Introduction to For Statement in MATLAB
The for loop is a fundamental control structure in MATLAB programming that enables developers to execute a block of code repetitively based on a specified condition. It is particularly advantageous when dealing with tasks that require iteration, such as processing array elements or performing repetitive calculations. The primary function of the for statement in MATLAB is to automate repetitive tasks, thereby enhancing efficiency and reducing the potential for human error.
The syntax of a for loop in MATLAB is straightforward and consists of three main components: the initialization, the condition, and the update. The general structure is as follows:
for index = startValue:endValue% Code to be executedend
The index
variable is initialized to startValue
, and the loop continues to execute until the index
reaches endValue
. Within each iteration of the loop, a designated block of code is executed, allowing for dynamic processing of data. After each iteration, the for statement automatically updates the index
variable, typically by incrementing it by one unless otherwise specified.
For loops are widely used in MATLAB due to their versatility. They are capable of handling complex algorithms, performing data analysis, and simplifying mathematical computations. By structuring code within a for loop, programmers can efficiently manage tasks that involve numerous repetitive operations. This ability to streamline the workflow is vital in various applications, making the for loop an indispensable tool in the MATLAB programming environment.
Using a Basic For Statement in MATLAB
Creating a basic for loop in MATLAB is an essential skill for anyone looking to leverage the power of this programming language for numerical computations and data processing. The primary syntax for a for loop is relatively straightforward and consists of the following structure: for index = startValue:endValue
. Here, index
serves as the loop variable, while startValue
and endValue
determine the loop’s range.
To better understand the creation of a for loop in MATLAB, let’s consider a practical example. Suppose you want to calculate the squares of integers from 1 to 5. You can implement this using the for statement in MATLAB like so:
for i = 1:5square = i^2;disp(square);end
In this example, the loop initializes the variable i
at 1 and increases it by 1 after each iteration until it reaches 5. The expression i^2
computes the square of i
, and the disp
function displays each result. It is also possible to specify a custom increment value. For instance, if you want to increment by 2, you would write:
for i = 1:2:5square = i^2;disp(square);end
This loop will calculate the squares for i
values of 1, 3, and 5, effectively showcasing flexibility in MATLAB’s for statement. However, while using for loops, it is essential to avoid common pitfalls such as forgetting to update the loop variable or incorrectly specifying the start and end values. These errors can prevent the loop from executing as expected or lead to infinite loops. Thus, careful attention should be paid to the structure of the for loop for optimal performance.
Understanding For Loop Control Statements
Loop control statements play a critical role in managing the flow of execution within loops, significantly enhancing their functionality in programming. In the context of MATLAB, two primary loop control statements—’break’ and ‘continue’—are often employed to facilitate more complex loop behaviors. Understanding how these commands operate within a for
statement in MATLAB is essential for creating efficient and effective scripts.
The ‘break’ statement is utilized to terminate the execution of a loop, thereby allowing the program to exit the loop immediately, regardless of the loop’s end condition. This command becomes particularly useful when the user needs to stop processing based on specific criteria that may arise during iteration. For example, if the intended functionality includes searching for a value within a dataset, the ‘break’ command can be strategically positioned following a conditional check. If the target value is found, the loop can be exited promptly, enhancing execution speed and performance.
Conversely, the ‘continue’ statement serves a different purpose within the loop structure. When encountered, it immediately halts the current iteration and proceeds to the next iteration of the loop. This can be highly advantageous when certain conditions necessitate skipping to the next loop cycle without executing the remaining statements in the current iteration. For instance, when processing data in a for
statement, it might be necessary to skip over invalid or unwanted data points without disrupting the entire loop’s functionality.
By effectively integrating these loop control statements, MATLAB programmers can create more dynamic and responsive for
loops, facilitating advanced manipulation of data structures. Mastering the application of ‘break’ and ‘continue’ empowers users to optimize their scripts and respond adeptly to various programming scenarios.
Combining For Statement in MATLAB with Conditional Statements
The integration of for loops and conditional statements in MATLAB is an essential skill for anyone looking to enhance their programming efficiency and flexibility. For loops facilitate the execution of a block of code a specified number of times, while conditional statements (if, elseif, and else) allow for the execution of code selectively based on certain conditions. This combination empowers programmers to create more dynamic and responsive code.
To effectively employ this combination, consider a scenario where you want to process a set of numerical data and categorize each number as either even or odd. The code snippet below illustrates how to achieve this using a for statement in MATLAB along with an if-else structure:
for i = 1:10if mod(i, 2) == 0disp([num2str(i) ' is even.']);elsedisp([num2str(i) ' is odd.']);endend
In this example, the for loop iterates through the numbers 1 to 10. The mod function determines whether the current number is even or odd. If the condition inside the if statement evaluates to true (the number is even), the corresponding message is displayed; otherwise, the else block executes, stating that the number is odd.
More complex conditions can be incorporated using the elseif branch. For instance, if we want to categorize numbers based on their divisibility by both 2 and 3, we might write:
for i = 1:15if mod(i, 2) == 0 && mod(i, 3) == 0disp([num2str(i) ' is divisible by both 2 and 3.']);elseif mod(i, 2) == 0disp([num2str(i) ' is even.']);elsedisp([num2str(i) ' is odd.']);endend
This example highlights how the control flow can become nuanced, enabling programmers to perform calculations or output messages based on multiple criteria. The combination of for loops with conditional statements not only increases the versatility of your code but also enhances its readability and maintainability. Mastering this technique is an invaluable step in mastering programming in MATLAB.
Need Help in Programming?
I provide freelance expertise in data analysis, machine learning, deep learning, LLMs, regression models, NLP, and numerical methods using Python, R Studio, MATLAB, SQL, Tableau, or Power BI. Feel free to contact me for collaboration or assistance!
Follow on Social
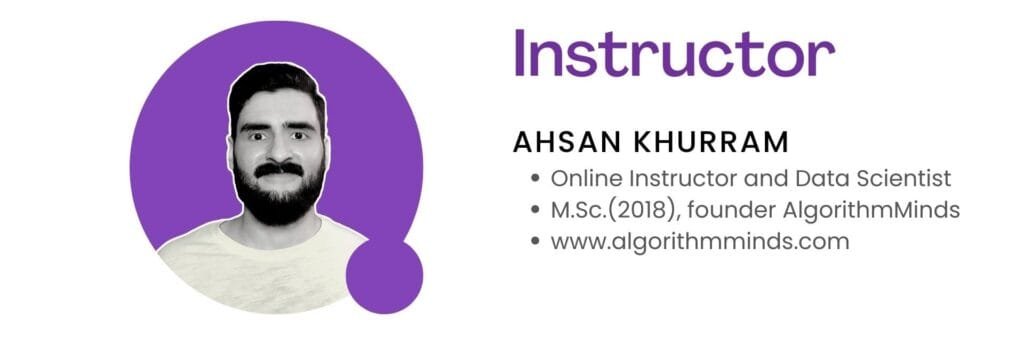
Common Applications of For Statement in MATLAB
For loops in MATLAB are invaluable tools that enable programmers to automate repetitive tasks efficiently. One of the most common applications of the for statement in MATLAB is iterative calculations, where a specific operation needs to be executed multiple times. For instance, if one were to calculate the factorial of a number, a for loop allows the coder to multiply sequential integers without writing repetitive code. This not only simplifies the code but also improves clarity and maintainability.
Additionally, for loops are extensively used in data processing. When working with large datasets, it is often required to perform operations such as normalization, filtering, or transformation on each data point. By employing a for loop, one can iterate over the data, applying necessary functions dynamically. This capability is particularly useful in tasks such as image processing and statistical analysis, where each pixel in an image or each data point in a data set needs to be modified or analyzed individually.
Furthermore, automating repetitive analysis tasks through for loops enhances productivity. For example, when conducting batch processing in simulations or conducting Monte Carlo methods, a for loop can be utilized to simulate a system’s behavior under various parameters. This approach allows researchers to run numerous experiments systematically without repetitive manual intervention, thereby saving considerable time and effort.
Moreover, the versatility of for loops in MATLAB extends to generating plots and visualizations. By using a for statement, one can create multiple datasets and corresponding plots in a single script, streamlining the process of data visualization. Thus, for loops not only simplify coding but also enhance the research workflow by enabling efficient iterations through data manipulation and analysis tasks.
Advanced For Loop Techniques
In MATLAB programming, for loops serve as a fundamental construct for iterating over arrays and executing repetitive tasks. However, as one delves deeper into more complex problems, it is vital to adopt advanced techniques that can enhance the efficiency and performance of these loops. One powerful method of optimizing for loops in MATLAB is through vectorization.
Unlike traditional for loops that operate on a scalar level, vectorization enables bulk operations on entire arrays simultaneously. This not only reduces the number of iterations needed but also improves execution speed, making the code cleaner and more efficient.
For example, consider a scenario where you want to compute the square of each element in an array. A conventional approach using a for loop might look like this:
for i = 1:length(array)squared(i) = array(i)^2;end
In contrast, by utilizing vectorization, the same task can be accomplished with a single line as follows:
squared = array.^2;
This single statement is not only more concise, but it also significantly enhances performance by leveraging MATLAB’s underlying optimizations.
Another advanced technique involves the use of nested loops, which can be essential for multi-dimensional array manipulations. Although nested loops can sometimes reduce readability, when applied judiciously, they can handle complex data structures effectively. For example, if you are working with a matrix and need to apply an operation on each element based on its row and column indices, a nested for loop can be quite valuable.
However, it is crucial to consider performance implications. Excessive use of nested loops can lead to increased computational time, especially for large datasets. Therefore, it is advisable to evaluate whether such a structure is genuinely necessary or if it can be replaced by vectorized operations.
By employing these advanced techniques, developers can effectively manage the intricacies of for statement MATLAB, leading to not only improved performance but also enhanced code readability. Understanding when and how to use these methods is key to mastering for loops in MATLAB.
Watch MATLAB tutorials – For MATLAB Loop
In this in-depth tutorial, we explore the core concepts of loops like while and for in MATLAB from the mathworks, with practical examples for every beginner to expert level. Whether you’re looking to understand the structure of for MATLAB loops, while loops, or how to leverage the break and continue statements to control flow, this guide covers it all! What you’ll learn:
- For Loop Example: Learn how to use the for MATLAB loop to repeat a block of code a specific number of times.
- While Loop Example: Master the while loop for executing code as long as a condition is true.
- Break Statement: See how to exit loops early with the break statement when certain conditions are met.
- Continue Statement: Learn how to skip over parts of your loop with the continue statement.
- Nested Loops: Understand how to work with nested loops for more complex operations and data processing.
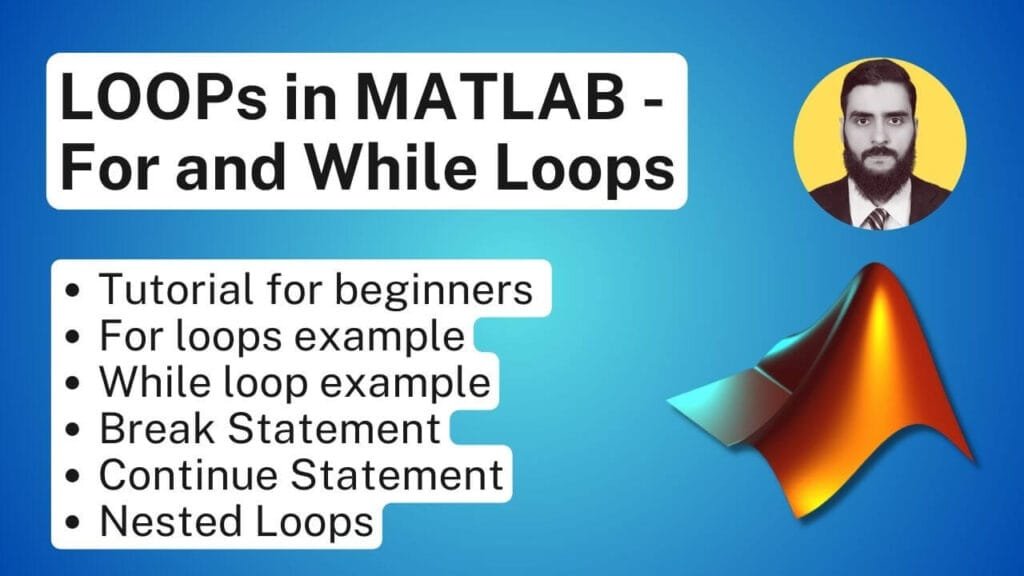
Debugging For Loops in MATLAB
Debugging is a fundamental skill that enhances code efficiency and accuracy, especially when working with loops in MATLAB. A for statement MATLAB is particularly useful for iterating through arrays or matrices, and errors can easily emerge during execution. Therefore, effectively debugging your loops is vital for any MATLAB programmer.
One of the primary strategies for debugging for loops in MATLAB is to utilize MATLAB’s built-in debugging tools. The MATLAB Editor includes a range of functionalities, such as highlighting syntax errors, which can be invaluable when you are trying to identify issues within your loops. This feature not only helps you pinpoint mistakes but also allows for a more organized approach to loop implementation.
Setting breakpoints within your code is another effective method to assist in debugging. With MATLAB, you can place breakpoints on specific lines of code where you suspect an error might be. When you run your script, MATLAB will pause execution at these points, enabling you to inspect variable values and the flow of execution. This is especially useful when a particular iteration of a for statement MATLAB causes abnormal behavior.
Additionally, implementing incremental testing is recommended when constructing your loop. This involves running your loop with a smaller dataset to observe its functionality before scaling up. This “test-driven” approach can uncover potential issues early on, allowing for quicker troubleshooting. It is also advisable to add display statements within your loop to provide real-time feedback on variable values and execution progress.
Lastly, practicing good coding habits, such as clear variable naming and avoiding overly complex logic, can significantly reduce the potential for errors in your loops. By following these strategies—using MATLAB’s debugging tools, setting breakpoints, conducting incremental testing, and maintaining code clarity—you can effectively debug your for loops in MATLAB and improve your overall coding efficiency.
Best Practices for Writing For Loops
When working with the for statement in MATLAB, it is essential to adhere to best practices that enhance code efficiency and maintainability. A well-structured loop not only improves performance but also aids in collaborative projects, making your code more understandable to peers. Here are some key strategies to consider.
First, focus on the clarity of your code structure. Ensure that loops are clearly defined and logically organized. Indentation is crucial as it visually separates the loop body from the rest of the code, allowing for easier tracking of flow and execution. When using the for statement MATLAB, maintain consistency in your variable naming conventions to reflect their purpose and the data they represent. This will significantly enhance readability for yourself and others.
Second, comment your code effectively. For every significant operation within the loop, include concise comments that describe what the section of code does. This is particularly important during the initial development phase and when revisiting your code after an absence. Well-commented code helps others—and your future self—understand the logic behind your approach, including the reasoning for specific loop iterations.
Additionally, consider performance when designing your loops. Avoid operations that could be vectorized or precomputed outside the loop. Such optimizations can lead to faster execution times and improved resource utilization. If a task can be accomplished without explicit loops, leverage MATLAB’s efficient array operations instead, as they are typically optimized for performance.
Lastly, when collaborating on projects, maintain open communication with your team. Discuss any loop-heavy sections of the code so that all contributors understand the design decisions made. Gather feedback to improve the efficiency and clarity of the loops being used, ensuring that they align with the project’s overall objectives.
Conclusion: Mastering For Loops in MATLAB
In summary, mastering the for statement in MATLAB is essential for anyone looking to enhance their programming skills within this robust computational environment. The for loop serves as a fundamental control structure, enabling users to execute a block of code multiple times while iterating through specified ranges or arrays. This feature is particularly valuable in various applications, from mathematical modeling to data analysis and visualization.
Throughout this guide, we have explored the syntax of the for statement in MATLAB, emphasizing its flexibility and the importance of clarity in code structure. Understanding key concepts such as loop counters, iteration limits, and nested loops will not only aid in crafting more efficient scripts but also improve the overall performance of your code. Furthermore, the proper usage of these control structures can lead to optimized algorithms, enhancing execution efficiency and contributing to cleaner, more maintainable code.
As you work on developing your proficiency with the for statement in MATLAB, it is crucial to engage in hands-on practice. Experimenting with varying constructs, such as leveraging arrays or creating complex nested loops, can help cement your understanding of the concepts discussed. Additionally, exploring supplementary resources, such as MATLAB’s official documentation, online tutorials, and coding forums, will further enrich your knowledge and skillset in loop optimization techniques.
Ultimately, the journey of mastering for loops in MATLAB is an ongoing process. Continually challenging yourself with more intricate problems will cultivate a deeper understanding and lead to more competent programming practices. Embrace this learning experience and regularly seek opportunities to apply the for statement in your projects, paving the way for expert-level proficiency in MATLAB programming.