Introduction to Enumerate in Python
The enumerate function in Python is a built-in utility that facilitates the addition of a counter to an iterable, such as a list or a tuple. This function is significant for simplifying loops when iterating over these objects and helps you do your Python assignments with ease. Without enumerate, one would typically need to manually maintain a counter variable to track the index of elements in the iterable, which can lead to less readable and more error-prone code.
When using enumerate, you automatically receive both the index and the value of each item in the iterable during each iteration. This dual output enhances code clarity by removing the need for auxiliary counter variables. For example, if one were iterating through a list of names and desired to print both the index and the name, instead of a traditional loop with manual index management, enumerate in python can streamline the operation as shown below:
for index, value in enumerate(my_list):
print(index, value)
In the above example, python for i in enumerate provides the index as i and the element directly (the name) within the same loop. This is particularly useful in scenarios where knowing the position of an element is crucial, such as when modifying lists or conditionally processing items based on their position.
Moreover, the versatility of the enumerate function extends beyond just lists. It can be applied to any iterable, making it valuable in various contexts, such as data processing and when working with sequences. The ease of use that python in enumerate provides is not merely a matter of convenience but also enhances the maintainability of your code, making it an essential tool for developers.
Looping through data is a fundamental part of Python programming. But what if you need to track the position of each item while iterating? Manually managing counters with range(len())
can be clunky and error-prone. That’s where Python’s enumerate()
function shines.
In this comprehensive guide, you’ll learn:
- What
enumerate()
is and why it’s useful - How to use
for in enumerate
loops effectively - Practical examples with lists, strings, and dictionaries
- Performance considerations and best practices
- Advanced techniques for real-world applications
By the end, you’ll be able to write cleaner, more Pythonic loops while keeping track of indices effortlessly. Let’s dive in!
What is enumerate()
in Python?
The enumerate()
function is a built-in Python utility that adds a counter to an iterable object. It returns an enumerate object which yields pairs containing the index and the corresponding item from the iterable.
Basic Syntax
enumerate(iterable, start=0)
iterable
: Any Python object capable of iteration (list, tuple, string, etc.)start
(optional): The number from which the counter starts (default is 0)
Simple Example
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits):
print(index, fruit)
Output:
0 apple
1 banana
2 cherry
This is much cleaner than the traditional approach using range(len())
:
# The old way - not recommended
for i in range(len(fruits)):
print(i, fruits[i])
Why Use enumerate() in Python?
Python’s enumerate()
offers several advantages over manual index tracking:
1. Improved Readability
The for index, value in enumerate(iterable)
pattern clearly expresses your intent to work with both the item and its position.
2. Reduced Errors
Manual index management often leads to off-by-one mistakes. enumerate()
eliminates this risk.
3. Versatility
It works with any iterable – lists, tuples, strings, dictionaries, generators, and even custom objects.
4. Pythonic Code
enumerate()
follows Python’s philosophy of simplicity and explicitness (Zen of Python).
How to Use for in enumerate
in Python
Let’s explore the most common patterns for using enumerate()
in your loops.
Basic Usage with Lists
colors = ['red', 'green', 'blue']
for position, color in enumerate(colors):
print(f"Color at position {position} is {color}")
Custom Start Index
You’re not limited to zero-based indexing:
# Start counting from 1
for rank, name in enumerate(['Alice', 'Bob', 'Charlie'], start=1):
print(f"Rank {rank}: {name}")
With Strings
enumerate()
works beautifully with strings:
word = "Python"
for idx, letter in enumerate(word):
print(f"Character {idx} is {letter}")
With Tuples
points = (10, 20, 30)
for i, point in enumerate(points):
print(f"Point {i}: {point}")
Common Use Cases for enumerate()
Now let’s look at practical scenarios where enumerate()
shines.
1. Modifying List Elements by Index
numbers = [10, 20, 30, 40]
for i, num in enumerate(numbers):
numbers[i] = num * 2
print(numbers) # [20, 40, 60, 80]
2. Creating Dictionaries from Lists
fruits = ['apple', 'banana', 'mango']
fruit_dict = {i: fruit for i, fruit in enumerate(fruits)}
print(fruit_dict) # {0: 'apple', 1: 'banana', 2: 'mango'}
3. Tracking Progress in Long Iterations
big_list = [x for x in range(10000)]
for i, item in enumerate(big_list):
if i % 1000 == 0:
print(f"Processing item {i}")
4. Data Processing and CSV Handling
import csv
with open('data.csv') as f:
reader = csv.reader(f)
for line_num, row in enumerate(reader, 1):
print(f"Line {line_num}: {row}")
Performance Considerations
Is enumerate()
faster than manual indexing? Let’s compare:
Benchmark Test
import timeit
setup = '''
data = [x for x in range(1000)]
'''
stmt1 = '''
for i in range(len(data)):
val = data[i]
'''
stmt2 = '''
for i, val in enumerate(data):
pass
'''
print("range(len()):", timeit.timeit(stmt1, setup, number=10000))
print("enumerate():", timeit.timeit(stmt2, setup, number=10000))
Results typically show enumerate()
being slightly faster (about 10-15%), but the real benefit is in code clarity and reduced errors.
Pitfalls and Best Practices os using Enumerate in Python
While enumerate()
is simple, there are some things to watch for.
1. Don’t Modify the Iterable During Iteration
# Bad practice
numbers = [1, 2, 3, 4]
for i, num in enumerate(numbers):
if num % 2 == 0:
del numbers[i] # This will cause unexpected behavior
2. Use Tuple Unpacking Clearly
# Good
for index, value in enumerate(items):
pass
# Potentially confusing
for i_v in enumerate(items):
index = i_v[0]
value = i_v[1]
3. Remember It Creates an Iterator
enumerate()
returns an iterator, so you can only consume it once:
data = ['a', 'b', 'c']
enum = enumerate(data)
list(enum) # [(0, 'a'), (1, 'b'), (2, 'c')]
list(enum) # [] (iterator is exhausted)
Advanced Tips and Tricks
Take your enumerate()
skills to the next level with these techniques.
1. Enumerating Dictionaries
person = {'name': 'Alice', 'age': 30, 'job': 'Engineer'}
for i, (key, value) in enumerate(person.items()):
print(f"{i}: {key} = {value}")
2. Combining with zip()
names = ['Alice', 'Bob', 'Charlie']
scores = [85, 92, 78]
for i, (name, score) in enumerate(zip(names, scores)):
print(f"{i}: {name} scored {score}")
3. Nested Enumerations
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
for row_idx, row in enumerate(matrix):
for col_idx, num in enumerate(row):
print(f"matrix[{row_idx}][{col_idx}] = {num}")
4. Using with Generators
def squares(n):
for i in range(n):
yield i**2
for idx, square in enumerate(squares(5), 1):
print(f"{idx}: {square}")
Video Tutorial on Enumerate in Python
In this in-depth Python tutorial, we’ll explore the versatility of the enumerate() function, a powerful tool for iterating over lists. We’ll delve into defining a list, utilizing for loops for iteration, and leveraging the enumerate function to access both the index and value within the loop. What you’ll learn:
How to define a list in Python 📋
Efficiently iterate over a list using a for loop 🔄
Utilize enumerate() to streamline your code and access both indices and values simultaneously 🔢
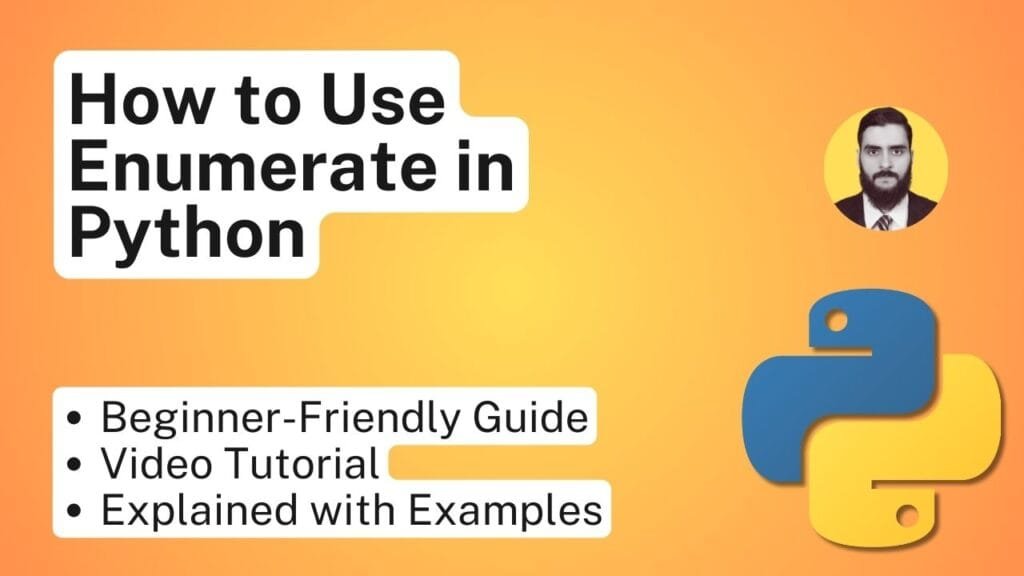
How Enumerate in Python Works: Syntax and Basics
The enumerate
function is a built-in helper function in Python that simplifies the task of iterating over iterables, such as lists and tuples. Primarily, it adds a counter to an iterable and returns it as an enumerate
object, which can subsequently be converted into a list of tuples. This makes it particularly useful in situations where both the index of each element and the element itself are required. The basic syntax of the enumerate
function is as follows:
enumerate(iterable, start=0)
In this syntax, the iterable
is the collection you want to enumerate (e.g., a list or tuple), while the start
parameter is optional and determines the starting index of the counter. By default, start
is set to 0, but this can be adjusted to any integer to begin the enumeration at that value.
For example, consider the following snippet that utilizes enumerate
in Python:
colors = ['red', 'blue', 'green']
for index, color in enumerate(colors):
print(index, color)
In this example, the for i in enumerate
loop will generate pairs of indices and corresponding colors from the list. The output will display each color along with its respective index, illustrating how enumerate in Python
can simplify loops that require knowledge of both element values and their positions. If we modify the starting index to 1, like this:
for index, color in enumerate(colors, start=1):
print(index, color)
Here, the enumeration will start at 1 instead of the default 0, demonstrating the flexibility of the enumerate
function. Overall, mastering the basics of python for i in enumerate
is an essential skill that enhances code clarity and efficiency.
Benefits of Using Enumerate in Python
The enumerate in Python function serves as a powerful tool for improving code readability and efficiency in scenarios where iteration with index tracking is required. Traditional looping methods, such as using a simple for loop, can lead to complications and increased potential for errors, particularly when managing complex data sets. By utilizing the python for i in enumerate approach, programmers can achieve cleaner and more straightforward code. This is one of the key advantages of using the enumerate function over conventional methods.
One significant benefit of python in enumerate is its capacity to streamline code by combining index and value retrieval into a single, concise line. For example, when using the enumerate function, one can iterate through a list while simultaneously accessing the index and the corresponding value. This is in contrast to traditional loop structures where separate handling of the index requires additional lines of code and calculations. Such simplifications enhance readability, making it easier for other developers to understand and maintain the code.
Moreover, the use of enumerate in Python can lead to minimized errors. In standard loop iterations, it is common to encounter off-by-one errors or mistakenly modifying the index variable, which can lead to unintended outcomes. The built-in nature of the enumerate function helps mitigate such concerns, providing a more reliable method to access elements within a loop.
From a performance perspective, the python for in enumerate syntax can also offer efficiency advantages. While typical for loops require explicit handling of both index and value assignments, the enumerate function uses built-in optimizations, making it not only a more elegant solution but potentially a more efficient one as well. Overall, leveraging the power of enumerate can significantly enhance coding practices in Python development.
Advanced Usage of Enumerate in Python: Start Index and Other Parameters
The enumerate
function in Python is a powerful tool that not only simplifies the process of iterating through iterables but also allows customization of its behavior through its parameters. Among the most notable features of enumerate
in Python is the ability to set a starting index. By default, the index begins at 0, but there are many scenarios where changing this initial value is advantageous. For instance, when processing data types that require a specific index alignment or when conducting experiments that leverage indexed results, starting from a different number can streamline coding efforts.
To use the enumerate
function with a custom starting index, you simply add a second argument. For example, if you want your loop to start counting from 1, you can do so as follows:
for i, value in enumerate(my_list, start=1):
print(i, value)
This modification is particularly useful in scenarios where users expect ‘natural’ counting, such as displaying items in a user interface. Each item will be preceded by its respective index beginning from one, enhancing user experience and clarity.
Moreover, the flexibility of the enumerate
function extends beyond just the starting index. It can also be used in more complex data structures like lists of tuples. Using for i, (a, b) in enumerate(my_tuples, start=1):
allows for unpacking elements on the fly while still maintaining access to the index.
In summary, understanding how to customize the starting index and utilizing enumerate
effectively opens the door to more efficient Python coding practices. This not only adapts to the requirements of varied programming scenarios but also aids in delivering cleaner and more readable code.
Common Mistakes When Using Enumerate
The enumerate function in Python is a powerful tool for iterating over items while keeping track of both the index and the value. However, developers, both novice and experienced, often stumble upon common pitfalls that can lead to errors in their code. One of the most frequent mistakes is related to indexing. When utilizing for i in enumerate(...)
, programmers sometimes forget that the index returned by enumerate starts from zero. This can lead to off-by-one errors, especially when translating the logic from other programming languages or when manually working with list indices.
Another common misunderstanding involves the type of object that enumerate
returns. The enumerate function produces an enumerator object that generates pairs of indices and values. New users may attempt to manipulate the result directly as a list or a tuple without converting it appropriately, which can result in unexpected behavior or errors. It is essential to recognize that while you can iterate directly over the enumerator object, converting it to a list or tuple may be necessary when you wish to access specific index-value pairs outside of the iteration context. For example, list(enumerate(my_list))
can make this clearer and more manageable.
To avoid these issues, it is advisable to familiarize oneself with the documentation regarding enumerate
in Python. Understanding the parameters and output types can drastically improve efficiency in code writing. Furthermore, carefully debugging or logging the index and values can assist in pinpointing areas where problems might arise. By being aware of these misconceptions and taking proactive steps, developers can leverage the full potential of the enumerate function without falling victim to its common pitfalls.
Practical Examples of Enumerate in Action
The enumerate function in Python serves as a valuable tool for streamlining various tasks that involve iteration. One of its primary applications is to iterate over a list of items while simultaneously maintaining the index of each item. Consider a scenario where you have a list of fruits, and you wish to print each fruit alongside its index:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits):
print(index, fruit)
In this example, python in enumerate showcases its ability to provide both the index and item in a clean and readable format. The output will be:
0 apple1 banana2 cherry
Another common use case involves more extensive datasets, such as a list of students with their scores. Using python for i in enumerate, one can easily identify and label each student’s score:
students = ['Alice', 'Bob', 'Charlie']
scores = [88, 92, 85]
for index, student in enumerate(students):
print(f'Student {index + 1}: {student} has scored {scores[index]} points.')
This code efficiently produces a report on each student’s performance, demonstrating how enumerate in python can help in managing enumeration in larger datasets while maintaining clarity.
In more advanced scenarios, python for in enumerate can assist with data manipulation, such as filtering elements based on certain conditions. For instance, if we need to filter out scores below a certain threshold, we can combine enumerate with a conditional statement:
threshold = 90
for index, score in enumerate(scores):
if score >= threshold:
print(f'Student {students[index]} has passed with a score of {score}.')
This practical implementation highlights how to use enumerate effectively in different contexts, maximizing efficiency and readability in Python code.
Performance Considerations: When to Use Enumerate
Understanding the performance implications of using the enumerate
function in Python is essential for selecting the best iteration techniques for various scenarios. The use of enumerate in python
provides a convenient method for iterating over a collection while gaining access to the index of each element. This functionality often leads to cleaner and more readable code. However, it is crucial to evaluate its efficiency relative to other methods, especially in performance-sensitive applications.
When deciding whether to use python for i in enumerate
, developers should consider the complexity of their iterations. The enumerate
function can significantly reduce the amount of boilerplate code necessary for maintaining index values, ultimately improving code clarity. For instance, instead of manually keeping track of an index variable, employing python for in enumerate
allows seamless access to both the index and the item in the loop.
However, certain performance benchmarks demonstrate that for very large datasets, using enumerate
might be marginally less efficient than alternative methods like list comprehensions or generator expressions, which can be more memory-efficient. This performance consideration is particularly relevant when high efficiency is paramount, such as in real-time processing applications. Therefore, while enumerate in python
is generally advantageous for simplicity, its use should be carefully weighed against the specific needs of the application.
Moreover, developers should also consider scenarios that can lead to limitations in utilizing the enumerate
function. For example, when dealing with nested loops or complex data structures, leveraging enumerate
could complicate the readability rather than enhance it. In such cases, explicitly managing index variables might be more appropriate.
Comparison with Other Iteration Techniques
When it comes to iterating through collections in Python, developers are often confronted with a variety of techniques, each offering distinct advantages and potential drawbacks. Among these, the use of the enumerate
function stands out when compared to traditional iteration methods, such as the range()
function alongside a conventional loop and the classic for loop.
The traditional for
loop is one of the simplest and most widely recognized ways to iterate through items in a collection. For example, when employing a for loop, developers can directly access each element within a list. However, this method lacks the ability to easily retrieve the index of each element, a limitation that can be cumbersome in scenarios where the index is necessary. This is where Python’s enumerate
function comes into play.
Using the enumerate
function, one can simultaneously retrieve both the index and the value of the items in a collection. For instance, utilizing for i, item in enumerate(collection)
provides a clear and concise method of accessing each item while retaining its corresponding index. This dual functionality not only streamlines the code but also enhances readability, making it a preferred choice in many situations.
On the other hand, employing the range()
function to iterate does allow for index access, yet can lead to additional complexity and verbosity. In a conventional setting, one may write a loop like for i in range(len(collection))
to access both the index and the value, which can often result in less elegant code compared to using enumerate
.
In conclusion, while each iteration method holds its unique strengths and weaknesses, the enumerate
function in Python remarkably simplifies common tasks where both the index and value of a collection are needed, thereby making it an essential tool for developers. Understanding when to leverage this function as opposed to traditional loops can enhance code efficiency and clarity.
Frequently Asked Questions
Q: Can you use enumerate()
with sets?
A: Technically yes, but since sets are unordered, the indices won’t correspond to any meaningful position:
s = {'a', 'b', 'c'}
for i, item in enumerate(s):
print(i, item) # Order not guaranteed
Q: Does enumerate()
work with generators?
A: Absolutely! Since enumerate()
returns an iterator itself, it pairs perfectly with generators without loading everything into memory.
Q: How to get just the index without the value?
A: Use an underscore _
as a throwaway variable:
for index, _ in enumerate(items):
print(f"Processing index {index}")
Q: What’s the difference between enumerate() and zip(range(len()), items)?
A: Functionally similar, but enumerate() is more readable and slightly more efficient.
Conclusion: The Power of Enumerate in Python
In this exploration of Python’s enumerate function, we have highlighted its significance and utility in streamlining the process of iterating over lists or other iterable objects while maintaining an index. The enumerate in Python allows developers to write cleaner, more efficient code by eliminating the need for manual index tracking. Instead of using a traditional for loop coupled with an index variable, you can leverage python for i in enumerate, thereby enhancing readability and reducing the potential for errors. This functionality is essential for managing collections of items where knowing the index is crucial.
We also discussed how python for in enumerate can be used effectively in various scenarios, such as when you need both the item and its position in an output. By adopting the enumerate function, developers can focus on core logic rather than boilerplate code, leading to increased productivity and maintainable projects. A key takeaway is the versatility of enumerate, which not only simplifies the loop structure but also enhances overall performance when dealing with large datasets.
Python’s enumerate()
function is a powerful tool that makes your loops cleaner, more readable, and less prone to errors. By using for index, value in enumerate(iterable)
, you can:
- Easily track positions while iterating
- Write more Pythonic and maintainable code
- Handle various data structures consistently
- Improve your code’s performance slightly
Want more Python tips? Check out our other tutorials on list comprehensions, generator expressions, and the zip()
function.
For those eager to deepen their Python proficiency further, we recommend exploring additional resources on iterating in Python. Consider checking out tutorials on list comprehensions, functional programming with Python’s map and filter functions, and the principles of generator expressions. These topics can complement your understanding of functions and the nuances of Python’s iteration capabilities. As you continue your journey with Python, mastering the enumerate function will undoubtedly be a valuable skill, empowering you to write efficient and elegant code.