MATLAB with Tutorials
Functions play a critical role in programming with MATLAB, enabling users to organize code effectively and promote ease of understanding and maintenance. At its core, a function is defined using the keyword function
, followed by the output variables, the function name, and the input variables. This structure aids in encapsulating code that performs a specific task, which is essential for modular programming. Creating functions allows for reusability and simplification of complex code, making it more manageable and scalable.
To define a function in MATLAB, the syntax follows a specific pattern. For example: function [output1, output2] = functionName(input1, input2)
. This sets the stage for specifying the function’s operations on the input variables and determining the values it returns. Assigning clear and descriptive names to functions enhances code readability and supports efficient collaboration when working in teams.
Input and output arguments are fundamental components of functions. Input arguments allow data to be passed to the function, while output arguments facilitate the return of results. Understanding how to manage these arguments is crucial for effective function design. Moreover, MATLAB’s ability to handle variable numbers of inputs and outputs offers flexibility, making it easier to create versatile functions tailored to particular needs.
Additionally, MATLAB comes equipped with numerous built-in functions that cater to various mathematical and engineering tasks. Utilizing these built-in functions can significantly streamline projects, as they are optimized for performance and tested for accuracy. By leveraging these resources, developers can focus on implementing unique functionalities in their projects rather than reinventing standard operations.
Incorporating functions into your MATLAB tutorials can tremendously enhance your programming capabilities. Overall, a solid understanding of how to create and utilize functions in MATLAB is essential for anyone aiming to master this versatile programming environment.
By leveraging these control flow structures, MATLAB programming can become more dynamic, enabling programmers to build more intricate and responsive applications. Through engaging with MATLAB tutorials, users can refine their understanding of these concepts and enhance their coding proficiency.
MATLAB interp1 Function
The interp1 function in MATLAB serves as a fundamental tool for conducting one-dimensional interpolation on a set of data points. Interpolation is a statistical method that estimates values between two known values, allowing for more precise data analysis and management. By employing the interp1 function, users can obtain interpolated values at specified points, thus enabling a deeper analysis of datasets.
MATLAB Ode45 function – Practical Tutorials
Ode45 is a widely utilized numerical solver designed for the effective resolution of ordinary differential equations (ODEs). It is a part of MATLAB’s ODE suite and is specifically tailored to handle problems where the solution requires the calculation of derivatives at multiple points. The significance of Ode45 stems from its implementation of the Runge-Kutta method, which offers a robust approach to approximating solutions with high accuracy.
MATLAB Diff function – Beginners Tutorials
MATLAB diff function is an essential tool utilized in the realms of data analysis and programming. Primarily, it calculates the differences between adjacent elements in an array, offering valuable insights into trends and behaviors of data sets. Through a blend of theoretical understanding and hands-on practical examples, this guide aspires to empower readers to utilize MATLAB’s diff effectively, transforming raw data into actionable insights.
MATLAB conv2 Function
Convolution is a mathematical operation that combines two functions to produce a third function, expressing how the shape of one function is modified by the other. Convolution is calculated using MATLAB conv2 function. This operation is particularly crucial in various fields such as image processing, signal analysis, and systems engineering. This function is specifically designed for two-dimensional convolution, making it preferable for tasks that involve images or other matrix-based data
fft2 in MATLAB
The FFT2 function in MATLAB is crucial for performing Fourier transforms on matrices, allowing practitioners to study and manipulate two-dimensional frequency components effectively. The Fast Fourier Transform (FFT) is an algorithm that computes the discrete Fourier transform (DFT) and its inverse efficiently. The DFT converts a sequence of equally spaced samples of a function into a sequence of coefficients of sinusoidal components, revealing the frequency spectrum of the sampled signal.
fzero MATLAB Function
The fzero in MATLAB is a powerful tool designed for finding the roots of nonlinear equations. This function plays a crucial role in various fields, including engineering, data science, and mathematical modeling. By utilizing fzero MATLAB, users can efficiently identify points where a given function equals zero, which is essential for solving problems that involve polynomial equations, differential equations, and optimization tasks.
Advanced MATLAB Techniques and Toolboxes
MATLAB has evolved into a powerful tool for various advanced applications, largely due to its extensive range of specialized toolboxes. These toolboxes are designed to address specific tasks and can be invaluable allies in enhancing the functionality and efficiency of your projects. Among the most popular are the Optimization Toolbox, Signal Processing Toolbox, and the Machine Learning Toolbox, each offering unique features that facilitate complex computations and analyses.
The Optimization Toolbox provides algorithms and tools for solving optimization problems, from linear programming to multi-objective optimization. It includes functions for finding optimal solutions in resource allocation, design configurations, and operational improvements. With this toolbox, users can refine their models and achieve superior outcomes, making it an essential asset for engineers and researchers alike.
In the realm of signal processing, the Signal Processing Toolbox is invaluable for analyzing and filtering large data sets. It encompasses a suite of algorithms and tools for Fourier analysis, time-frequency analysis, and digital filter design. These features are crucial for applications ranging from communications to biomedical engineering, where signal integrity and accuracy are paramount. By utilizing this toolbox, one can implement advanced techniques that uncover insights from complex datasets.
Machine learning has become increasingly popular in recent years, and MATLAB’s Machine Learning Toolbox makes it easy to integrate sophisticated algorithms into projects. This toolbox supports a wide range of supervised and unsupervised learning algorithms, facilitating tasks such as classification, clustering, and regression. By leveraging these advanced techniques, users can harness the full potential of their data and build predictive models that contribute to informed decision-making.
In conclusion, these advanced MATLAB tutorials on specialized toolboxes illustrate how leveraging these functionalities can significantly enhance the success and efficiency of various projects. Embracing these tools will empower learners to take full advantage of MATLAB’s capabilities and improve their analytical prowess.
Debugging and Error Handling in MATLAB
Debugging is an essential skill for any programmer, and MATLAB provides a variety of tools and techniques to assist users in this endeavor. Understanding how to properly debug MATLAB code can significantly enhance one’s coding efficiency and effectiveness. The first step in debugging is recognizing common errors, which can often be categorized as syntax errors, runtime errors, and logical errors.
Syntax errors occur when the code does not adhere to MATLAB’s grammatical rules, which can be easily identified with the help of the MATLAB editor, as it often highlights them in red. Runtime errors happen when the code is syntactically correct but fails during execution, which can lead to unexpected results or crashes.
Another critical aspect of effective debugging is interpreting error messages. MATLAB provides detailed error messages that can guide users in identifying the source of an issue. When an error occurs, MATLAB will display an error message in the command window that typically includes information such as the type of error, the line number where it occurred, and a brief description of the problem. Familiarizing oneself with these messages can significantly reduce troubleshooting time and facilitate a smoother debugging process.
In addition to these methods, MATLAB boasts several built-in tools designed for debugging. The Debugger allows users to set breakpoints, step through code, and examine variable values at different stages of execution. By using the debugging features, users can pause their code at critical junctures, enabling a granular analysis of the flow and state of their programs.
Furthermore, techniques such as print debugging—adding statements that display variable values or progress in the command window—can also be beneficial. Engaging with these debugging tactics can lead to improved coding practices, making users more adept at resolving issues in their MATLAB tutorials and fostering a more robust programming experience.
Real-World Applications of MATLAB with Tutorials
MATLAB, a high-level programming language and interactive environment, has made significant strides in numerous fields, demonstrating its versatility as an essential problem-solving tool. Its application across various sectors, including engineering, finance, healthcare, and machine learning, highlights how MATLAB tutorials equip learners with the skills necessary to tackle real-world challenges.
In engineering, MATLAB is extensively used for simulations, data analysis, and algorithm development. For instance, aerospace engineers utilize MATLAB for designing flight control systems and analyzing aerodynamic properties. A notable case is the development of the Boeing 787 Dreamliner, where teams employed MATLAB to perform complex modeling and simulations, resulting in enhanced performance and safety measures. MATLAB tutorials in engineering thus foster a profound understanding of system dynamics and linear control theory.
Similarly, in the finance sector, MATLAB serves as a powerful tool for quantitative analysis and risk management. Financial institutions leverage MATLAB for modeling asset prices and managing investment portfolios. A classic example involves the use of MATLAB in algorithmic trading, where firms implement sophisticated trading strategies that analyze market conditions in real time. Tutorials focusing on MATLAB for finance can impart learners with essential analytical skills and an understanding of financial modeling techniques.
Healthcare is another vital domain where MATLAB has found extensive application. Medical researchers often employ MATLAB for image processing in diagnostics, such as analyzing MRI and CT scans. A striking project involved using MATLAB to develop a system for detecting tumors in medical images, leading to early diagnoses and improved patient outcomes. MATLAB tutorials tailored for healthcare professionals can provide insights into biomedical signal processing and medical imaging techniques.
Lastly, in the field of machine learning, MATLAB offers a robust platform for developing algorithms and testing various models. Its capacity for handling large datasets proves invaluable for predictive analytics and deep learning applications. Successful projects, such as developing intelligent assistants and fraud detection systems, have showcased the practicality of MATLAB in machine learning endeavors. Education through focused MATLAB tutorials is particularly beneficial for those seeking to delve into this rapidly evolving area.
Tutorial on Newton Raphson Method in MATLAB
Implementing the Newton-Raphson method in MATLAB is a straightforward process and allows for the automation of iterative calculations. MATLAB’s rich libraries and intuitive interface facilitate the coding of the algorithm, providing users with the tools to explore complex functions. By leveraging MATLAB’s capabilities, one can easily handle numerical computations and visualize the convergence of the method, thus deepening the understanding of its mechanics and applications.
Learn to Implement Newton Raphson Method in MATLAB
Tutorial on Euler Method in MATLAB
One of the key advantages of the Euler method is its simplicity and ease of implementation, particularly in programming environments such as MATLAB.
Learn to Implement Euler Method in MATLAB
The method allows for a rapid computation of approximate values, making it a popular choice for introductory courses in numerical methods. the Euler method, named after the Swiss mathematician Leonhard Euler, is a fundamental numerical technique widely used in mathematical analysis for solving ordinary differential equations (ODEs).
Getting Started with MATLAB: Installation and Setup
To begin your journey into the realm of MATLAB tutorials, the first step is to successfully download and install the software on your computer. MATLAB, developed by MathWorks, is compatible with various operating systems, including Windows, MacOS, and Linux. Before starting the installation, it is crucial to ensure that your system meets the necessary requirements.
These typically include a supported version of the operating system, a minimum amount of RAM, and available disk space. As of the latest updates, MATLAB recommends at least 4GB of RAM and 2GB of free disk space for optimal performance.
The installation process begins with obtaining the MATLAB installation package. This can be done by visiting the official MathWorks website and creating an account if you do not already have one. After signing in, you can select your preferred version of MATLAB and download the installer. Once the download is complete, run the installer and follow the on-screen prompts.
This involves selecting the installation type (typical or custom), choosing the destination folder, and entering your license information. If you are a first-time user, opting for the typical installation is generally recommended, as it preselects the most essential components.
It is advisable to configure the MATLAB environment for a tailored experience right after installation. Begin by exploring the MATLAB desktop, where you will find essential tools such as the Command Window, Workspace, and Editor. Familiarizing yourself with these components can enhance your productivity in following MATLAB tutorials.
Moreover, consider customizing your preferences by going to the ‘Preferences’ menu, where you can adjust settings like the default font and color scheme. This will make your learning experience more comfortable, allowing you to focus on mastering MATLAB’s powerful features effectively.