Introduction to Python Data Types
In Python, data types serve as the fundamental building blocks for any programming endeavor. They define the nature and characteristics of data, allowing programmers to manipulate this data effectively. Data types comprise specific attributes that dictate how the values are stored in memory and how they can be processed by the application. Understanding these data types is crucial for effective coding or doing Python Assignments, as they shape how variables behave and interact within a program.
Python provides a variety of built-in data types, which can broadly be categorized into mutable and immutable categories. Mutable data types, such as lists and dictionaries, can be altered after their creation, making them ideal for scenarios where data may need to be changed frequently. In contrast, immutable data types, including tuples and strings, cannot be modified once defined, ensuring their integrity throughout the program’s execution. This distinction between types is crucial for memory management and performance optimization.
When declaring variables in Python, the choice of data type inherently influences the operations that can be performed on them. For instance, numerical data types, such as integers and floats, allow arithmetic operations, while string data types enable text manipulation. As Python is dynamically typed, variable data types are assigned automatically at runtime based on the assigned value. However, understanding the underlying data type is vital for effective programming, particularly in debugging and algorithm optimization.
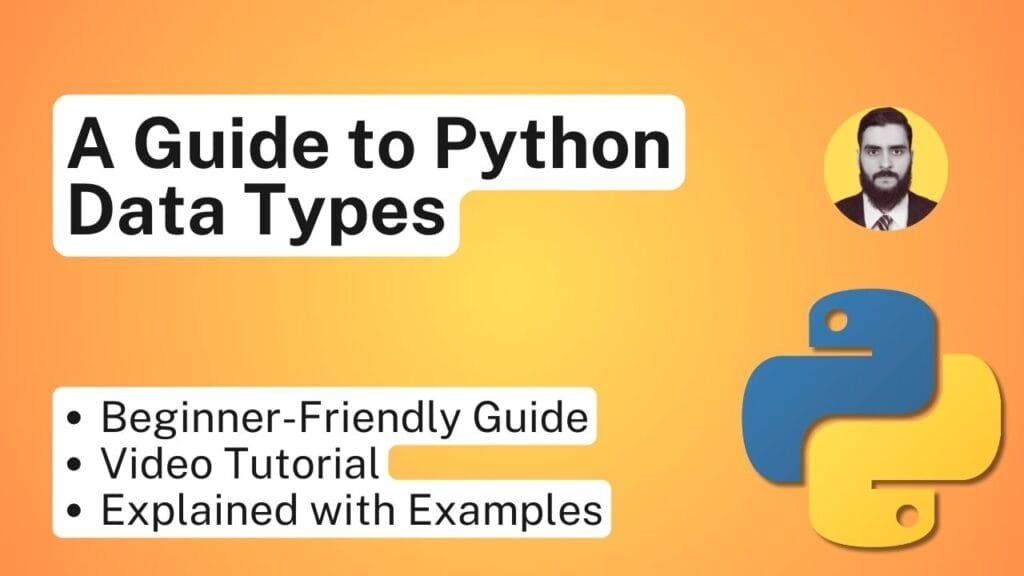
As programming evolves, the significance of grasping data types becomes increasingly apparent. Mastery of these concepts not only facilitates writing more efficient and reliable code but also enhances collaboration among developers by establishing a common understanding of how data interacts within the Python environment.
Numeric Data Types in Python
In Python, numeric data types play a fundamental role in representing various kinds of numerical values. The three primary numeric data types available are integers, floats, and complex numbers. Each type serves a distinct purpose and is utilized in different contexts depending on the requirements of the application.
Integers, commonly referred to as “ints,” represent whole numbers without any fractional or decimal components. They can be positive or negative, and Python allows for the use of arbitrarily large integers. For example, declaring a variable as x = 10
assigns the integer 10 to x. Integers are typically used for counting or indexing, where precise whole number values are needed.
On the other hand, floating-point numbers, or “floats,” are used to represent real numbers that require decimal points. A float can be defined as y = 10.5
, where y holds a decimal value. This type is essential for scenarios requiring precision, such as scientific calculations or financial computations where fractions are common.
Complex numbers are another numeric data type that combines both real and imaginary parts. In Python, a complex number is defined as z = 3 + 4j
, where 3 is the real part and 4j denotes the imaginary part. Complex numbers are particularly significant in advanced mathematics, engineering, and certain computational applications.
It is also important to understand type conversion among these numeric types. Python provides built-in functions such as int()
, float()
, and complex()
to facilitate easy conversions between integers, floats, and complex numbers. For instance, if you want to convert a float to an integer, you can use int(10.5)
, which would yield 10. Properly utilizing numeric data types ensures the effective handling of mathematical operations in Python, enhancing overall program robustness.
String Data Type
The string data type in Python is a versatile and essential element for representing textual data. It is defined as a sequence of Unicode characters and can encompass letters, digits, symbols, and whitespace characters. Strings can be created by enclosing text in either single quotes, double quotes, or triple quotes for multi-line strings. For example:
single_quote_string = 'Hello, World!'
double_quote_string = "Python Programming"
triple_quote_string = '''This is a multi-line string.'''
Once a string is created, various operations can be performed to manipulate it. String concatenation enables users to join different strings together using the +
operator, while repetition can be achieved using the *
operator. For instance:
greeting = "Hello" + " " + "World!"
repeated_string = "Ha" * 3 # Outputs "HaHaHa"
Python also provides a rich set of methods for string manipulation. Commonly used methods include lower()
for converting strings to lowercase, upper()
for uppercase conversion, split()
for breaking the string into a list, and replace()
for substituting parts of a string. For example:
text = "Python is great"
text_list = text.split() # Outputs ['Python', 'is', 'great']
Another crucial aspect of strings in Python is their immutability. Once a string object is created, it cannot be altered. This attribute ensures integrity and security, particularly in large applications where inadvertent changes to string data could lead to bugs. If modifications need to be made, a new string must be generated, reflecting the desired changes. For instance:
original = "Python"
modified = original.replace("P", "J") # Outputs "Jython"
In practical applications, Python strings are integral for handling user input, generating output for applications, manipulating text data, and processing files, making them indispensable in programming.
Boolean Data Type
The boolean data type is a fundamental element in Python, representing one of the simplest data structures within the programming language. Specifically, it has two possible values: True and False. These values are integral to various programming constructs, especially in control flow statements. In Python, the boolean type is not merely a class; it also plays a crucial role in taking decisions in programs.
In control flow statements, such as if
, elif
, and else
, boolean expressions evaluate conditions to determine the path the program will take. For instance, consider the following code snippet:
temperature = 30if temperature > 25: print("It's a warm day.")else: print("It's a cool day.")
Here, the expression temperature > 25
evaluates to a boolean value. If the condition holds true (i.e., temperature is indeed greater than 25), the code block under if
executes, illustrating how boolean data types influence the flow of the program.
Furthermore, boolean values are crucial for comparison operations. In Python, various comparison operators return boolean results, such as ==
(equal to), !=
(not equal to), >
(greater than), and so on. These operators allow programmers to conduct checks on data types to ensure they operate correctly. For example:
a = 10b = 20result = a < b # Evaluates to True
This boolean result can be used in conditional statements to guide the flow of the script. Additionally, logical operations such as and
, or
, and not
enable complex condition evaluations, increasing the expressiveness of boolean logic in Python programming. The ability to manipulate and use boolean data optimally is essential for efficient coding practices.
List Data Type
In Python, the list data type is one of the most versatile and commonly used data structures, designed to hold an ordered collection of items. Lists can contain elements of different data types, such as integers, strings, and even other lists, making them inherently flexible. This characteristic differentiates lists from some other data types, such as tuples, which require all elements to be of the same type. Python lists are defined by enclosing elements within square brackets, with items separated by commas.
A key feature of lists in Python is their mutability. Unlike strings and tuples, which are immutable, lists can be modified after their creation. This means that users can freely add, remove, or change elements in a list, allowing for dynamic adjustments based on the program’s needs. Operations such as appending new elements with the append()
method or removing elements via the remove()
method exemplify the flexibility that Python lists offer to developers.
Accessing elements in a list is straightforward; Python uses zero-based indexing for this purpose. For example, to access the first element of a list, one would use the syntax list_name[0]
. Additionally, Python allows for slicing, which enables users to retrieve a subsequence of a list with minimal effort. Slicing can be implemented via the colon operator, such as list_name[start: end]
, to obtain a subset of the list.
Lists find extensive applications in various domains, including data handling, algorithms, and even user interface design. For instance, they can be utilized to store multiple user inputs in an interactive application or to manage datasets for machine learning tasks. With their distinctive characteristics and ease of use, the list data type remains an essential component of Python programming.
Tuple Data Type
In Python, a tuple is a built-in data type that is similar to a list but possesses a significant characteristic: tuples are immutable. This means that once a tuple is created, its contents cannot be altered, making it a stable and secure choice for certain applications. The immutability of tuples allows them to be utilized as keys in dictionaries, which is not possible with lists. This fundamental difference between these two data structures is vital for understanding when to use each one.
Creating a tuple in Python is straightforward. A tuple is defined by enclosing a sequence of elements in parentheses, separating each element with a comma. For example, my_tuple = (1, 2, 3)
creates a tuple containing three integers. An important feature of tuples is their ability to hold mixed data types, which allows for diverse and flexible data storage. For example, mixed_tuple = (1, "example", 3.14)
contains an integer, a string, and a float.
Tuple unpacking is another notable characteristic. This feature enables simultaneous assignment of tuple values to multiple variables. For instance, if coordinates = (10, 20)
is defined, one can unpack the values using x, y = coordinates
. After executing this, x
will equal 10 and y
will equal 20, allowing for clean and efficient code.
Tuples find their use in various scenarios. They are particularly advantageous when you want to maintain a fixed collection of items, such as the days of the week or geographical coordinates. When performance is a consideration, tuples can be more efficient than lists, especially when handling large data sets, as their immutable nature can lead to faster operations in certain contexts.
Need Help in Programming?
I provide freelance expertise in data analysis, machine learning, deep learning, LLMs, regression models, NLP, and numerical methods using Python, R Studio, MATLAB, SQL, Tableau, or Power BI. Feel free to contact me for collaboration or assistance!
Follow on Social
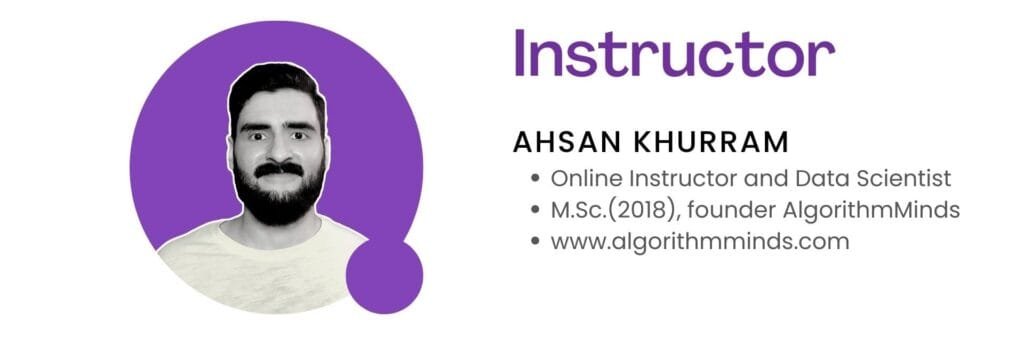
Set Data Type
In Python, the set data type is a built-in data structure designed to store unordered collections of unique items. This characteristic of uniqueness means that no duplicate elements can exist within a set. The unordered nature of sets ensures that the elements do not retain any specific positioning, making sets inherently different from lists and tuples, which maintain order. These properties make sets particularly useful for various programming scenarios, including membership testing and eliminating duplicate entries in data.
Set operations are fundamental to understanding how to manipulate collections of unique items efficiently. Python provides a range of operations that can be performed on sets, including union, intersection, and difference. The union operation combines two sets, returning a new set containing all unique elements from both sets. In Python, the union can be executed using either the `union()` method or the `|` operator. By contrast, the intersection operation retrieves only the elements that are present in both sets, which can be accomplished through the `intersection()` method or the `&` operator. The difference operation allows for the identification of elements present in one set but absent in another, further enhancing the analytic capabilities of set data types.
Practical examples of set usage can be found in numerous scenarios. For instance, when managing a unique collection of user IDs, such as verifying registered users in a system, sets enable efficient membership checks and prevent duplication of IDs. Another application might involve analyzing data sets for overlap, such as determining the common items purchased by multiple customers. In these instances, the set data type not only simplifies the code but also optimizes performance by reducing time complexity during collection operations.
Dictionary Data Type
In Python, the dictionary data type is a built-in data structure that allows storage and management of data in key-value pairs. This versatile type is ideal for situations where it is essential to associate specific keys with their corresponding values, facilitating efficient data retrieval and manipulation. Dictionaries are particularly effective when managing large data sets, as their inherent structure enables quick access to information.
To create a dictionary in Python, one can use curly braces or the dict() constructor. A simple example would include creating a dictionary of fruit names with their respective quantities:
fruits = {'apple': 10, 'banana': 5, 'orange': 8}
This example illustrates that “apple,” “banana,” and “orange” are the keys, while their respective quantities (10, 5, and 8) are the values. Accessing a value in a dictionary is done through its key. For instance, to retrieve the quantity of bananas, one would employ:
print(fruits['banana']) # Output: 5
Manipulating dictionaries is straightforward. You can add a new item, update an existing one, or delete a key-value pair. To add an item, simply assign a new value to a new key:
fruits['grape'] = 12
For updating, you can assign a new value to an existing key:
fruits['apple'] = 15
To remove a key-value pair, the del keyword can be utilized:
del fruits['orange']
Dictionaries are widely applicable in different programming scenarios, such as storing configuration settings for applications or organizing various types of data for easy access. Due to their ability to store complex data structures, including lists and other dictionaries as values, they can accommodate diverse use cases in data science and database management, making them an essential component of the Python data types.
Learn Python with Free Online Tutorials
This guide offers a thorough introduction to Python, presenting a comprehensive guide tailored for beginners who are eager to embark on their journey of learning Python from the ground up.
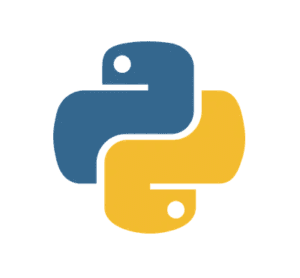
User-Defined Data Types
In Python, user-defined data types play a pivotal role in the realm of object-oriented programming. Developers can create custom data types using classes, which serve as blueprints for creating objects. These classes encapsulate both data and behaviors associated with that data, offering a way to model real-world entities more effectively. By defining attributes and methods within a class, programmers are able to tailor data types according to their specific needs, thus enhancing flexibility and organization in their code.
Encapsulation is a key principle associated with user-defined data types. It refers to the bundling of data and methods that operate on that data within one unit—essentially, a class. This allows for a controlled interface where the internal workings of the data type are hidden from the outside world. Such separation supports data integrity and security, ensuring that users interact with an object through defined interfaces rather than directly manipulating the data. This encapsulation is particularly important for maintaining clean and maintainable code structures.
When defining a user-defined data type, one starts by creating a class using the class
keyword. For example, consider a simple class representing a `Car`. This class may have attributes such as `color` and `model`, and methods like `drive()` and `stop()`. After defining the class, one can create instances, or objects, of that class, each encapsulating their own states while sharing the behavior defined in the class. By employing user-defined data types, programmers can implement complex functionalities while adhering to the principles of modularity and reusability in their code.
To illustrate, if a developer needs to keep track of multiple car objects, they could instantiate the `Car` class multiple times, each with different attributes, offering a practical approach to manage multiple data records simultaneously. Ultimately, user-defined data types in Python empower developers by allowing them to build robust applications that leverage the full spectrum of object-oriented design principles.
Video Tutorial on Python Data Types
Discover the building blocks of Python programming! In this video, we delve into data types in python, from numeric values to text strings, and explore essential structures like lists, tuples, dictionaries, and sets. 🐍🔍
🔑 Key Takeaways:
- Understand how Python handles integers, floats, and booleans.
- Dive into the versatility of text strings and their manipulation.
- Explore the magic of lists, tuples, dictionaries, and sets.
- Learn about seamless data conversion techniques.
🔍 What You’ll Learn:
- Numeric data types: int, float, complex
- Text data types: str (strings)
- Boolean data types: True and False
- Lists: Creating, accessing, and modifying list elements
- Tuples: Immutable sequences
- Dictionaries: Key-value pairs
- Sets: Unordered collections of unique elements
- Data conversion techniques in Python
Whether you’re a beginner or an experienced coder, this video will empower you with the knowledge you need to harness Python’s data types effectively. 🚀
Explanation
Introduction to Data Types
Data types in Python represent the kind of values that variables can hold. Here are some common data types:
Numeric Types:
- Integers (int): Whole numbers without decimal points.
- Floating-Point Numbers (float): Real numbers with decimal points.
Complex Numbers (complex): Numbers with a real and imaginary part.
Text Type:
- Strings (str): Sequences of characters enclosed in single or double quotes.
Boolean Type:
- Boolean (bool): Represents either True or False.
Data Structures
Python provides several built-in data structures to organize and manipulate data:
- Lists: Ordered collections of items (mutable).
- Tuples: Ordered collections of items (immutable).
- Sets: Unordered collections of unique items.
- Dictionaries: Key-value pairs for efficient data retrieval. Dictionaries are unordered, mutable, and iterable, making them efficient for accessing and manipulating data based on keys.
Mutable Data Types:
Allow their contents to be modified after creation. This means you can add, remove, or modify elements without changing the identity of the object.
Immutable Data Types:
Do not allow their contents to be changed after creation. Once an immutable object is created, its value cannot be modified.
Type Conversion
You can convert between different data types using type casting functions like int(), float(), str(), etc.
Python Code
Numeric Data Type
# Integers
my_integer = 42
print(type(my_integer)) # Output: <class 'int'>
# Floating-Point Numbers
my_float = 3.14
print(type(my_float)) # Output: <class 'float'>
# Complex Numbers
my_complex = 2 + 3j
print(type(my_complex)) # Output: <class 'complex'>
String Data Type
text_str = "Hello, world!"
formatted_str = "My name is {} and I am {} years old".format("John", 30)
print(type(text_str))
Boolean
bool_example = True
print(type(bool_example))
Lists , Tuple, Sets, Dictionaries
my_list = [1, 2, 3, 4]
print(type(my_list))
my_tuple = (1, 2, 3, 4, 5)
print(type(my_tuple))
set_example = {1, 2, 3, 4, 5}
print(type(set_example))
my_dict = {"name": "Alice", "age": 30}
print(type(my_dict))
Data Type Conversion
# Convert float to integer
my_float = 3.14
my_integer = int(my_float)
print(type(my_integer))
# Convert integer to float
my_integer = 42
my_float = float(my_integer)
print(type(my_float))
# Convert string to integer
my_string = "123"
my_integer = int(my_string)
print(type(my_string))