fzero Function in MATLAB
The fzero in MATLAB is a powerful tool designed for finding the roots of nonlinear equations. This function plays a crucial role in various fields, including engineering, data science, and mathematical modeling. By utilizing fzero MATLAB, users can efficiently identify points where a given function equals zero, which is essential for solving problems that involve polynomial equations, differential equations solutions such as diff and ode45 functions in MATLAB.
One of the primary purposes of ‘fzero’ is to provide numerical solutions to equations that may not be solvable through analytical methods. For instance, in data science, it is common to encounter nonlinear models where roots determine critical thresholds or performance metrics. ‘Fzero’ allows researchers and programmers to systematically find these roots with a high level of precision. It uses iterative methods to navigate the complexities of nonlinear functions, making it an efficient choice for many computational scenarios.
The importance of ‘fzero’ extends beyond its primary function as a root-finding algorithm. It serves as an integral part of many algorithms in data analysis and machine learning, where model fitting often requires the identification of solution points. Furthermore, users can apply ‘fzero’ to optimize functions, determine equilibrium points in system dynamics, or fine-tune parameters based on empirical data. The versatility of this function positions it as a staple in the toolkit of data scientists and programmers alike.
Exploring the ‘fzero’ function in detail reveals its numerous options and capabilities, setting the stage for effective application in varied contexts. In the following sections, we will delve deeper into how to leverage ‘fzero’ for practical problem-solving, ensuring that users can maximize its utility in their projects.
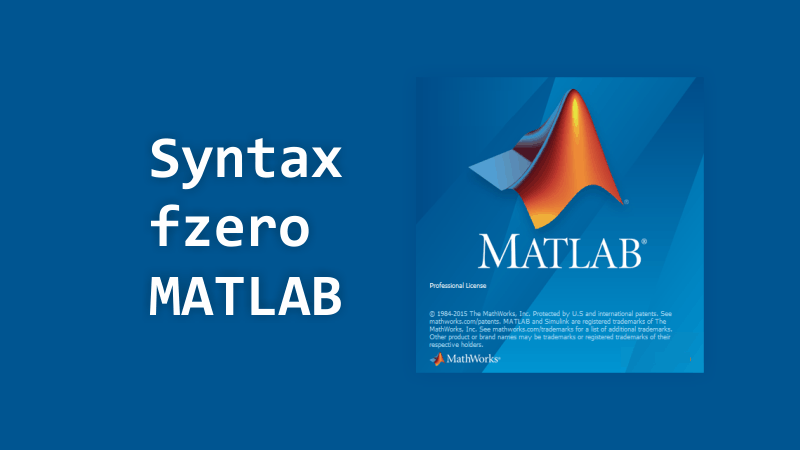
Understanding Nonlinear Equations
Nonlinear equations are mathematical expressions that do not adhere to the principle of linearity, meaning they do not graph as straight lines. These equations can take many forms, including polynomials, exponentials, or trigonometric functions. In data science and programming, understanding nonlinear equations is crucial as many real-world problems can be modeled with such equations. For instance, logistic growth in populations or the relationship between temperature and chemical reaction rates often exhibit nonlinear characteristics.
Finding the roots of these nonlinear equations is a fundamental task, particularly in algorithm development and optimization problems. A root is defined as a value of the variable that satisfies the equation, rendering it equal to zero. Efficiently locating these roots is integral to various applications, such as machine learning algorithms, where nonlinear relationships frequently describe data patterns. Consider a case in regression analysis where a model must fit non-linear data points; identifying the appropriate curve often involves solving nonlinear equations.
Moreover, many algorithms, including those implemented in fzero matlab, are designed specifically for this purpose. They utilize numerical methods to approximate the roots of nonlinear equations, thereby assisting in optimization tasks where traditional analytical solutions are infeasible. One example of a practical application is in finance, where various decision models consider nonlinear relationships between variables to determine optimal investment strategies.
In conclusion, understanding nonlinear equations and their roots is essential in data science and programming. The complexity of these equations requires sophisticated solutions like those offered by fzero matlab, enhancing the potential for effective algorithm development and optimization in real-world scenarios.
Install and Set Up MATLAB for Fzero
To effectively utilize the fzero MATLAB function for root-finding tasks, it is essential to have MATLAB installed and configured correctly on your system. Begin by ensuring that your computer meets the necessary system requirements for the version you intend to install. MATLAB runs on various operating systems including Windows, macOS, and Linux, so it is important to download the version compatible with your operating system from the official MathWorks website.
After downloading the installer, follow the prompts to complete the installation. If you are new to MATLAB, it is advisable to select the default settings, which include essential toolboxes required for fzero functionality. Particularly, the Optimization Toolbox is crucial, as it provides additional functions and tools that enhance your ability to solve complex numerical problems.
Once MATLAB is installed, launch the program and familiarize yourself with its interface. A well-organized environment is critical for productive coding. Create a dedicated folder for your MATLAB projects where you can store scripts, functions, and data files. This not only helps in maintaining an orderly workspace but also aids in efficient file management when using fzero.
Next, configure your MATLAB path to include the folder where your projects reside. This can be done using the ‘Set Path’ option found under the ‘Home’ tab. By doing so, you ensure that MATLAB recognizes and can access your scripts and functions easily. It is also beneficial to keep your scripts modular; breaking down large programs into smaller functions can improve clarity and facilitate debugging.
In summary, the proper installation and setup of MATLAB are fundamental steps for effectively utilizing the fzero MATLAB function. By ensuring you have the right version, necessary toolboxes, and an organized workspace, you will be well-prepared to tackle challenges in root-finding with confidence.
Using fzero in MATLAB: Syntax and Parameters
The ‘fzero’ function in MATLAB is an essential tool for finding the roots of nonlinear equations. Mastery of its syntax and parameters will enhance your proficiency in numerical methods, which is invaluable for data scientists and programmers alike. The primary syntax for ‘fzero’ is as follows: fzero(fun, x0)
, where fun
is the function handle, and x0
is the initial guess for the root.
The function handle can be created using either an anonymous function or by referencing a separate function file. Anonymous functions are defined using the syntax @(x) your_function_expression
. For example, fzero(@(x) x^2 - 4, 1)
will find a root for the equation x² – 4, beginning with an initial guess of 1. Alternatively, if your function is defined in a M-file, you might invoke it simply by using its name, such as in fzero('myFunction', 1)
.
In addition to the basic structure, ‘fzero’ possesses optional parameters that enhance its functionality. For instance, you may specify a search interval by incorporating two initial guesses, such as fzero(fun, [xLower, xUpper])
. This syntax instructs MATLAB to look for a root within the provided bounds. The parameters also include options to adjust the algorithm’s behavior through the optimset
function, allowing for a more tailored approach to finding solutions.
It is pivotal to ensure that the function you are analyzing changes sign over the interval defined by the initial guesses. This is fundamental since ‘fzero’ leverages the Intermediate Value Theorem and requires that at least one root exists within the specified range. Overall, familiarizing yourself with the syntax and various parameters available in ‘fzero’ is crucial for efficient root-finding and will undoubtedly contribute significantly to your MATLAB repertoire.
Practical Examples of fzero in MATLAB
The fzero function in MATLAB is a powerful tool for finding roots of nonlinear equations, and its utility can be best appreciated through practical examples. Let’s examine a few scenarios illustrating how to effectively use fzero in solving different types of functions.
Consider the first example where we want to find the root of a simple quadratic function, specifically ( f(x) = x^2 – 4 ). The root can be computed by defining the function and using fzero as follows:
f = @(x) x.^2 - 4 root = fzero(f, 2) disp(root)
This code snippet defines ( f ) as an anonymous function that computes ( x^2 – 4 ). Calling fzero with a starting guess of 2 leads to the output of the root ( x = 2 ).
Moving on to a more complex example with trigonometric functions, let’s find the root of ( f(x) = sin(x) – 0.5 ). In MATLAB, we can implement this as follows:
f = @(x) sin(x) - 0.5 root = fzero(f, 1) disp(root)
Once again, we define the function as an anonymous function and provide a starting point of 1. The result will reveal one of the possible solutions to the equation, showcasing fzero’s capability to handle periodic functions.
Lastly, for a function that may have multiple roots, such as ( f(x) = x^3 – 3x + 2 ), we can demonstrate how fzero can be employed to isolate a specific root. Here’s how:
f = @(x) x.^3 - 3*x + 2 root1 = fzero(f, -2) root2 = fzero(f, 2) disp(root1) disp(root2)
In this example, starting points of -2 and 2 allow us to identify different roots. Utilizing fzero in these varied contexts underscores its versatility and effectiveness in root-finding for both simple and complicated functions.
Common Pitfalls and Troubleshooting with fzero in MATLAB
The utilization of the fzero MATLAB function is a common approach for root-finding in mathematical problems. However, users often encounter specific pitfalls that can hinder successful execution and yield inaccurate results. Understanding these pitfalls, as well as their potential solutions, is crucial for effective problem-solving.
One prevalent issue arises when the initial guess for the root is poorly chosen. If the guess is too far from the actual root or not within the function’s domain, fzero may fail to converge. To mitigate this, it is advisable to visualize the function graphically first. This allows users to identify approximate locations of the roots more accurately, leading to a more informed initial guess.
Another common error is the implementation of the function itself. Users sometimes fail to ensure that the function is vectorized, which can lead to performance issues or erroneous outcomes. It is important to confirm that the function handles array inputs correctly, thereby enhancing both functionality and readability.
Additionally, MATLAB users may experience misleading results due to discontinuities or singularities in the function being evaluated. Such issues can cause fzero MATLAB to diverge or miscalculate the root. To address this, one should conduct a thorough analysis of the function to identify potential anomalies in the specified interval. By refining the interval or applying a different root-finding method, users can often achieve better results.
Debugging function outputs is also significant when using fzero. Establishing breakpoints and using MATLAB’s debugging tools can help identify where the process is going awry. Such techniques provide insights into the behavior of the function, allowing users to pinpoint discrepancies and refine their approach. Practicing these strategies not only minimizes common errors but also cultivates a deeper understanding of how to utilize MATLAB for complex calculations.
Learn MATLAB with Online Tutorials
Explore our MATLAB Online Tutorial, your ultimate guide to mastering MATLAB! Whether you’re a beginner or an advanced user, this guide covers everything from basic MATLAB concepts to advanced topics
Advanced Applications of Fzero in Data Science
The function ‘fzero’ in MATLAB is primarily used for finding roots of nonlinear equations. Its applications extend beyond simple root-finding tasks and can be effectively utilized in various advanced data science scenarios, including optimization problems, parameter estimation, and model fitting. As data scientists face increasingly complex models, leveraging ‘fzero’ becomes crucial for delivering insightful solutions.
One significant application of ‘fzero’ is in optimization problems where objective functions are nonlinear and require root determination. For instance, in financial modeling, analysts may need to find the break-even point of a complex equation that describes cost and revenue structures. By formulating the relevant aspects as a function and utilizing ‘fzero’, the roots can provide key insights into when profits will equal costs, which is of utmost importance in strategic planning.
Another noteworthy application of ‘fzero’ is in parameter estimation. In many scientific models, certain parameters are embedded in equations that describe empirical data relationships. By employing ‘fzero’, data scientists can estimate optimal parameter values that minimize the error between predicted and observed data. For example, in developing a regression model, ‘fzero’ can help identify parameters of polynomial functions that best fit a set of data points, leading to enhanced predictive capability.
Lastly, model fitting can also benefit greatly from the application of ‘fzero’. Consider a case where a researcher is trying to fit a sigmoid curve to bioassay data, which is common in biological studies. Using ‘fzero’, the researcher can adjust the parameters iteratively until the model adequately represents the data, thereby ensuring that the underlying trends are captured accurately. Such advanced applications of ‘fzero’ in MATLAB illustrate its capability to address complex data-driven problems effectively, ultimately empowering data scientists and programmers in their analyses.
Integration of fzero with Other MATLAB Functions
The ‘fzero’ function in MATLAB serves as a powerful root-finding tool, particularly useful for solving nonlinear equations. However, its capabilities can be significantly enhanced when integrated with other MATLAB functions and toolboxes, making it an essential component of more complex computational workflows. By leveraging these integrations, data scientists and programmers can optimize their processes and achieve more efficient solutions.
One common way to integrate ‘fzero’ is with optimization routines such as ‘fminunc’ or ‘fmincon’. These functions are designed to perform general optimization and can benefit from the root-finding abilities of ‘fzero’. For instance, if a programmer needs to find the minimum value of a function that has constraints, they may first use ‘fzero’ to identify crucial points or intersections that can guide the optimization process. This strategy not only shortens the processing time but also improves accuracy, as the values found through ‘fzero’ can serve as calibrated starting points for the optimization routine.
Another area where ‘fzero’ shines is in data visualization. By integrating it with plotting functions such as ‘plot’ or ‘fplot’, users can visualize the function whose roots they are solving for. For example, once ‘fzero’ has determined the root of a specific equation, plotting the function’s graph alongside the root can provide a clear visual representation of the solution. Using ‘hold on’ functionality allows for the inclusion of multiple plots, helping stakeholders grasp the results quickly and effectively. This integration of ‘fzero’ with visualization tools is particularly beneficial in educational settings, where illustrating complex concepts aids learning.
Combining ‘fzero’ with other functions in MATLAB not only enhances its root-finding capabilities but also broadens applications of MATLAB across various engineering, physics, and financial sectors. Through these integrations, the potential for innovative solutions is amplified, making ‘fzero’ a versatile asset in the MATLAB environment.
MATLAB Ode45 function – Practical Tutorials
Ode45 is a widely utilized numerical solver designed for the effective resolution of ordinary differential equations (ODEs). It is a part of MATLAB’s ODE suite and is specifically tailored to handle problems where the solution requires the calculation of derivatives at multiple points. The significance of Ode45 stems from its implementation of the Runge-Kutta method, which offers a robust approach to approximating solutions with high accuracy.
MATLAB Diff function – Beginners Tutorials
MATLAB diff function is an essential tool utilized in the realms of data analysis and programming. Primarily, it calculates the differences between adjacent elements in an array, offering valuable insights into trends and behaviors of data sets. Through a blend of theoretical understanding and hands-on practical examples on MATLAB, this guide aspires to empower readers to utilize MATLAB’s diff effectively, transforming raw data into actionable insights.
MATLAB conv2 Function
Convolution is a mathematical operation that combines two functions to produce a third function, expressing how the shape of one function is modified by the other. Convolution is calculated using MATLAB conv2 function. This operation is particularly crucial in various fields such as image processing, signal analysis, and systems engineering. This function is specifically designed for two-dimensional convolution, making it preferable for tasks that involve images or other matrix-based data
fft2 in MATLAB
The FFT2 function in MATLAB is crucial for performing Fourier transforms on matrices, allowing practitioners to study and manipulate two-dimensional frequency components effectively. The Fast Fourier Transform (FFT) is an algorithm that computes the discrete Fourier transform (DFT) and its inverse efficiently. The DFT converts a sequence of equally spaced samples of a function into a sequence of coefficients of sinusoidal components, revealing the frequency spectrum of the sampled signal.
MATLAB interp1 Function
The interp1 function in MATLAB serves as a fundamental tool for conducting one-dimensional interpolation on a set of data points. Interpolation is a statistical method that estimates values between two known values, allowing for more precise data analysis and management. By employing the interp1 function, users can obtain interpolated values at specified points, thus enabling a deeper analysis of datasets.
Need Help in Programming?
I provide freelance expertise in data analysis, machine learning, deep learning, LLMs, regression models, NLP, and numerical methods using Python, R Studio, MATLAB, SQL, Tableau, or Power BI. Feel free to contact me for collaboration or assistance!
Follow on Social
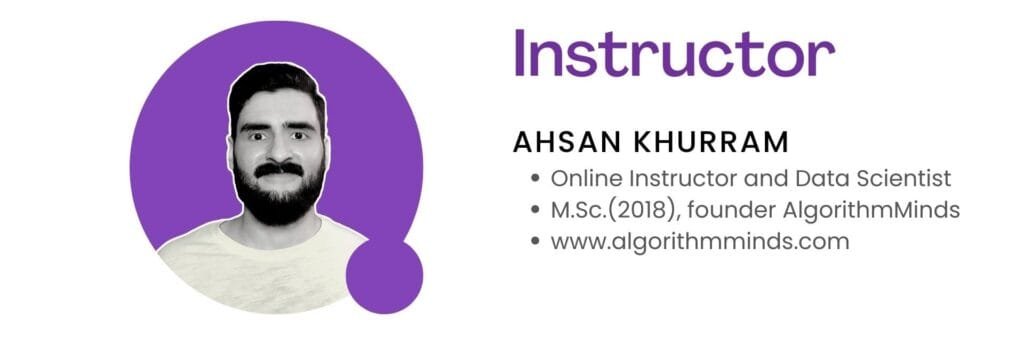
Conclusion and Key Takeaways
In this comprehensive guide, we have explored the importance of the fzero function in MATLAB, particularly for data scientists and programmers who frequently tackle numerical analysis and root finding problems. Understanding how to effectively utilize the fzero function is crucial for optimizing solutions in diverse applications, from engineering computations to mathematical modeling.
We began by outlining the core functionalities of fzero, detailing its purpose of locating a point where a given function reaches zero, which is an essential skill when aiming to solve nonlinear equations. The significance of providing appropriate inputs, such as function handles and initial guesses, was emphasized as these elements profoundly influence the success of root-finding operations. Additionally, we delved into examples that showcase various scenarios of fzero in practice, demonstrating its versatility and widespread applicability.
Moreover, we highlighted the potential challenges in using fzero, such as convergence issues and sensitivity to initial conditions. A deep understanding of these aspects equips readers with the tools needed to troubleshoot common problems encountered while employing fzero in MATLAB. Readers are thus encouraged to practice implementing these concepts in real-world data projects, fostering a practical grasp of the function’s capabilities.
Finally, as you continue your journey in mastering numerical methods, consider exploring additional resources such as MATLAB documentation, online forums, and practice datasets that can provide further insights into the use of fzero. By remaining proactive in learning and experimentation, you will enhance your proficiency in MATLAB, ultimately contributing to your effectiveness as a data scientist or programmer.
For additional assistance, we invite you to explore our comprehensive blog article featuring 60 detailed MATLAB examples. These examples are designed to help you enhance your understanding of MATLAB’s functionalities and application in various fields. Each example is thoughtfully selected to cover a range of topics, from basic syntax and operations to more advanced programming techniques, ensuring that learners at all levels can benefit from them. Whether you’re just beginning or looking to refine your skills, this resource will provide valuable insights and practical knowledge.