Importance of While Loops in MATLAB
The while MATLAB loop is a fundamental control structure in MATLAB that enables programmers to execute a block of code multiple times as long as a specified condition remains true. This iterative process is crucial for tasks where the number of iterations is not predetermined, making it especially relevant in programming scenarios that involve data analysis, simulations, and algorithm development. By allowing for condition-based execution, while loops empower engineers, data scientists, and analysts to create dynamic and responsive code.
Control flow structures play a vital role in every programming language, serving as the backbone of how we manage the flow of execution. In the world of basic MATLAB, two essential constructs— the for loop and while loop—allow programmers to run repetitive tasks efficiently and effectively, making code not just functional but dynamic and responsive!
For data scientists and analysts, the utility of while loops in MATLAB is particularly significant when processing large datasets or running simulations that require iterative calculations. In scenarios where the end result is contingent upon intermediate results, while loops facilitate the handling of emerging conditions—such as convergence criteria in optimization problems or iterative refinement of estimates—thus providing a more flexible approach compared to other looping structures like for loops.
Understanding how to effectively utilize while loops can enhance a programmer’s ability to write efficient code. For example, engineers designing control systems can implement while loops to continuously monitor sensor data until a certain operational condition is met. Meanwhile, MATLAB’s powerful computation capabilities, combined with while loops, allow for the development of complex algorithms that require repetitive calculations without prior knowledge of iteration limits. By mastering this control construct, practitioners can optimize their processes, improve performance, and streamline their workflows.
By the conclusion of this guide, readers can expect to develop a comprehensive understanding of while loops in MATLAB. They will learn when to use while loops, how to structure them effectively, and practical tips for debugging and optimization, enabling them to apply these concepts in their respective fields of work or study.
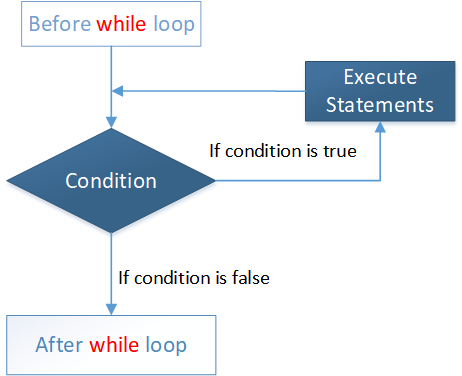
What Exactly is a While Loop in MATLAB?
A while loop in MATLAB is a control flow statement that allows for the execution of a block of code repetitively, as long as a specified condition remains true. This looping structure is particularly useful in scenarios where the number of iterations is not predetermined, as it continues to execute until the condition evaluates to false. This flexibility makes while loops an essential tool for data scientists and programmers, especially when dealing with complex data processing tasks.
The fundamental structure of a while loop consists of the keyword “while,” followed by the condition in parentheses, and finally the block of code that will be executed. This process allows for continuous evaluation of the condition, making it suitable for tasks such as monitoring data values or performing computations iteratively until a specific criterion is met. Examples include scenarios like calculating averages, managing iterative simulations, or processing data streams where data might come in dynamically.
In practice, while loops offer considerable advantages in various data-driven decision-making contexts. For instance, consider an application that requires continuous data acquisition from a sensor. A while loop can be employed to read data and process it in real-time, adjusting the operations performed based on the incoming inputs until a particular threshold or stop condition is met. The use of while loops is not limited to data collection, as they can also help in search algorithms where a certain condition must be validated before concluding the search results or altering resource allocations in a dynamic environment.
This capability to execute code repeatedly until a condition changes underscores the significance of while loops in MATLAB, solidifying their role as an indispensable feature in the arsenal of any programmer or data scientist.
Core Concepts of While Loops in MATLAB: A Step-by-Step Breakdown
The while loop is a fundamental control flow statement in MATLAB, widely used in programming and data analysis. It allows a block of code to be executed repeatedly as long as a specified condition remains true. Understanding the structure and functionality of a while loop is essential for data scientists and programmers who need to handle iterative calculations and conditional execution effectively.
At its core, the syntax of a while loop in MATLAB consists of the while keyword followed by a condition, and the loop body enclosed in end statements. The basic structure can be outlined as follows:
while condition% Code to executeend
The execution begins when MATLAB evaluates the condition. If it evaluates to true, the code block within the loop runs. After each iteration, the condition is evaluated again, and if it remains true, the loop continues. This process repeats until the condition becomes false, at which point the control exits the loop. It is crucial to ensure that the condition eventually evaluates to false; otherwise, an infinite loop may occur, leading to potential performance issues and resource exhaustion.
To illustrate this concept, consider a simple example where a while loop is used to compute the factorial of a number. The loop continues to multiply the accumulating product with decreasing integers until the counter reaches one. This demonstrates the utility of while loops in execution that depends on dynamic conditions.
Moreover, utilizing flowcharts can significantly enhance comprehension of while loops. A flowchart illustrates the decision-making process and the iterative nature of the loop visually, providing an intuitive understanding of its control flow. By engaging with these core concepts of while loops and applying them to practical examples, individuals can effectively master the use of this powerful programming construct in MATLAB.
Real-World Applications of MATLAB While Loops
While loops in MATLAB serve as powerful tools for various applications across different fields, particularly in data science, software development, and automation. These loops allow for the execution of a block of code multiple times as long as a specified condition remains true, thereby granting flexibility that is often required in real-world scenarios.
In data science, while loops can be employed in iterative data processing tasks. For instance, when working with large datasets, a data scientist might utilize a while loop to continuously load and analyze data chunks until the entire dataset has been processed. This method efficiently manages memory usage and speeds up the analysis, especially when dealing with data that cannot fit into memory all at once. Moreover, while loops can facilitate convergence in optimization problems, such as implementing gradient descent algorithms. The loop continues iterating until a predefined threshold is met, ensuring that the model is trained effectively.
Software development also greatly benefits from while loops, particularly in creating responsive applications. For example, in event-driven programming, a while loop may be used to continuously check for user input or other events, allowing the program to respond promptly. This is common in game development, where the loop processes input until the game session ends or the user quits. Additionally, a while loop can serve in scenarios where a system requires repeated checks, such as monitoring system resources or automating repetitive tasks.
Furthermore, in automation, while loops can control processes like data scraping or system monitoring. Tasks can be automated to repeat until desired criteria are met, eliminating the need for manual intervention. For instance, a script can be designed to scrape web data while conditions such as network availability or data integrity are satisfied.
Overall, the versatility of while loops in MATLAB makes them indispensable across various domains, streamlining processes and enhancing productivity in data-driven tasks.
A Comprehensive Tutorial on Implementing While Loops
To effectively utilize while loops in MATLAB, it is crucial to have the appropriate software installed. MATLAB can be downloaded from the official MathWorks website. Ensure that you select the correct version compatible with your operating system, and follow the installation instructions provided. Once installed, you can launch MATLAB and begin your programming journey.
To write your first while loop, open a new script file in MATLAB. A basic structure of a while loop consists of the keyword ‘while’, followed by a condition that must be true for the loop to execute, and ‘end’ to signify its conclusion. For instance, consider the following simple code snippet:
count = 1 while count < = 5disp(count) count = count + 1 end
This code will display the numbers from 1 to 5 in the command window. The loop continues executing as long as the condition ‘count <= 5’ remains true. It is vital to ensure that the loop will eventually terminate; otherwise, you may inadvertently create an infinite loop, which can occur if the loop’s condition remains valid indefinitely.
Creating a Simple Loop
counter = 0;
while counter < 3
disp("Iteration " + counter);
counter = counter + 1;
end
Dynamic Loop Conditions
n = 5;
result = 1;
while n > 0
result = result * n;
n = n - 1;
end
disp(result);
Visualizing Results
x = 0;
step = pi / 20;
while x <= 2 * pi
plot(x, sin(x), 'bo');
hold on;
pause(0.1);
x = x + step;
end
hold off;
Different approaches can achieve similar results. For instance, you may use a for loop to accomplish tasks similarly. However, while loops are beneficial when the number of iterations is not predetermined. One effective way to test your code is by incorporating troubleshooting techniques, such as adding breakpoints and using the ‘disp’ command to evaluate variable values during execution.
As a pro tip, always comment your code, as this practice enhances readability and helps other programmers, or even yourself, understand the logic later. By starting with these fundamentals, you can confidently explore the various applications of while loops in MATLAB, gradually building your programming skills. Experiment with your loops to better understand their functionality in practical scenarios.
For additional assistance, we invite you to explore our comprehensive blog article featuring 60 detailed MATLAB examples. These examples are designed to help you enhance your understanding of MATLAB’s functionalities and application in various fields. Each example is thoughtfully selected to cover a range of topics, from basic syntax and operations to more advanced programming techniques, ensuring that learners at all levels can benefit from them. Whether you’re just beginning or looking to refine your skills, this resource will provide valuable insights and practical knowledge.
Watch Tutorial for While Loop in MATLAB
In this in-depth tutorial, we explore the core concepts of loops like while and for in MATLAB from the mathworks, with practical examples for every beginner to expert level. Whether you’re looking to understand the structure of for loops, while loops, or how to leverage the break and continue statements to control flow, this video covers it all!
What you’ll learn in this video:
- For Loop Example: Learn how to use the for loop to repeat a block of code a specific number of times.
- While Loop Example: Master the while loop for executing code as long as a condition is true.
- Break Statement: See how to exit loops early with the break statement when certain conditions are met.
- Continue Statement: Learn how to skip over parts of your loop with the continue statement.
- Nested Loops: Understand how to work with nested loops for more complex operations and data processing.
Common Challenges with While Loops and How to Overcome Them
While loops in MATLAB are powerful tools for controlling the flow of a program, yet they come with their own set of challenges. One prevalent misconception is that while loops will always execute at least once. This can be misleading; the loop may never run if the condition is false from the beginning. Students while using MATLAB should ensure that the condition is appropriately set to avoid this trap. A common solution is to initialize loop variables cautiously to guarantee that the loop condition is met.
Another challenge often faced by MATLAB users involves infinite loops. An infinite loop occurs when the exit condition of the while loop is never met, which can lead to a program hang. Users should implement checks and balances within their loop logic, such as incrementing a variable or adding a timeout mechanism, to provide a way for the loop to terminate properly. Debugging tools in MATLAB can be instrumental in monitoring loop behavior during runtime.
Additionally, proper placement of the loop condition is critical for effective execution. Misplaced or missing updates can result in unexpected behavior. Thus, it’s best practice to update loop variables as early as possible within the loop body. Comments in the code can also assist in understanding the flow and purpose of the while loop, preventing confusion over variable changes and conditions. Using clear, descriptive variable names contributes further to clarity, making the code more readable and maintainable.
To enhance understanding, testing your while loops with simple cases can clarify their behavior and build confidence before moving on to more complex scenarios. In conclusion, recognizing these common challenges and applying best practices can greatly enhance a programmer’s proficiency in using while loops within MATLAB, allowing for more robust data processing solutions.
Recap of Key Points and Practical Insights
As we reflect on the utilization of the while loop in MATLAB, several key points emerge that are essential for data scientists and programmers alike. The while loop serves as a fundamental control structure, enabling users to execute a block of code repeatedly based on specified conditions. This functionality is invaluable in scenarios where the number of iterations is not predetermined, providing flexibility in programming solutions.
Throughout this discussion, we have explored the syntax and structure of while loops, elucidating how they function within MATLAB’s environment. The tutorial provided practical examples that demonstrate the effective application of while loops in real-world scenarios, showcasing their role in tasks such as data analysis, algorithm design, and automation of repetitive operations. A clear understanding of the while loop’s mechanics not only improves code efficiency but also enhances problem-solving capabilities.
Furthermore, practical insights highlighted the importance of incorporating proper termination conditions within while loops. This practice is critical to prevent infinite loops, which can lead to program crashes and resource exhaustion. By combining thorough debugging techniques and strategic planning, programmers can ensure robust implementations of the while loop, tailored to their project requirements.
In addition to reaping the benefits of the while loop in specific projects, it is essential for readers to consider the broader implications of mastering this concept. Understanding how to effectively use while loops can lead to more optimized code and streamlined data processing workflows. As you venture into your own programming projects, consider the various applications of this control structure, and how it can be leveraged to enhance both performance and accuracy in your work with MATLAB.
Exploring Additional Resources for Mastery
Embarking on the journey to master the while loop in MATLAB requires access to quality resources and tools that cater to various learning styles. One of the most effective methods for augmenting your understanding of MATLAB programming is through online tutorials. Websites like MathWorks offer comprehensive video tutorials that not only explain the syntax and functionality of the while loop but also present practical examples to reinforce learning.
For those inclined toward structured learning, textbooks can serve as an invaluable resource. “MATLAB for Engineers” by Holly Moore, for instance, contains detailed sections on conditional statements and looping constructs, including the while loop. Such textbooks often contain exercises that can deepen your comprehension and facilitate hands-on experience.
Incorporating software tools such as MATLAB’s Live Editor can enhance the learning process. It allows you to write scripts and see results in real-time, fostering a better grasp of programming concepts. Moreover, engaging with the MATLAB community through forums, user groups, and social media can provide a platform for exchanging knowledge and experiences with others facing similar challenges. Resources like Stack Overflow and MATLAB Central can be extremely beneficial in resolving specific queries or learning from others’ insights.
Learn MATLAB with Online Tutorials
Explore our MATLAB Online Tutorial, your ultimate guide to mastering MATLAB! Whether you’re a beginner or an advanced user, this guide covers everything from basic MATLAB concepts to advanced topics
Tailored Support and Professional Services
To further augment your learning experience, consider seeking professional support. I offer personalized guidance tailored to your specific needs, whether you are a beginner or seeking advanced techniques. This individualized approach can significantly streamline your learning path and ensure that you grasp MATLAB’s capabilities effectively. Engaging with a professional not only provides direct assistance but also enriches your learning process by integrating real-world applications into your training.
Need Help in Programming?
I provide freelance expertise in data analysis, machine learning, deep learning, LLMs, regression models, NLP, and numerical methods using Python, R Studio, MATLAB, SQL, Tableau, or Power BI. Feel free to contact me for collaboration or assistance!
Follow on Social
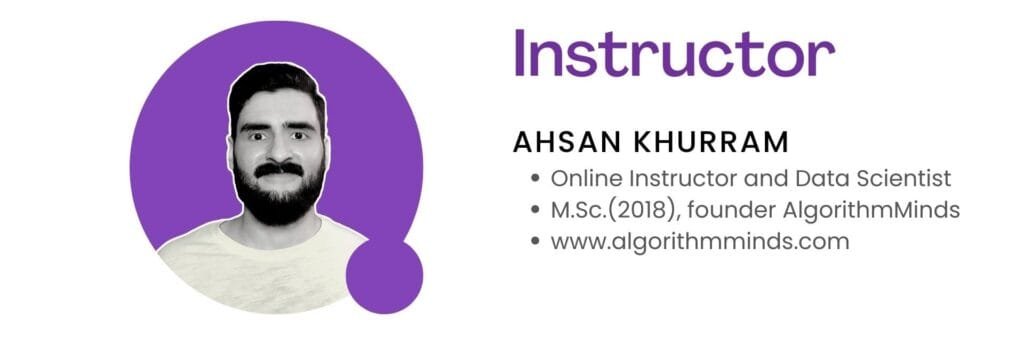
Frequently Asked Questions about While Loops in MATLAB
While loops are a fundamental component of programming in MATLAB, but they can often lead to confusion or misapplications if not fully understood. One common question pertains to the structure of while loops. A typical while loop consists of a loop condition that is evaluated before each iteration. If the condition evaluates to true, the code inside the loop executes. Programmers new to MATLAB often struggle with ensuring their loop eventually terminates. It’s crucial to modify a variable within the loop that influences the condition; otherwise, they risk creating an infinite loop.
Another prominent question addresses the difference between while loops and for loops in MATLAB. While loops allow for more dynamic repetition since the number of iterations is not predetermined. This is particularly advantageous when the loop needs to continue until a certain condition is met rather than iterating a specific number of times. In contrast, for loops are typically utilized when the termination condition can be quantified upfront. Data scientists may prefer while loops in scenarios where conditions evolve based on data processing, as this provides more flexibility.
Error handling is also a significant concern for users of while loops in MATLAB. It is advisable to use try-catch constructs within the loop to manage potential runtime errors gracefully. This approach not only improves the robustness of the code but also provides a mechanism to log errors without terminating the entire program. In situations where the loop’s primary task is to process data inputs, having error handling in place ensures data integrity and allows for smoother program execution.
In summary, understanding while loops is essential for effectively utilizing MATLAB. Addressing common questions regarding their structure, differences from for loops, and error handling can empower programmers to write more effective and efficient code. By mastering these aspects, one can significantly enhance their MATLAB skills and streamline their programming tasks.