Introduction to For Loops in MATLAB
For loops are a fundamental programming construct in MATLAB, designed to execute a block of code repeatedly for a specified number of iterations. They are integral to programming, especially in scenarios where a task needs to be performed multiple times with varying values. The purpose of using a for loop in MATLAB is to automate repetitive tasks, enhance code efficiency, and allow for scalable solutions, making it a valuable tool for developers and engineers alike.
The basic syntax of a for loop in MATLAB is straightforward. It begins with the keyword “for” followed by a loop variable that iterates through a specified range. The structure is as follows:
for index = startValue:endValue% Code to be executedend
In this syntax, “index” serves as an integer variable taking values from “startValue” to “endValue.” This mechanism significantly simplifies coding, as it eliminates the need for manual repetition of similar commands. For instance, if we want to calculate the squares of the first five integers, the implementation would look like this:
for i = 1:5disp(i^2);end
This example demonstrates how the for loop iterates over the values 1 to 5, calculating and displaying each square in succession. The simplicity and clarity provided by for loops make them particularly effective in processing arrays or performing computations that require repetitive processes such as simulations and iterative algorithms.
For loops in MATLAB shine particularly in data analysis tasks, where they can process large datasets efficiently. When faced with operations that require iterative adjustments, implementing a for loop can significantly streamline coding practices, allowing developers to focus on problem-solving rather than syntax mechanics. As we move forward in this guide, we will explore more advanced applications of for loops in MATLAB, improving functionality and usability for various programming challenges.
Watch MATLAB tutorials – For MATLAB Loop
In this in-depth tutorial, we explore the core concepts of loops like while and for in MATLAB from the mathworks, with practical examples for every beginner to expert level. Whether you’re looking to understand the structure of for MATLAB loops, while loops, or how to leverage the break and continue statements to control flow, this guide covers it all! What you’ll learn:
- For Loop Example: Learn how to use the for MATLAB loop to repeat a block of code a specific number of times.
- While Loop Example: Master the while loop for executing code as long as a condition is true.
- Break Statement: See how to exit loops early with the break statement when certain conditions are met.
- Continue Statement: Learn how to skip over parts of your loop with the continue statement.
- Nested Loops: Understand how to work with nested loops for more complex operations and data processing.
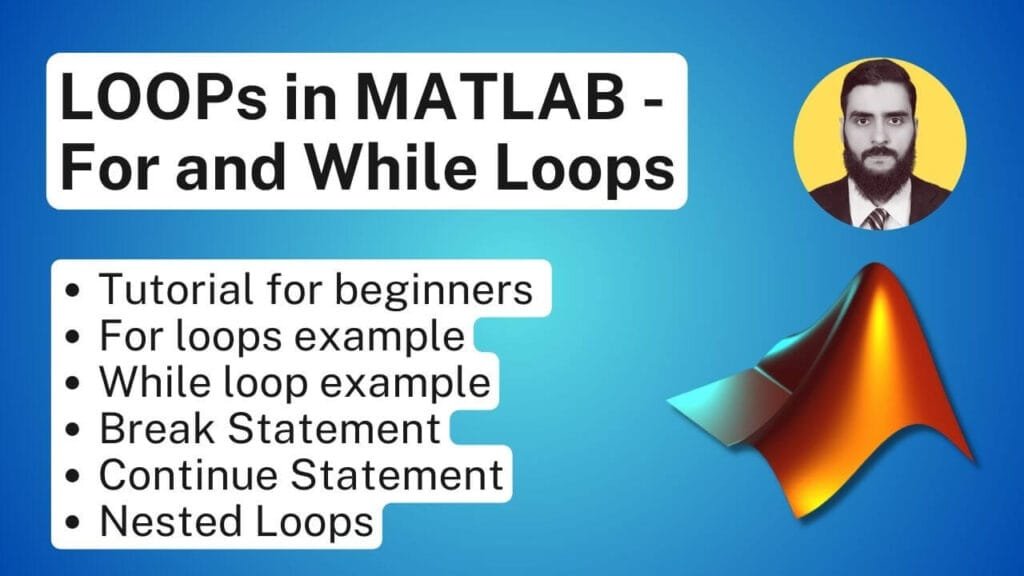
Constructing and Using For MATLAB Loop
In MATLAB, the for loop is an essential construct that facilitates repetitive operations on data sets. The basic structure of a for loop consists of initialization, condition-checking, and iteration, allowing users to efficiently execute tasks involving arrays and complex calculations. To construct a for loop, one begins by specifying an iteration variable, often referred to as the loop variable. This variable is initialized at a specific numeric value, which determines the starting point of the loop.
The syntax for a simple for loop can be expressed as follows:
for index = startValue:endValue% Code to executeend
Here, ‘index’ serves as the loop variable, while ‘startValue’ and ‘endValue’ define the loop’s range. During each cycle of the loop, MATLAB evaluates the specified code block, updating the loop variable until the end value is reached. This can be particularly useful for iterating through arrays and performing repetitive calculations, ensuring that complex tasks are executed systematically.
For instance, consider a scenario where you need to calculate the square of the first ten positive integers. By employing a for loop, this can be accomplished succinctly:
for i = 1:10squares(i) = i^2;end
However, it is crucial to be mindful of common pitfalls such as infinite loops or off-by-one errors, which can arise from improper initialization or condition-setting. To avoid these issues, it is advisable to thoroughly test the loop conditions and ensure that the iteration correctly progresses towards the termination condition.
Best practices when writing MATLAB for loops include keeping the code within the loop simple and efficient, as well as recognizing when vectorized operations may offer better performance. By adopting these strategies, users can master the art of constructing and utilizing for loops in MATLAB effectively.
Applications of For Loops in MATLAB
For loops in MATLAB programming serve as a fundamental construct that facilitates iteration, making them essential in various applications across multiple domains. By allowing users to execute a block of statements repeatedly, for loops significantly streamline processes such as data analysis, simulation, numerical methods, and graphical programming.
In data analysis, for loops enable the efficient processing of large datasets. For instance, when calculating the mean of a series of values or iterating through a dataset to filter or aggregate data points, a MATLAB for loop can be instrumental. For example, if one needs to analyze multiple variables from a dataset, one can use a for loop to iterate over each variable systematically, applying necessary transformations or calculations, thereby enhancing the data processing experience.
In simulation tasks, MATLAB’s for loop can effectively model complex systems over discrete time intervals. For instance, in a physics simulation, a for loop can iterate through a series of time steps to compute positions or velocities of particles, allowing users to observe and analyze dynamic behavior over time. This application demonstrates how for loops can handle complex computational tasks, producing outputs that visualize system behavior under various conditions.
Additionally, for loops are widely utilized in numerical methods. Algorithms such as those for solving differential equations often involve iterative computations. A for loop can drive the numerical process, allowing MATLAB to apply methods like Euler’s method or the Runge-Kutta method across a series of points or steps efficiently.
Furthermore, in graphical programming, a MATLAB for loop can aid in creating visual representations of data. For example, multiple plots can be generated in succession by iterating through datasets with a for loop, enhancing data visualization capabilities and providing insightful comparative analyses.
Advanced Techniques and Optimization with For MATLAB Loop
In MATLAB, leveraging the for loop effectively can significantly enhance performance, especially when dealing with large datasets. However, it’s essential to understand specific optimization techniques to make your code run more efficiently. One common method to optimize loops is to minimize the amount of computation performed within the loop body. Instead of repeatedly calculating the same expression inside the loop, compute it once before the loop starts and store the result in a variable.
Another advanced technique is vectorization, which involves rewriting for loops as vectorized operations. MATLAB is designed to work exceptionally well with matrices and arrays, allowing users to perform operations on entire data sets without explicitly using loops. By utilizing vectorized commands, you can achieve significant performance improvements and write cleaner, more concise code. For example, instead of iterating over an array with for loops, try using built-in functions like sum()
, mean()
, or logical indexing to perform operations.
Performance considerations also play a critical role when utilizing for loops in MATLAB. When executing a loop, the speed of execution can be affected by the operations performed within. It is advisable to preallocate arrays that will store results rather than allowing MATLAB to dynamically resize them during loop execution. This practice can greatly reduce memory allocation overhead, thus increasing execution speed.
In addition to these techniques, MATLAB offers several built-in functions that can often serve as alternatives to for loops. Functions such as arrayfun()
and parfor()
for parallel execution can help streamline tasks that might otherwise require lengthy for loops. Embracing these methodologies not only enhances performance but also promotes better coding practices, making your MATLAB code more efficient and maintainable.
Need Help in Programming?
I provide freelance expertise in data analysis, machine learning, deep learning, LLMs, regression models, NLP, and numerical methods using Python, R Studio, MATLAB, SQL, Tableau, or Power BI. Feel free to contact me for collaboration or assistance!
Follow on Social
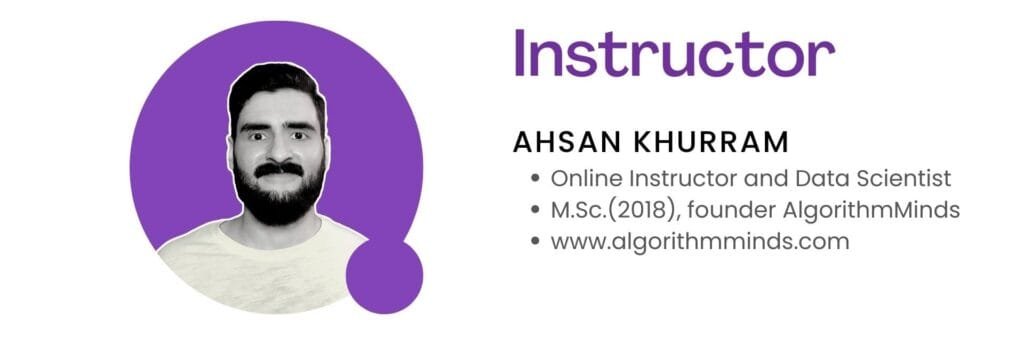