Introduction to Error Handling in Python
Error handling is a fundamental aspect of programming, and Python provides robust mechanisms like Try and Except for managing exceptions that may arise during the execution of code. It is critical for developers to effectively manage errors to enhance the stability and reliability of their applications. Effective error handling allows developers to anticipate potential issues, respond to them appropriately, and maintain control over the flow of their programs. In contrast, failing to address exceptions can lead to unpredictable behavior, application crashes, and poor user experience.
In Python, errors can be broadly categorized into two main types: syntax errors and runtime errors. Syntax errors occur during the parsing phase when the code written by the programmer does not follow the correct structure or rules defined by the Python language. These errors are easily identifiable at the development stage, as they prevent the program from running entirely until the issue is corrected. On the other hand, runtime errors occur while the program is running, potentially due to exceptional circumstances such as division by zero, invalid input types, or out-of-bound array access.
The robust error handling in Python is primarily achieved through the use of try and except blocks. By enclosing code that may potentially raise exceptions within a try block, developers can catch those exceptions in the corresponding except block. This approach enables developers to handle errors gracefully, providing the opportunity to implement alternative logic and inform the user appropriately about the nature of the issue without abruptly terminating the application. Mastering this skill is essential for Python developers, as it directly contributes to writing resilient and user-friendly applications, ultimately leading to a more pleasant and efficient programming experience.
What is Try and Except in Python?
The try
and except
keywords in Python are integral components of error handling, allowing developers to manage exceptions that may arise during the execution of a program. Exception handling is crucial for creating robust applications, as it helps prevent crashes by catching errors and allowing the program to continue running in a controlled manner. The basic syntax consists of a try
block that contains code that may potentially raise an exception, and one or more except
blocks that specify how to respond to those exceptions.
Understanding Python’s Error Hierarchy
Before we dive into try-except
blocks, it’s crucial to understand Python’s exception hierarchy. All exceptions inherit from the base BaseException
class, but most exceptions you’ll handle derive from Exception
. Here’s a breakdown of common exception types:
- ArithmeticError: Base class for math-related errors
ZeroDivisionError
: Division/modulo by zeroOverflowError
: Result too large to represent
- LookupError: Base class for key/index errors
IndexError
: Sequence subscript out of rangeKeyError
: Dictionary key not found
- OSError: System-related errors
FileNotFoundError
: Missing filePermissionError
: Insufficient permissions
- RuntimeError: Errors that don’t fit other categories
- TypeError: Operation on inappropriate type
- ValueError: Correct type but inappropriate value
The Anatomy of Try-Except Blocks
The basic structure of Python’s error handling consists of four possible clauses:
try:
# Code that might raise exceptions
except ExceptionType:
# Handle specific exception
except (ExceptionType1, ExceptionType2):
# Handle multiple exceptions
else:
# Run if no exceptions occurred
finally:
# Always execute, with or without exceptions
Let’s examine each component in detail:
1. The Try Block
This is where you place code that might raise exceptions. Best practices include:
- Keep try blocks as small as possible – only wrap code that might actually raise exceptions
- Avoid putting entire functions in try blocks unless the whole function is error-prone
- Document what exceptions might be raised using docstrings
2. Except Clauses
The except block catches and handles exceptions. There are several patterns:
# Basic exception handling
try:
file = open('data.txt')
except FileNotFoundError:
print("File not found, using default data")
data = default_data
# Catching multiple exceptions
try:
config = load_config()
except (FileNotFoundError, PermissionError) as e:
print(f"Config error: {e}")
config = default_config
# Getting exception details
try:
result = calculate()
except ValueError as e:
print(f"Calculation failed: {str(e)}")
print(f"Exception type: {type(e).__name__}")
print(f"Traceback: {e.__traceback__}")
3. The Else Clause
Often overlooked, the else clause runs only when no exceptions occur. It’s perfect for code that depends on the try block’s success but shouldn’t be in the try block itself.
try:
connection = connect_to_database()
except DatabaseError:
print("Failed to connect to database")
else:
# Only execute if connection succeeded
records = connection.query("SELECT * FROM users")
process_records(records)
connection.close()
4. The Finally Clause
The finally block always executes, making it ideal for cleanup operations. Even if an exception occurs and isn’t caught, or if you return from a function, finally will run.
file = None
try:
file = open('important.data', 'w')
file.write(sensitive_data)
except IOError as e:
print(f"Failed to write data: {e}")
finally:
if file is not None:
file.close() # Always close the file
secure_erase_temp_files() # Always clean up
Advanced Exception Handling Patterns
1. Exception Chaining
Python 3 introduced explicit exception chaining with raise from
:
try:
import third_party_module
except ImportError as e:
raise ImportError("Required dependency missing") from e
This preserves both the original and new exceptions in the traceback.
2. Context Managers for Resource Handling
While finally
works, context managers (using with
statements) are often cleaner:
# Instead of:
file = None
try:
file = open('data.txt')
process(file)
finally:
if file:
file.close()
# Use:
with open('data.txt') as file:
process(file) # Automatically closed
3. Logging Exceptions Properly
Proper exception logging helps with debugging:
import logging
import traceback
try:
risky_operation()
except Exception:
logging.error("Operation failed", exc_info=True)
# Or equivalently:
logging.exception("Operation failed") # Automatically includes traceback
4. Creating Exception Hierarchies
For complex applications, define your own exception hierarchy:
class DatabaseError(Exception):
"""Base class for database errors"""
class ConnectionError(DatabaseError):
"""Connection-related failures"""
class QueryError(DatabaseError):
"""Query execution errors"""
def execute_query():
try:
# Database operations
except ConnectionResetError:
raise ConnectionError("Connection lost") from None
except MalformedQuery:
raise QueryError("Invalid query syntax")
Real-World Use Cases
1. Web API Requests
Handling HTTP requests requires robust error handling:
import requests
from requests.exceptions import RequestException
def fetch_data(url):
try:
response = requests.get(url, timeout=5)
response.raise_for_status() # Raises HTTPError for bad status
return response.json()
except RequestException as e:
if isinstance(e, requests.Timeout):
retry_request(url)
elif response.status_code == 404:
raise NotFoundError(f"Resource not found: {url}")
else:
raise APIError(f"Request failed: {str(e)}")
2. File Processing Pipeline
A complete file processing example with multiple error checks:
def process_files(filepaths):
processed = []
for path in filepaths:
try:
with open(path, 'r') as f:
try:
data = json.load(f)
except json.JSONDecodeError:
print(f"Invalid JSON in {path}, skipping")
continue
try:
result = transform_data(data)
except DataValidationError as e:
print(f"Invalid data in {path}: {e}")
continue
except FileNotFoundError:
print(f"File {path} not found, skipping")
continue
except PermissionError:
print(f"No permission to read {path}, skipping")
continue
except Exception as e:
print(f"Unexpected error processing {path}: {e}")
continue
processed.append(result)
return processed
Performance Considerations
While exception handling is crucial, it’s important to use it judiciously:
- Try blocks are cheap when no exceptions occur
- Exception handling is expensive compared to condition checking
- Prefer condition checking for common cases you expect to handle
Example of when to use conditions vs exceptions:
# Good - Checking a common condition
if key in my_dict:
value = my_dict[key]
else:
handle_missing_key()
# Better for uncommon cases
try:
value = my_dict[key]
except KeyError:
handle_missing_key()
Best Practices Summary
- Be specific in exception handling – catch only what you can handle
- Document exceptions your functions might raise
- Preserve context using
raise from
when appropriate - Clean up resources using
finally
or context managers - Log exceptions with sufficient context for debugging
- Create meaningful custom exceptions for your domain
- Don’t overuse exceptions for flow control
- Test error paths as thoroughly as happy paths
Video Tutorial on Try and Except in Python
In this video, we’ll delve into the intricacies of try…except blocks, handling specific exceptions like ZeroDivisionError or IndexError, and utilizing try with else. What You’ll Learn:
- try…except Block: Understand the basics of handling exceptions.
- Handling Specific Exceptions: Learn how to manage specific errors like ZeroDivisionError and IndexError effectively.
- Using try with else: Discover how to execute code when no exceptions are raised, making your programs more robust and error-proof.
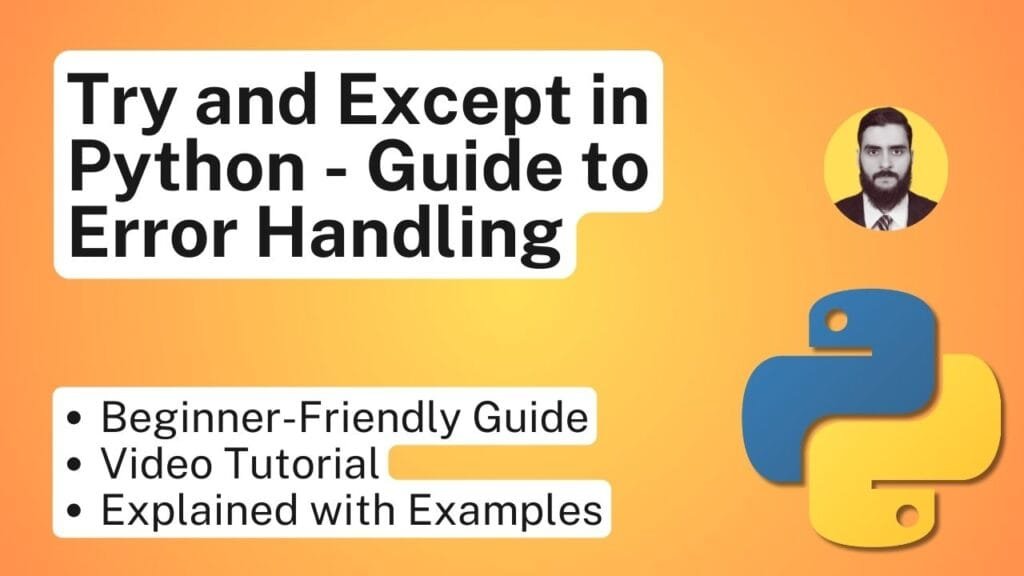
When Python encounters an error within the try
block, it immediately stops executing the rest of the code in that block and looks for a matching except
clause to handle the error. If a corresponding exception handler is found, control passes to that block, where developers can define specific responses to different types of errors. This mechanism enables users to handle expected and unexpected issues gracefully, thus ensuring an uninterrupted user experience.
For instance, consider the following example of a simple try
and except
statement:
try:
num = int(input("Enter a number: "))
result = 100 / num
except ValueError:
print("Invalid input! Please enter a valid number.")
except ZeroDivisionError:
print("Cannot divide by zero!")
In this case, the user is prompted to enter a number. If the user inputs a non-integer value, a ValueError
will be raised and handled accordingly. Similarly, if the input is zero, a ZeroDivisionError
will occur, which is also caught by the except
clause. This illustrates how the try
and except
structure is essential for handling errors dynamically and maintaining code stability in Python applications.
Need Help in Programming?
I provide freelance expertise in data analysis, machine learning, deep learning, LLMs, regression models, NLP, and numerical methods using Python, R Studio, MATLAB, SQL, Tableau, or Power BI. Feel free to contact me for collaboration or assistance!
Follow on Social
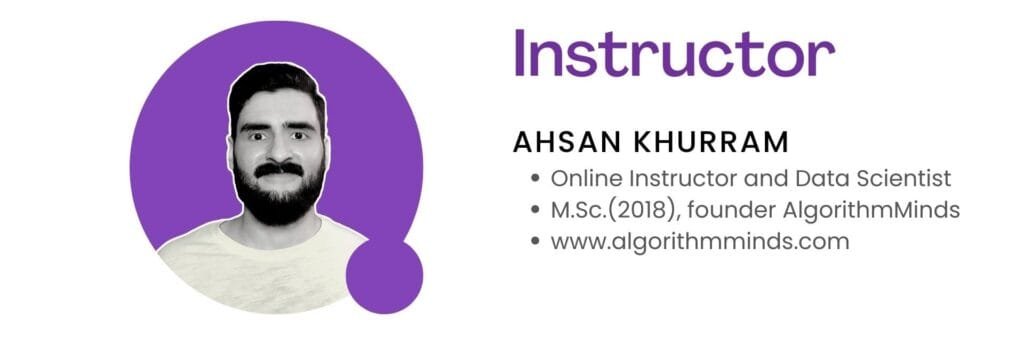
How to Use Try and Except in Python
The try
and except
constructs in Python are essential for managing errors and ensuring your program executes smoothly. They allow you to handle exceptions effectively, preventing unexpected crashes. To implement these constructs, you begin by writing a block of code that might raise an exception within the try
section. If the code within the try
block causes an error, the control will immediately transfer to the corresponding except
block, where you can define how the program should respond to that specific error.
Here is a basic structure of how to use try
and except
in Python:
try:
# Code that may generate an exception
x = int(input("Please enter a number: "))
print("You entered:", x)
except ValueError:
# This block executes if a ValueError occurs
print("That's not a valid number!")
In this example, if the user inputs a non-integer value, the program catches the ValueError
using the except
clause and prompts an appropriate message instead of crashing. You can also define multiple except
blocks to handle different types of exceptions separately. This capability ensures your Python program can react gracefully to various error conditions.
For example, if performing file operations, you can utilize try
and except
like this:
try:
with open('file.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("The file was not found.")
except IOError:
print("An error occurred trying to read the file.")
In this scenario, the program handles both FileNotFoundError
and IOError
, thereby making your error handling more robust. Using try
and except
allows programmers to write clean, efficient, and resilient code that can adapt to unforeseen circumstances, significantly improving user experience.
Learn Python with Free Online Tutorials
This guide offers a thorough introduction to Python, presenting a comprehensive guide tailored for beginners who are eager to embark on their journey of learning Python from the ground up.
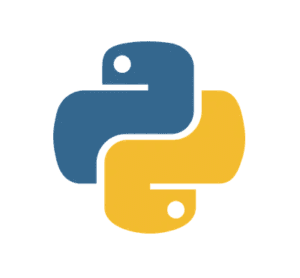
Multiple Except Clauses for Try and Except in Python
In Python, the try and except structure allows for sophisticated error handling, making it possible to create robust applications. One of the key features of this mechanism is the ability to utilize multiple except clauses within a single try block. This flexibility enables developers to catch and handle various types of exceptions that may arise during program execution.
When utilizing multiple except clauses, the first step is to implement a try block that contains the code prone to errors. Following this, various except statements can be added to handle specific exceptions. For instance, if you are performing operations that may raise both a ValueError and a TypeError, you can define separate except clauses for each type. This specificity is vital in ensuring that the right errors are addressed appropriately.
Consider the following code example:
try:
user_input = int(input("Please enter a number: "))
result = 10 / user_input
except ValueError:
print("Invalid input: Please enter a valid integer.")
except ZeroDivisionError:
print("Error: Division by zero is not allowed.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
In this example, three except clauses are utilized to handle different scenarios. The ValueError
exception is caught if the user enters a non-integer, while the ZeroDivisionError
exception is triggered if the input is zero. The last clause utilizes a more general catch-all syntax by using Exception
to handle any unforeseen errors.
This approach not only enhances the readability of the code but also allows for clearer communication of what went wrong, which can be particularly beneficial during debugging. By employing multiple except statements, developers can ensure a tailored response to various error conditions, ultimately leading to a more resilient application.
Else and Finally Blocks
In Python, the try
and except
constructs provide a robust mechanism for error handling, allowing developers to gracefully manage exceptions that may arise during the execution of a program. In addition to these primary blocks, Python also includes optional else
and finally
blocks, which can further enhance the control flow of error management.
The else
block is executed if the code within the try
block runs without any exceptions. This feature is particularly useful when you want to separate the error-handling logic from the code that should execute when no errors occur. For instance, if a function computes a value that might raise an exception, the else
block allows you to process the result only if the computation is successful. Here is an example:
try:
result = divide(10, 2)
except ZeroDivisionError:
print("Cannot divide by zero.")
else:
print("The result is:", result)
In this example, if the division is successful, the message with the result will be printed; otherwise, the except
block will handle the exception.
The finally
block, on the other hand, is executed regardless of whether an exception was raised or not. This block is ideal for executing cleanup actions, such as closing files or releasing resources that need to be managed after the main operation. The definitive characteristic of the finally
block is its ability to ensure that the code within it is run in all circumstances, serving as a fail-safe mechanism. Here is a pertinent illustration:
try:
file = open('example.txt', 'r')
data = file.read()
except FileNotFoundError:
print("File not found.")
finally:
file.close()
In this example, irrespective of whether the file was found, the file will be closed, demonstrating the purpose of the finally
block in effective resource management.
Raising Exceptions
In Python, exceptions are events that can modify the normal flow of a program’s execution. While the language provides built-in exceptions for various error categories, there are instances when it is beneficial to create custom exceptions. This is where the raise
keyword comes into play, allowing developers to trigger exceptions intentionally when certain conditions arise. Understanding how to efficiently use the raise
keyword can improve the clarity and robustness of your error handling in Python applications.
Raising exceptions can be particularly useful in scenarios where the built-in exceptions do not fit the specific context of your application. By creating custom exceptions, you can provide more meaningful error messages, making it easier for yourself and others to debug issues. For example, consider a file-based application that requires specific data formats; if the provided data does not conform, you can raise a custom exception to reflect this violation of expectations.
To define and raise a custom exception in Python, you start by subclassing the base Exception
class. Below is a simple example illustrating how to create and raise a custom exception:
class DataFormatError(Exception):
pass
def validate_data(data):
if not isinstance(data, dict):
raise DataFormatError("Data must be a dictionary.")
In this example, a custom exception called DataFormatError
is defined, which can be raised when the data format does not meet the expected specifications. When the validate_data
function is called with an argument that doesn’t meet this criterion, the custom exception gets triggered, allowing developers to leverage Python’s try
and except
constructs to capture and manage these errors effectively. Understanding how to use the raise
keyword in Python not only enhances error handling but also empowers developers to create more maintainable code.
Best Practices for Error Handling with Try and Except
When employing the try and except in Python, it is essential to follow best practices that enhance the robustness and maintainability of your applications. One of the paramount practices is to avoid catching overly broad exceptions. Instead of using a generic exception clause that captures all types of exceptions, aim to specify the particular exceptions your code may encounter. This approach provides clarity in your error-handling logic and aids in diagnosing issues more effectively.
Another critical aspect of using the except python clause involves appropriate logging of errors. It is not sufficient merely to catch exceptions; it is vital to log them in a way that supports future debugging efforts. Implementing logging allows developers to track issues as they arise, offering insights into the nature and frequency of errors encountered by the application. Utilizing the built-in logging module in Python provides a structured way to manage error logs, which can be invaluable for both development and ongoing maintenance.
Code readability should also be a priority when integrating the try except catch python structure into your codebase. Maintaining clean and succinct code enhances collaboration among developers and aids in the long-term understanding of the code’s functionality. To improve readability, keep the code within the try block minimal, ensuring that any logic prone to exceptions is well-contained. This practice also facilitates easier identification of potential errors. Additionally, providing meaningful messages within the except block can assist in communicating error details to users or developers.
Ultimately, by applying these best practices, developers can create clean, efficient, and effective error-handling mechanisms using python try except except. This approach not only helps in managing errors but also contributes to overall code quality and maintainability.
Common Use Cases for Try and Except
The use of try and except in Python is essential for robust error handling across various applications. One prevalent use case is file handling, where developers read from or write to files. Utilizing a try
block when opening a file can prevent the program from crashing if the file does not exist or is inaccessible. For instance, using try:
to open a file allows the subsequent except IOError:
block to manage file-related errors gracefully, prompting users about issues without abrupt termination.
Another critical area where try except is immensely useful is in network requests. When a program communicates over a network, there is always the possibility of connectivity issues. By implementing a try
block while making a network request, developers can catch exceptions specifically related to that operation, such as connection timeouts or endpoint failures. This usage not only enhances the program’s stability but also improves user experience by providing informative error messages rather than generic failures.
User input validation is a further common scenario that benefits from the inclusion of try except catch Python constructs. When accepting input from users, it is vital to ensure that the provided data adheres to expected formats. For example, if a user is expected to input a number, a try
statement can attempt to convert the input into an integer. If the conversion fails, the corresponding except ValueError:
block can handle the error, allowing the program to prompt the user again for valid input without crashing.
By integrating try-except structures into these areas, developers can significantly enhance the reliability and user-friendliness of their applications, ensuring smoother and more predictable operation even when unexpected issues arise.
Conclusion: The Importance of Error Handling in Python
Error handling is a critical aspect of Python programming that cannot be overlooked. As applications grow in complexity, the likelihood of encountering errors increases, making robust error handling essential for maintaining application reliability and performance. The use of the try and except in Python offers developers a structured way to address possible issues that may arise during code execution. By anticipating errors and implementing appropriate handlers, programmers can ensure that their applications continue to run smoothly, even when faced with unexpected conditions.
Implementing try except catch in Python allows developers to gracefully manage exceptions, whether that means logging an error, providing feedback to the user, or attempting a safe recovery. Ignoring potential exceptions may result in application crashes or unintended behaviors, which can compromise user experience. Hence, the utilization of the python try except mechanism not only protects the integrity of the software but also enhances user satisfaction, as it leads to a more robust and reliable product.
Adopting best practices in error handling, such as using specific exceptions and only broad exceptions when absolutely necessary, goes a long way towards improving code quality. Furthermore, understanding exception types and the context in which they can occur empowers developers to write more defensive code. This familiarity with the except Python construct ultimately augments a programmer’s skill set, making them more adept at problem-solving in real-world scenarios.
Mastering Python’s try
and except
is about more than just preventing crashes – it’s about creating robust, maintainable applications that handle edge cases gracefully. By understanding the exception hierarchy, using the full try-except-else-finally syntax, and applying the patterns we’ve covered, you’ll be able to write Python code that’s both more reliable and easier to debug.
Remember that good error handling:
- Makes failures visible and debuggable
- Preserves application state when errors occur
- Provides meaningful feedback to users
- Follows consistent patterns across your codebase
As you implement these techniques, you’ll find your Python programs becoming more resilient and professional-grade. The next time an error occurs, you’ll be ready to handle it properly!
In summary, leveraging Python’s error handling capabilities through effective use of the try and except blocks is vital for developing resilient applications. By thoughtfully incorporating these error handling strategies, developers can significantly enhance the dependability of their code, leading to improved outcomes in their software development projects.