Introduction to For Loops in MATLAB
In the realm of programming and data analysis, the concept of loops plays a crucial role, and one of the most fundamental types is the “for loop.” For loops in MATLAB are a powerful control structure that allows for the automation of repetitive tasks by executing a block of code a specific number of times. This functionality is invaluable in programming, particularly for students who are learning MATLAB, as it enhances efficiency and facilitates complex calculations.
The syntax for a for loop in MATLAB is straightforward and consists of the keywords `for`, a loop variable, a range of values, and the `end` keyword to signify the conclusion of the loop. A basic structure looks like this: for index = startValue:endValue
, where “index” is the loop variable that takes on each value from “startValue” to “endValue” during the iterations. The block of code indented under the for loop executes for each value of the index, thus allowing for systematic iteration over a defined range.
For instance, consider a simple scenario where a student wants to calculate the squares of the first five integers. Using a for loop, this can be efficiently achieved as follows:
for i = 1:5square = i^2;disp(square);end
In this example, the variable `i` iterates from 1 to 5, allowing the program to compute and display the square of each integer sequentially. The use of for loops not only streamlines coding efforts but also significantly reduces the potential for human error in repetitive calculations. This feature is particularly beneficial for students encountering large datasets or complex algorithms in MATLAB, empowering them to approach data analysis with confidence and clarity.
Creating For Loops in MATLAB
In MATLAB, the for
loop is a fundamental control structure that allows you to repeat a block of code a specific number of times. When creating a for
loop, it is essential to adhere to its basic structure, which comprises three main components: initialization, condition, and increment expressions. Understanding these components will help students write effective loops tailored to their specific needs.
The initialization occurs at the start of the loop and sets the loop variable to its initial value. For example, in the syntax for i = 1:10
, the loop variable i
is initialized to 1. The condition, specified by the range 1:10
, defines the endpoints for the loop; in this case, the loop will run as long as i
is less than or equal to 10. After each iteration, the increment expression modifies the loop variable, which by default increases by one unless otherwise specified.
To illustrate this, consider a simple example: calculating the sum of the first ten positive integers. By using a for
loop, students can initialize a variable for the sum and then iterate through the integers, adding each to the sum during every loop iteration. Below is a sample code snippet:
sum = 0;for i = 1:10sum = sum + i;enddisp(sum);% Output: 55
Furthermore, for
loops can be effectively utilized to process arrays or datasets. For instance, if you are working with a vector of data, you can loop through each element to perform calculations, transformations, or statistical analyses. The flexibility and power of for
loops make them an indispensable tool for students in MATLAB as they work towards mastering programming and data analysis.
Watch MATLAB tutorials – For MATLAB Loop
In this in-depth tutorial, we explore the core concepts of loops like while and for in MATLAB from the mathworks, with practical examples for every beginner to expert level. Whether you’re looking to understand the structure of for MATLAB loops, while loops, or how to leverage the break and continue statements to control flow, this guide covers it all! What you’ll learn:
- For Loop Example: Learn how to use the for MATLAB loop to repeat a block of code a specific number of times.
- While Loop Example: Master the while loop for executing code as long as a condition is true.
- Break Statement: See how to exit loops early with the break statement when certain conditions are met.
- Continue Statement: Learn how to skip over parts of your loop with the continue statement.
- Nested Loops: Understand how to work with nested loops for more complex operations and data processing.
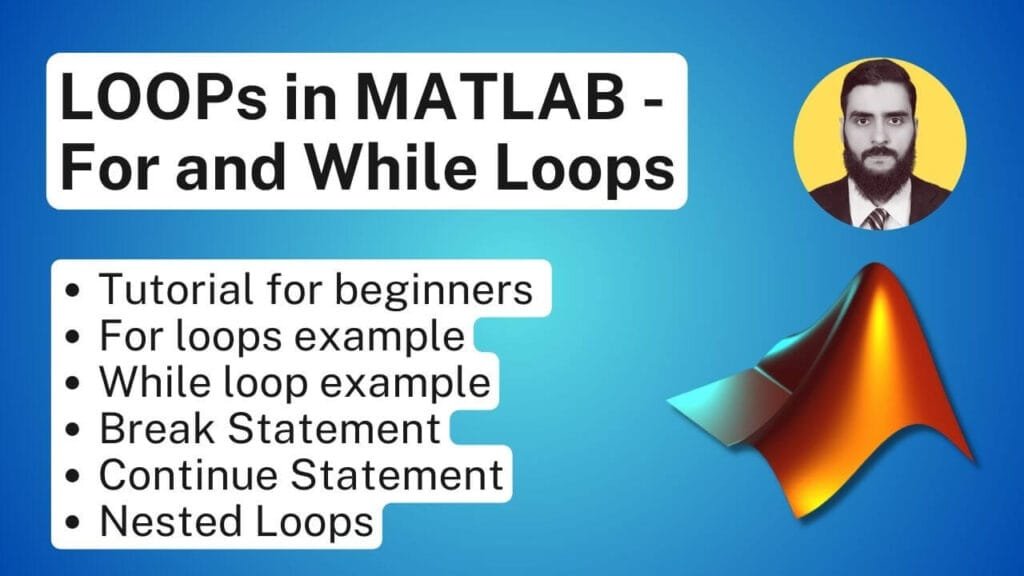
System Requirements for Using MATLAB
To effectively utilize MATLAB, particularly for tasks involving for loops, it is essential to ensure that your computer meets certain system requirements. These specifications encompass hardware capabilities, operating system compatibility, and necessary software dependencies that collectively enhance the performance of MATLAB programming.
Firstly, the hardware specifications play a pivotal role in executing MATLAB efficiently. A recommended starting point includes a modern multi-core processor, such as Intel Core i5 or an equivalent AMD processor, which can manage multiple tasks simultaneously. Additionally, having at least 8 GB of RAM is advisable to allow for smooth execution of complex calculations and data manipulations, especially when using for loops which can be computationally intensive. For optimal performance, having more than 16 GB of RAM is preferred, particularly when working with larger datasets or applications that require significant computational power.
When it comes to operating systems, MATLAB is compatible with various platforms, including Windows, macOS, and Linux. It is crucial to use the latest versions of these operating systems, as compatibility issues may arise with older versions, impacting MATLAB’s functionality. Students should verify the specifications listed on the official MATLAB website for the most current system requirements relevant to their operating system.
Moreover, software dependencies such as .NET Framework (on Windows) or Xcode (on macOS) may be required for full functionality. Ensuring these dependencies are installed can prevent execution errors when running MATLAB. Students can check if their computer is suitable for MATLAB by visiting the MATLAB documentation and using the system check utilities provided as part of the installation process. This guidance empowers students to prepare their systems effectively for programming in the MATLAB environment.
Control Statements in For Loops
In MATLAB, for loops are a fundamental construct that allows for the execution of a block of code repeatedly for a specified number of iterations. Within these loops, control statements such as ‘break’ and ‘continue’ serve as powerful tools for altering the natural flow of execution, thus giving programmers refined control over the iterative processes.
The ‘break’ statement is used to exit a for loop prematurely. When a certain condition is met, the ‘break’ command terminates the loop immediately, and control is transferred to the next line of code following the loop. This is particularly useful in situations where continuing the iterations is not necessary or efficient—for example, when searching for a specific value in an array. Consider the following illustrative example:
for i = 1:length(array)if array(i) == target_valuedisp('Value found!');break; % Exit the loop once the target is foundendend
In this example, the loop will only iterate as long as the condition is not met. Once the target value is located, the ‘break’ statement ensures that unnecessary iterations are avoided, thereby enhancing efficiency.
The ‘continue’ statement, on the other hand, is employed to skip the remainder of the current iteration and move directly to the next iteration of the loop. This is particularly advantageous when certain conditions render additional code within the loop unnecessary for specific iterations. For example:
for j = 1:10if mod(j, 2) == 0continue; % Skip even numbersenddisp(j); % This will only display odd numbersend
In this case, the loop iterates through numbers 1 to 10, but the ‘continue’ statement excludes even numbers from any further processing, leading to a cleaner and more efficient output. Utilizing these control statements adeptly can significantly improve the readability and effectiveness of MATLAB programs.
Need Help in Programming?
I provide freelance expertise in data analysis, machine learning, deep learning, LLMs, regression models, NLP, and numerical methods using Python, R Studio, MATLAB, SQL, Tableau, or Power BI. Feel free to contact me for collaboration or assistance!
Follow on Social
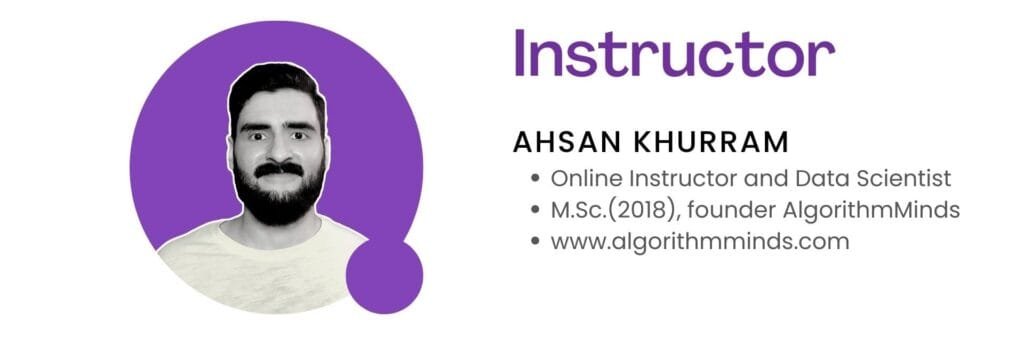